In R programming, the modulo operator (%%) is a useful tool that often goes unnoticed yet holds the potential to enhance our data analysis and computational tasks significantly. In this comprehensive guide, we will dive deep into the world of modulo in R, exploring its functionality, applications, and practical usage.
%% Operator
In R, the %%
operator is the modulo operator. It calculates the remainder of the division between two numbers. For example, a %% b
gives the remainder when dividing a by b. If the remainder is 0, it means a is divisible by b.
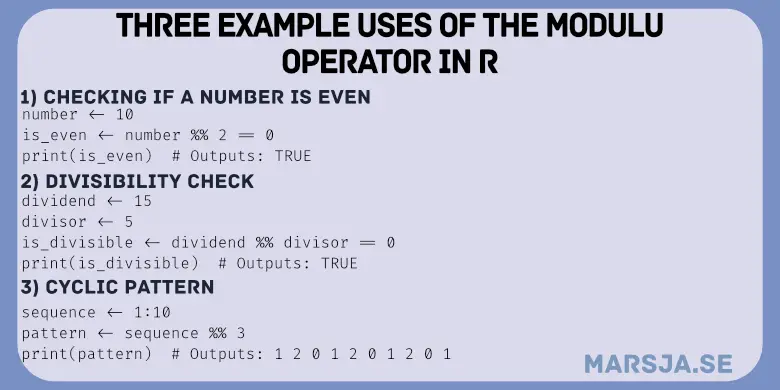
Table of Contents
- %% Operator
- Outline
- Prerequisites
- Synthetic Data
- Modulo in R: Practical Examples
- Conclusion
- Resources
Outline
The outline of the post is as follows: We begin with an overview of the modulo operator, getting into its fundamental concept and its significance within the context of programming. Subsequently, we explore a range of real-world applications of the modulo operator in the R programming language.
To ensure a smooth comprehension of the forthcoming examples, we outline the prerequisites for readers to engage effectively with the content. We then generate synthetic data, providing readers with a hands-on opportunity to practice utilizing the modulo operator through practical exercises.
The post strives after using practical examples to demonstrate the effective use of the modulo operator in R. In the first example, we will look at applying the modulo operator for data segmentation.
Moving forward, we focus on checking if numbers are odd or even using the modulo operator. This practical illustration highlights the simplicity and elegance of employing the modulo operator to categorize and process numerical data systematically.
In the subsequent example, we demonstrate the utility of the modulo operator in filtering specific days of the week from timestamp data. This scenario underlines the operator’s ability to efficiently extract cyclic patterns from time-based datasets.
Finally, we explore the optimization of iterative processes using the modulo operator. This example illuminates how the modulo operator can enhance code efficiency.
Throughout this post, the examples accompany straightforward code snippets and concise explanations, fostering a comprehensive understanding of leveraging the modulo operator in R for various practical applications.
Understanding Modulo
At its core, the modulo operator (%%) calculates the remainder of the division between two numbers. While this might sound simple, its implications are diverse. From data manipulation to control flow, we can use the modulo operator to simplify complex operations and achieve efficient results.
Applications of the Modulo in R
The versatility of R’s modulo operator makes it an indispensable tool for data analysts, programmers, and researchers alike. Here is a glimpse of what we will cover in this guide:
- Data Segmentation: Discover how modulo can assist in splitting datasets into smaller, manageable chunks. This is particularly useful when dealing with large-scale data analysis or when we want to create, e.g., control/experimental groups.
- Checking if a number is odd or even: Utilizing the modulo operator for determining odd or even numbers enhances your ability to categorize and process numerical data efficiently.
- Iterative Processes: Explore how modulo can streamline repetitive tasks, such as looping through arrays or lists. This enhances code efficiency and readability.
- Date and Time Manipulation: We can do time-related computations using modulo, making handling recurring events or time intervals easier.
Prerequisites
Before getting into the practical applications of the modulo operator in R, let us look at the prerequisites. Basic knowledge of R forms the foundation for understanding the concepts discussed in this post. If you are new to R, familiarizing yourself with its fundamental syntax and concepts will be beneficial.
