In this tutorial, we will learn how to use Python to check if a file is empty without relying on external libraries. Python’s built-in OS module provides powerful tools for file manipulation and validation, making it an ideal choice for this task. Whether working with text files, CSVs, or other data formats, mastering file validation is crucial for ensuring data integrity and optimizing data processing workflows. Additionally, we will explore file validation for Zip and Rar files, broadening the scope of our data handling capabilities. Here, however, we need to rely on the library rarfile
for checking if a file in a Rar archive is empty with Python.
By validating files before processing, you can efficiently skip empty data files, potentially saving valuable time and resources. This ensures that only meaningful and relevant data is loaded and analyzed, enhancing the overall efficiency of your data processing tasks.
We will explore various methods to check for an empty file, including single files, all files in a folder, and recursively within nested folders. By understanding these different approaches, you can choose the one that best fits your use case.
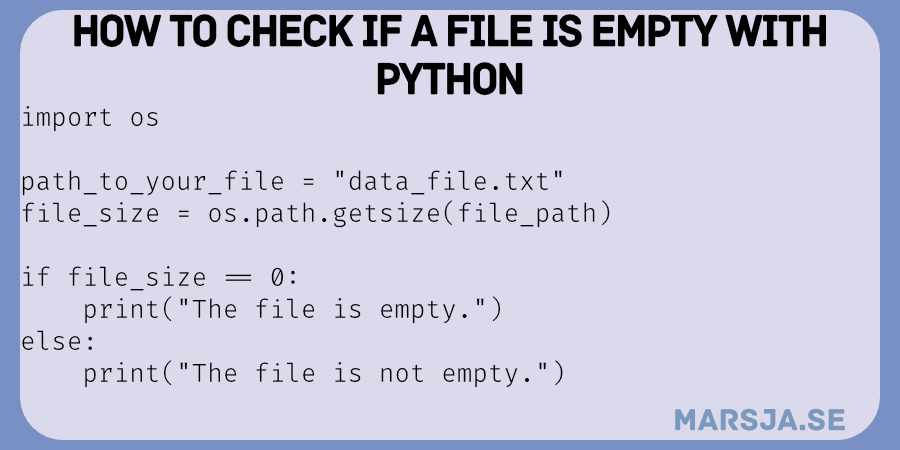
Python’s simplicity and versatility, combined with the functionality of the OS module, allow for efficient file validation, saving you time and reducing the risk of potential errors in your data analysis projects.
This tutorial will provide clear and concise code examples, empowering you to implement file validation easily. By the end of this post, you will be equipped with valuable techniques to confidently handle empty files and ensure the quality and reliability of your data.
Table of Contents
- Outline
- Prerequisites
- How to Use Python to Check if a File is Empty
- Illustrating the Process with Examples for Different File Formats:
- How to use Python to Check if Multiple Files in a Folder are Empty
- How to Check if Files of a Specific Type are Empty using Python
- How to Use Python to Check if Files are Empty Recursively
- How to use Python to Check if Files Contained in Zip & Rar files are Empty
- Conclusion: Check if a File is Empty with Python
- Resources
Outline
The outline of this Python tutorial is as follows. First, using the os
library, we will learn how to use Python to check if a file is empty. We will go through a step-by-step process, importing the os module, defining the file path, and using os.path.getsize()
to check the file size for emptiness.
Next, we will look at some practical examples of different file formats. We will illustrate using Python to check for empty text, CSV, and JSON files, providing code samples for each scenario.
Once we understand how to validate single files, we will progress to validating multiple files in a specific folder. This section will guide you in validating all files in a given directory using Python and explore code examples for handling various file formats.
Additionally, we will learn how to check for files of a specific type using Python and the glob
library. We will look at how to check if specific file types are empty in a folder. Consequently, narrowing down the validation process to focus on specific data formats.
For more extensive file validation tasks, we will look at using Python to check files recursively in nested folders. This section will provide code snippets to navigate nested directories and efficiently validate files.
Finally, we will explore how to check files within compressed Zip and Rar archives. This section will discuss methods for validating files within these archives. Here we will use the zipfile
and rarfile
libraries.
Prerequisites
To follow this tutorial, a basic understanding of Python programming is essential. Familiarity with Python’s syntax, data types, variables, and basic control structures (such as loops and conditional statements) will be beneficial.
Throughout this tutorial, we will primarily use Python’s built-in modules, which come pre-installed with Python. However, you must install the rarfile
library to validate files within Rar archives. You can easily install it using pip or conda by running the following command in your terminal or command prompt:
Using pip:
pip install rarfile
Code language: Bash (bash)
Using conda:
conda install -c conda-forge rarfile
Code language: Bash (bash)
Additionally, it is essential to ensure that pip is up to date. You can upgrade pip by running the following command:
pip install --upgrade pip
Code language: Bash (bash)
By having these prerequisites in place, you will be well-equipped to follow along with the examples and effectively validate files in Python, regardless of their format or nesting level. Let us explore how to use Python to check if a file is empty and optimize your data processing workflows.
How to Use Python to Check if a File is Empty
Here are a few steps to use Python to check if a file is empty:
Step 1: Import the os module
First, we must import the os module, which provides various methods for interacting with the operating system, including file operations.
import os
Code language: Python (python)
Note that we can use os
when reading files in Python as well.
Step 2: Define the File Path
Next, specify the file path. Replace ‘file_path’ with the path to the file you want to check:
# Replace with the actual file path
file_path = 'file_path'
Code language: Python (python)
Step 3: Use os.path.getsize() to Check File Size
The os.path.getsize()
function returns the file size in bytes. We can determine if the file is empty by comparing the size with zero:
# Get the file size of the file
file_size = os.path.getsize(file_path)
# Check if the file is empty
if file_size == 0:
print("The file is empty.")
else:
print("The file is not empty.")
Code language: Python (python)
In the code chunk above, we first get the file size using the os.path.getsize()
function. This step allows us to determine the file’s content.
Next, we use an if-else statement to check if the file size equals zero. If the file size is zero, it means the file is empty. We print the message “The file is empty.” Otherwise, if the file size is not zero, we print the message “The file is not empty.”
Following these simple steps and using the os module in Python, we can efficiently perform file validation and quickly show if a file is empty. In the following sections, we will check if different file formats are empty.
Illustrating the Process with Examples for Different File Formats:
Here are three examples on checking if a file is empty with Python. All files can be downloaded here.
Example 1: How to use Python for Checking Empty Text Files
Here is how to use Python to check whether a text file is empty:
import os
file_path = 'data6.txt'
file_size = os.path.getsize(file_path)
if file_size == 0:
print("The text file is empty.")
else:
print("The text file is not empty.")
Code language: Python (python)
In the code chunk above, we checked if the data6.txt file was empty. We can see from the output that it is empty:
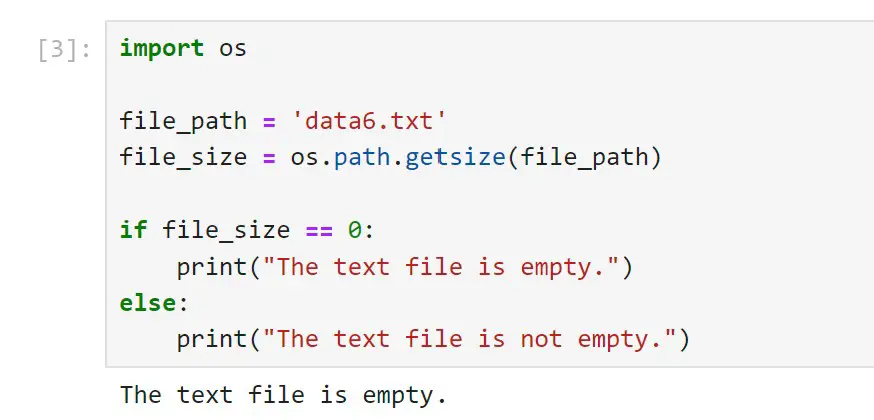
Example 2: How to Use Python for Checking Empty CSV Files
Now, here is how to use Python to check if a CSV file is empty:
import os
file_path = 'data5.csv'
file_size = os.path.getsize(file_path)
if file_size == 0:
print("The CSV file is empty.")
else:
print("The CSV file is not empty.")