Our first example will use the dplyr package, an essential data manipulation and analysis tool. To follow along, make sure you have dplyr installed. This package offers a plethora of functionalities, including column deletion, renaming, summarization, and more. Consider installing the tibble package, which extends R’s native dataframe. Tibble provides flexibility for data handling, including adding a column to R’s dataframe. Furthermore, if you plan to work with the synthetic data for the exercises, you must install tibble and dplyr.
As a best practice, I also recommended checking your R version and ensuring it is up-to-date. Updating R to the latest version ensures compatibility with packages and features, optimizing your programming experience.
Synthetic Data
Here is a synthetic dataset that can be used to practice working with the modulo operator in R:
# Create a dataset with a sequence of numbers and dates
data <- tibble(
id = seq(1, 1000),
value = seq(1, 1000, by = 1),
date = seq(as.Date("2020-11-30"), as.Date("2023-08-26"), by = "days")
)
# Add a category column for pattern detection
data <- data %>%
mutate(category = ifelse(id %in% c(20, 40, 60, 80), "Pattern", "Normal"))
# Display the first few rows of the dataset
head(data)
Code language: R (r)
We use the tibble and dplyr packages in the code chunk above to manipulate and organize data. By using these tools, we facilitate efficient data analysis and presentation. We initialize the random number generator’s seed with a set to ensure reproducibility (seed(123)
), guaranteeing consistent results for subsequent operations.
We create a data tibble containing three essential columns: id
, value
, and date
. The id column ranges from 1 to 100, and the value column increments in steps 1 from 1 to 1000. Here, we used the seq()
function to generate the sequence of numbers. Moreover, the date column encompasses dates between September 30, 2020, and August 26, 2023, with a daily interval.
To facilitate pattern detection, we introduce a category column. Using the mutate()
function from the dplyr package, we apply the ifelse()
function to classify certain rows as “Pattern” or “Normal” based on whether their id values match elements from sequence c(20, 40, 60, 80). Here we used %in% in R to achieve this. In the following sections, we will use this dataset to practice using modulo in R (%%).
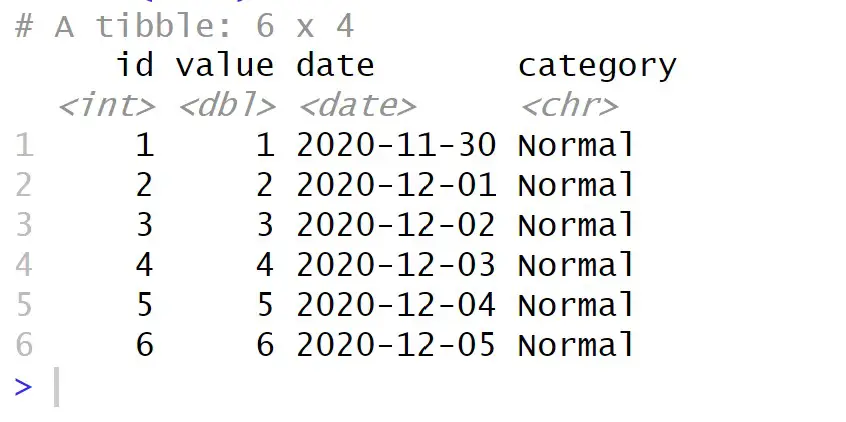
Modulo in R: Practical Examples
This section will explore some practical examples of the modulo operator in R.
Example 1: Modulo Operator in R for Data Segmentation
We can use the modulo operator in R to segment the dataset into smaller chunks. Here we do this based on modulo division of subject id:
# Load necessary libraries
library(dplyr)
# Segment the data based on modulo operation
segmented_data <- data %>%
mutate(segment = id %% 20)
# Randomly select a subset of persons from a specific segment (e.g., segment 5)
selected_subset <- segmented_data %>%
filter(segment == 5) %>%
sample_n(size = 10) # Randomly select 10 individuals from segment 5
# Display the selected subset
print(selected_subset)
Code language: R (r)
In the code chunk above, we use the modulo operator in R to perform data segmentation and create targeted subsets for specific applications. First, we loaded the dplyr library.