Code language: PHP (php)
Here we can see the results from checking the CSV file:
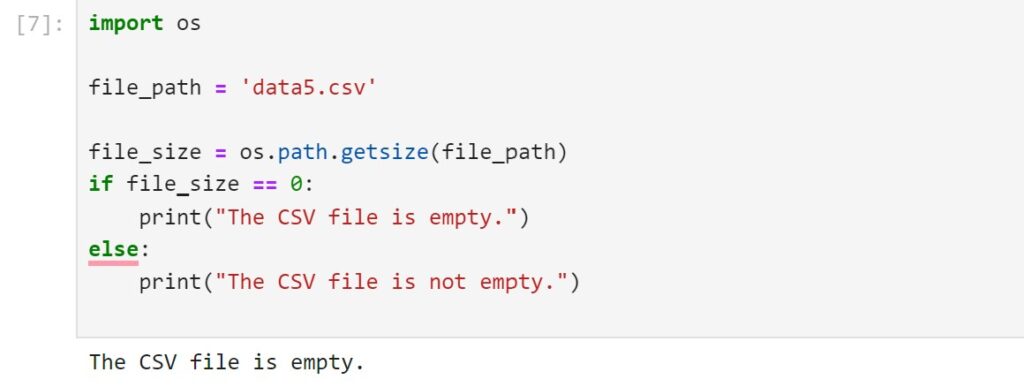
Example 3: How to Use Python for Checking Empty JSON Files
We can also use Python to check if a JSON file is empty:
import os
file_path = 'data1.json'
file_size = os.path.getsize(file_path)
if file_size == 0:
print("The CSV file is empty.")
else:
print("The CSV file is not empty.")
Code language: PHP (php)
Following these step-by-step instructions and using the code examples for different data file formats, you can quickly check if a single file is empty in Python using the OS module. Here we see that the “data1.json” file was not empty:
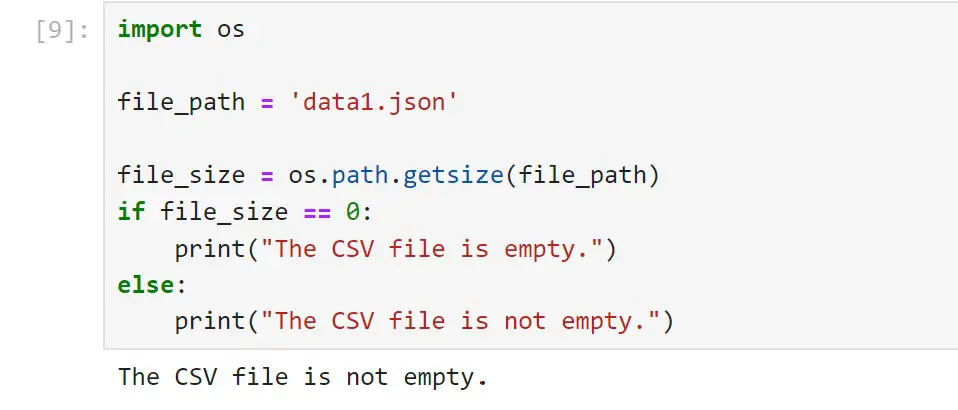
How to use Python to Check if Multiple Files in a Folder are Empty
Here is an example of how we can use Python to check which files in a folder are empty:
import os
# Specify the directory path
folder_path = "/path/to/your/folder"
# Get the list of all files in the folder
files = os.listdir(folder_path)
# Loop through each file and check if it's empty
for file in files:
file_path = os.path.join(folder_path, file)
file_size = os.path.getsize(file_path)
if file_size == 0:
print(f"The file {file} is empty.")
else:
print(f"The file {file} is not empty.")
Code language: Python (python)
In the code block above, we first specify the folder_path
variable to point to the folder containing the files we want to validate. The os.listdir()
function retrieves a list of all files in the specified folder, which we store in the files variable.
Next, we loop through each file in the list and use the same file validation process. For each file, we check if the file size is zero to determine if the file is empty or not. We print the corresponding message indicating whether the file is empty depending on the result. We can also store the non-empty files in a Python list:
import os
# Specify the directory path
folder_path = "/path/to/your/folder"
# Get the list of all files in the folder
files = os.listdir(folder_path)
# Create an empty list to store non-empty files
non_empty_files = []
# Loop through each file and check if it's empty
for file in files:
file_path = os.path.join(folder_path, file)
file_size = os.path.getsize(file_path)
if file_size == 0:
print(f"The file {file} is empty.")
else:
print(f"The file {file} is not empty.")
non_empty_files.append(file)
# Display the list of non-empty files
print("Non-empty files:", non_empty_files)
Code language: Python (python)
In the code chunk above, we added the list (non_empty_files
). Moreover, we add each non-empty file to this Python list. See the highlighted lines in the code chunk above. We can use this list to, for example, read all the CSV files that are empty. Importantly, change the folder_path
variable to the path to your data. Here is the result when running the above code on a folder containing some of the example data files:
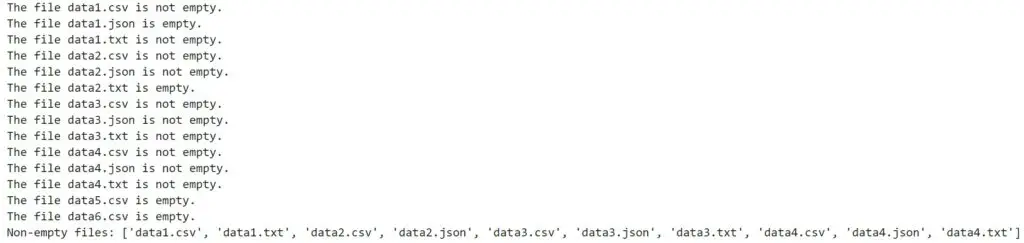
How to Check if Files of a Specific Type are Empty using Python
We can use the glob module to filter files based on a specific file type using wildcards. The glob.glob()
function allows you to search for files in a folder using wildcards. Here is how we can modify the code to read only text files:
import os
import glob
# Specify the directory path with wildcard for file type
folder_path = "/path/to/your/folder/*.txt"
# Get the list of all files matching the wildcard in the folder
files = glob.glob(folder_path)
# Create an empty list to store non-empty files
non_empty_files = []
# Loop through each file and check if it's empty
for file in files:
file_size = os.path.getsize(file)
if file_size == 0:
print(f"The file {os.path.basename(file)} is empty.")
else:
print(f"The file {os.path.basename(file)} is not empty.")
non_empty_files.append(os.path.basename(file))
# Display the list of non-empty files
print("Non-empty files:", non_empty_files)
Code language: PHP (php)
In the code chunk above, we use the glob.glob()
function to get the list of files matching the *.txt wildcard. Consequently, we will only process files with the .txt extension. The rest of the code remains the same as in the previous example.
How to Use Python to Check if Files are Empty Recursively
To use Python to check if files are empty recursively for nested folders, we can use the os.walk()
function. Here is a code example to perform file validation recursively:
import os
# Specify the top-level directory path
top_folder_path = "/path/to/your/top_folder"
# Function to validate files in a folder
def validate_files_in_folder(folder_path):
# Get the list of all files in the folder
files = os.listdir(folder_path)
# Create an empty list to store non-empty files in the current folder
non_empty_files = []
# Loop through each file and check if it's empty
for file in files:
file_path = os.path.join(folder_path, file)
file_size = os.path.getsize(file_path)
if file_size == 0:
print(f"The file {file} in folder {folder_path} is empty.")
else:
print(f"The file {file} in folder {folder_path} is not empty.")