Assuming a pre-existing dataset named ‘data,’ we first employ the modulo operation (id %% 20) to segment the data into distinct groups, each represented by the ‘segment’ column. This segmentation aids in organizing the dataset, making it easier to work with subsets. We use the filter()
function to select the subjects in segment 5.
Next, we use the sample_n()
function, which enables us to select rows (in our case, individuals) randomly ls from the chosen segment. In this case, we select a subset of 10 individuals from segment 5. This process exemplifies how we can use modulo-based data segmentation to create targeted subgroups for various purposes. Here is the filtered data:
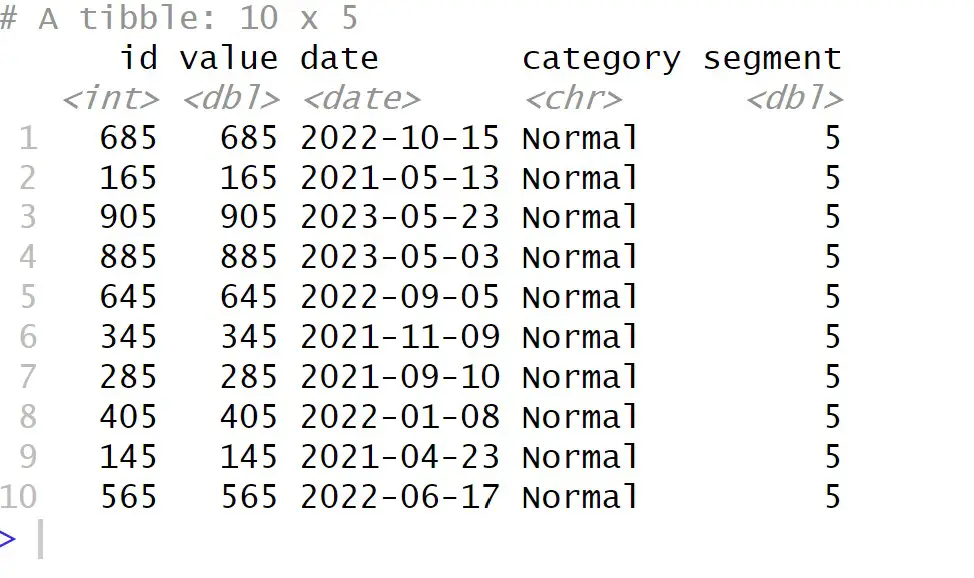
Example 2: Checking if Numbers are odd or Even with mod in R
Here, we use modulo in R to check if numbers are odd even:
# Check if a number is odd or even using the modulo operator
check_odd_even <- function(number) {
if (number %% 2 == 0) {
return("Even")
} else {
return("Odd")
}
}
# Example usage
input_number <- 17
result <- check_odd_even(input_number)
print(paste("The number", input_number, "is", result))
Code language: R (r)
In the code chunk above, we create a function named check_odd_even()
designed to determine the parity of a given number using the modulo operator. This function takes a single input, the number to be analyzed. Within the function, the modulo operator (%%
) evaluates if the input number can be divided by 2. If the remainder is 0, the function concludes that the number is even; otherwise, it identifies the number as odd.
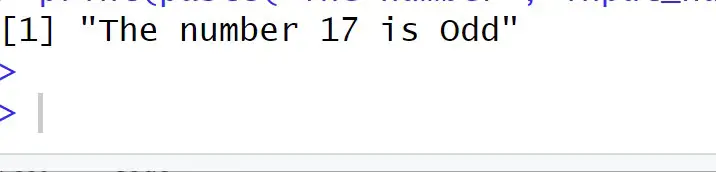
Example 3: Filtering Day of the Week using Modulo in R
Here is how we can utilize the modulo in R to filter data so that we get the rows where the date is a Friday:
# Convert timestamps to Date objects
dates <- as.Date(data$date)
# Categorize events by day of the week using modulo
day_of_week_index <- as.numeric(format(dates, "%u")) # 1: Monday, 2: Tuesday, ..., 7: Sunday
day_of_week <- weekdays(dates)
events_by_day <- data.frame(DayOfWeek = day_of_week, EventDate = dates,
DayIndex = day_of_week_index)
# Filter events for a specific day of the week using modulo
selected_day <- "Friday"
filtered_events <- events_by_day[events_by_day$DayIndex %% 5 == 0 &
events_by_day$DayOfWeek == selected_day, ]
Code language: R (r)
In the code chunk above, we first convert the ‘date’ column of the ‘data’ dataset into Date objects, allowing for date-based computations and analyses.