non_empty_files.append(file)
return non_empty_files
# Function to recursively validate files in nested folders
def recursively_validate_files(top_folder_path):
non_empty_files_in_nested_folders = []
for root, _, _ in os.walk(top_folder_path):
non_empty_files = validate_files_in_folder(root)
non_empty_files_in_nested_folders.extend([(root, file) for file in non_empty_files])
return non_empty_files_in_nested_folders
# Perform recursive file validation for nested folders
result = recursively_validate_files(top_folder_path)
# Display the list of non-empty files in nested folders
print("Non-empty files in nested folders:")
for root, file in result:
print(f"{os.path.join(root, file)}")
Code language: Python (python)
In the code block above, we create two functions: validate_files_in_folder()
and recursively_validate_files()
. We can use the validate_files_in_folder()
function to check if files are empty in a specific folder, similar to the previous example. However, the recursively_validate_files()
function uses os.walk()
to navigate through all nested folders under the top_folder_path
. Moreover, it calls validate_files_in_folder()
for each folder. The function then collects the non-empty files from all the nested folders and returns a list of tuples containing the folder path and file name for each non-empty file. By using os.walk()
, we can effectively check if files are empty in all nested folders and subdirectories. Here is the result from running the above code:
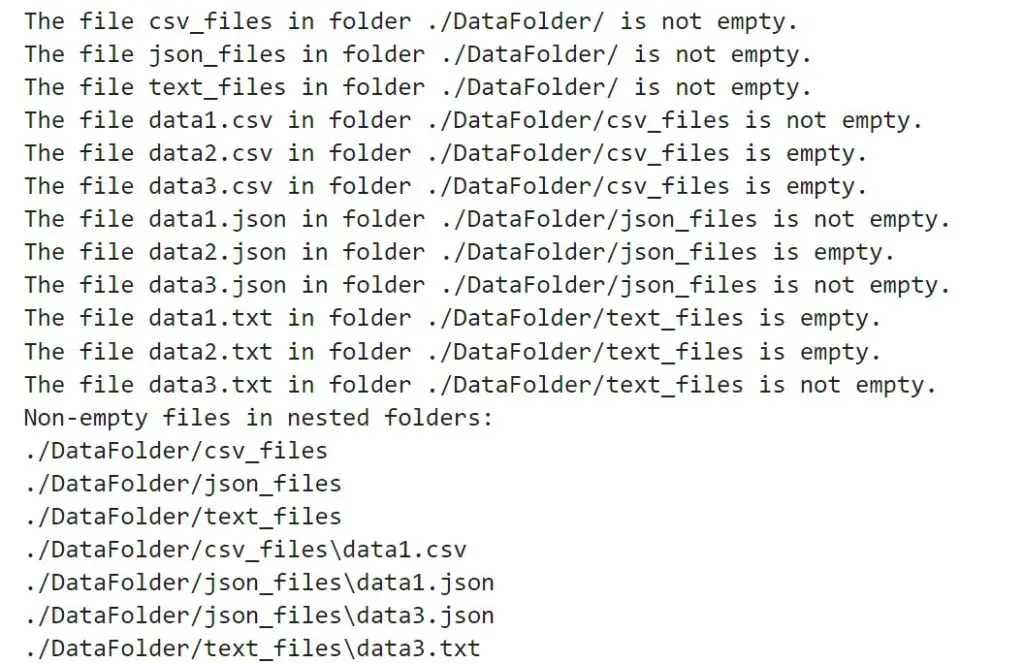
As can be seen from the image above, the script will also check if a directory is empty or not with Python.
How to use Python to Check if Files Contained in Zip & Rar files are Empty
When working with compressed Zip and Rar archives, we can use Python libraries like zipfile
and rarfile
to check whether the files contained within these are empty. These libraries allow us to extract and access the files without actually decompressing the entire archive, which is a significant benefit when dealing with large compressed data sets.
Validating Zip Files
Here is a Python code example that you can use to check whether the files within a Zip file are empty:
import os
import rarfile
# Specify the path to the compressed Zip archive
zip_file_path = "/path/to/your/file.zip"
# Function to validate files within a Zip archive
def validate_files_in_zip(zip_file_path):
with zipfile.ZipFile(zip_file_path, "r") as zip_file:
non_empty_files = []
for file_info in zip_file.infolist():
# Get the file size of each file in the archive
file_size = file_info.file_size
# Check if the file is empty
if file_size == 0:
print(f"The file {file_info.filename} in the Zip archive is empty.")
else:
print(f"The file {file_info.filename} in the Zip archive is not empty.")
non_empty_files.append(file_info.filename)
return non_empty_files
# Perform file validation for Zip archive
non_empty_files_in_zip = validate_files_in_zip(zip_file_path)
# Display the list of non-empty files in the Zip archive
print("Non-empty files in the Zip archive:")
for file in non_empty_files_in_zip:
print(file)
Code language: PHP (php)
In the code chunk above, we validate files within a Zip archive using Python’s zipfile
library. The key difference compared to the previous examples is that we are now dealing with a compressed Zip archive
We start by importing the required modules, os
and zipfile
. Next, we define a function called validate_files_in_zip
, which takes the path to the compressed Zip archive as input. We use the with statement inside the function to open the Zip archive specified by zip_file_path
. The “r” mode opens the archive in read mode.
We then iterate through each file in the Zip archive using a for loop and the infolist()
method of the zip_file
object. For each file, we retrieve its file size using the file_size
attribute of the file_info
object.