Next, we extract the day-of-week indices from the Date objects in R using as.numeric(format(dates, "%u"))
. This numeric representation ranges from 1 (Monday) to 7 (Sunday), enabling categorization.
We also retrieve the actual day names using weekdays(dates)
to enhance interpretability, resulting in the ‘day_of_week’ variable.
Furthermore, we create an ‘events_by_day’ dataframe, containing the day-of-week information, the corresponding Date objects, and the day indices.
In the final step, we apply the modulo operator in R to the day indices (DayIndex
) to filter events occurring on a specific day of the week. For instance, we focus on ‘Friday’ by utilizing the condition events_by_day$DayIndex %% 5 == 0 & events_by_day$DayOfWeek == selected_day
. Note how we use the $
operator to select columns. Here is the filtered data (or the first 6 rows):
We can, of course, use dplyr to filter the data instead:

Example 4: Optimizing Iterative Processes with Modulo in R:
We can enhance code efficiency by using the power of modulo in R for iterative processes:
# Generate a sequence of numbers
sequence <- 1:20
# Initialize an empty list to store results
results <- list()
# Apply modulo-based filtering
for (num in sequence) {
if (num %% 4 == 0) {
results[[num]] <- num^2
}
}
# Display the filtered results
cat("Results for numbers divisible by 4:", "\n")
for (num in sequence) {
if (!is.null(results[[num]])) {
cat("Number:", num, "Squared:", results[[num]], "\n")
}
}
Code language: R (r)
In the code chunk above, we first generate a sequence of numbers ranging from 1 to 20 using the 1:20
notation. This sequence represents the numbers we will process in our iterative optimization.
Next, we create an empty list named results, serving as a container for storing computed values. This efficient data structure ensures optimal storage of results without preallocating space.
The application of the modulo operator (num %% 4 == 0
) occurs within the for loop. Iterating through the sequence, each num is checked using the modulo operation to determine if it is divisible by 4. When this condition is satisfied, the square of the number (num^2
) is calculated and stored at the appropriate index within the results list.
To conclude, we display the filtered results using a second loop. Using cat()
facilitates the presentation of each number that meets the modulo-based criterion and its corresponding squared value. Here is the output:
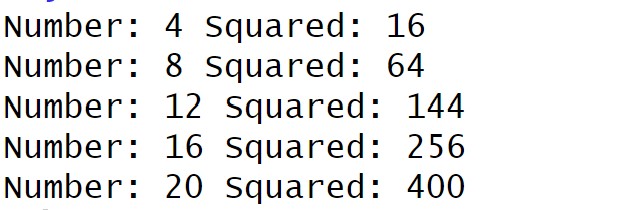
Conclusion
In this post, we have seen practical examples that hopefully show the versatility and power of the modulo operator in R. From data segmentation to categorizing numbers, filtering days of the week, and optimizing iterative processes, the modulo operator can be a valuable tool for streamlining code efficiency and enhancing data analysis.
By clearly understanding how to apply the modulo operator in real-world scenarios, I hope to have equipped you with a valuable addition to your programming toolkit.
Please share this post with your peers on social media to spread knowledge and engage in discussions about innovative applications of the modulo operator. Comment below if you have any suggestions, ideas, or requests for future topics or examples. Your feedback is invaluable in creating my content.
Resources
Here are some more resources you may find useful:
- How to Calculate Z Score in R
- Z Test in R: A Tutorial on One Sample & Two Sample Z Tests
- How to Create a Sankey Plot in R: 4 Methods
- R Count the Number of Occurrences in a Column using dplyr
- Correlation in R: Coefficients, Visualizations, & Matrix Analysis
- Countif function in R with Base and dplyr
- How to Standardize Data in R