Next, we use a Python if statement to check if the file is empty, much like in the previous examples.
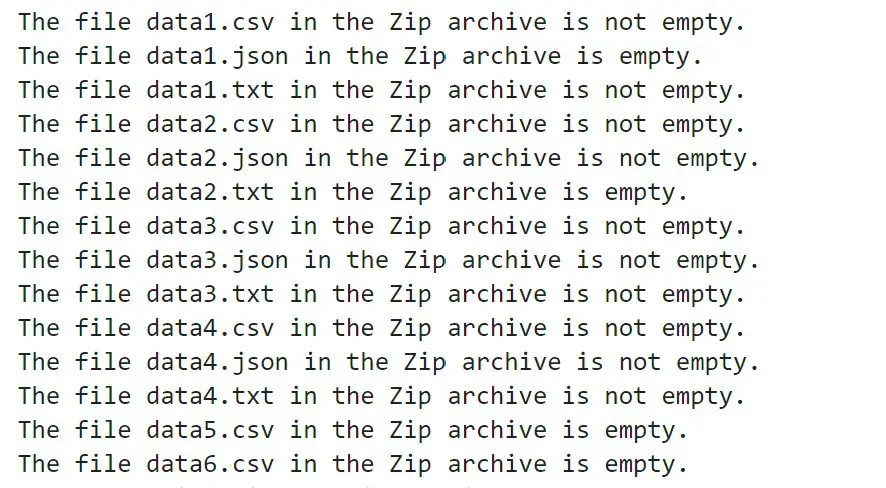
Finally, after validating all files in the Zip archive, we return the list of non-empty file names. The function validate_files_in_zip()
is then called with the specified zip_file_path
, and the list of non-empty files is stored in the variable non_empty_files_in_zip
.
Validating Rar Files
Here is a code example that you can use to check whether the files within a Rar file are empty:
import os
import rarfile
# Specify the path to the compressed Rar archive
rar_file_path = "/path/to/your/file.rar"
# Function to validate files within a Rar archive
def validate_files_in_rar(rar_file_path):
with rarfile.RarFile(rar_file_path, "r") as rar_file:
non_empty_files = []
for file_info in rar_file.infolist():
# Get the file size of each file in the archive
file_size = file_info.file_size
# Check if the file is empty
if file_size == 0:
print(f"The file {file_info.filename} in the Rar archive is empty.")
else:
print(f"The file {file_info.filename} in the Rar archive is not empty.")
non_empty_files.append(file_info.filename)
return non_empty_files
# Perform file validation for Rar archive
non_empty_files_in_rar = validate_files_in_rar(rar_file_path)
# Display the list of non-empty files in the Rar archive
print("Non-empty files in the Rar archive:")
for file in non_empty_files_in_rar:
print(file)
Code language: Python (python)
Note that the only difference is the name of the function and that we use the rarfile
library.
Conclusion: Check if a File is Empty with Python
In conclusion, mastering file validation in Python is a valuable skill for any data analyst or scientist. By learning Python to check if a file is empty, you can ensure data integrity and optimize your data processing workflows. Whether you are working with text files, CSVs, or other data formats, quickly identifying and handling empty files is crucial for accurate data analysis.
Moreover, checking if files are empty becomes even more beneficial when dealing with large datasets or many data files. You can save time and resources by efficiently validating files, avoiding unnecessary data processing and analysis on empty files.
We have explored various methods to validate files, including single files, multiple files in a folder, and files within compressed archives like Zip and Rar files. Through step-by-step explanations and practical code examples, you now understand how to use Python’s capabilities for effective file validation.
If you found this tutorial helpful, consider sharing it on your social media platforms to help others looking to enhance their data validation skills using Python. Additionally, I welcome your comments and suggestions below. If you have any requests for new posts or need assistance with any data-related challenges, feel free to share them with me. I strive to provide valuable Python tutorials and resources.
Resources
Here are some other good tutorials may elevate your learning:
- Coefficient of Variation in Python with Pandas & NumPy
- Your Guide to Reading Excel (xlsx) Files in Python
- How to Make a Violin plot in Python using Matplotlib and Seaborn
- Find the Highest Value in Dictionary in Python
- How to get Absolute Value in Python with abs() and Pandas
- Levene’s & Bartlett’s Test of Equality (Homogeneity) of Variance in Python