Finding the highest value in a dictionary is a common task in Python programming. Whether you are working with a dictionary containing numerical data or other values, knowing how to extract the maximum value can be invaluable. In this tutorial, we will explore various techniques to accomplish this task and provide a comprehensive understanding of how to find the maximum value in a dictionary using Python.
There are numerous scenarios where finding the highest value in a dictionary becomes essential. For instance, you should identify the top-selling product when analyzing sales data. You should determine the highest-scoring student in a dictionary of student grades. Finding the maximum value is crucial for data analysis and decision-making regardless of the use case.
Throughout this Python tutorial, we will demonstrate multiple approaches to tackling this problem. From utilizing built-in functions like max()
and sorted()
to employ list comprehension and lambda functions, we will cover a range of techniques suitable for different scenarios. Additionally, we will discuss potential challenges and considerations when working with dictionaries in Python.
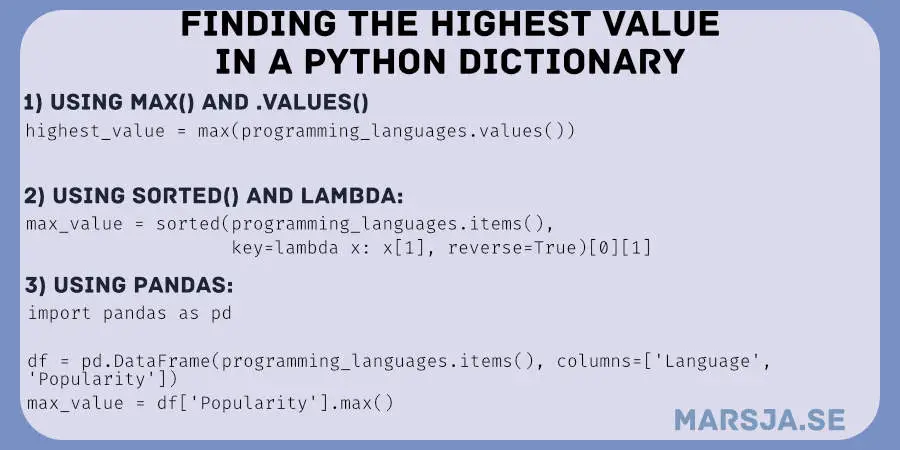
By the end of this tutorial, you will have a solid grasp of various methods to find the highest value in a dictionary using Python. Whether you are a beginner or an experienced Python programmer, the knowledge gained from this tutorial will equip you with the tools to handle dictionary operations and quickly extract the maximum value efficiently. So let us dive in and learn how to find the maximum value in a dictionary in Python!
Table of Contents
- Outline
- Prerequisites
- Python Dictionary
- How to Find the Highest Value in a Dictionary in Python
- How to Find the Key of the Max Value in a Dictionary in Python
- Finding the Highest Value in a Dictionary in Python with sorted()
- Find the Highest Value in a Dictionary in Python using Collections
- Finding the Highest Value in a Python Dictionary using Pandas
- Which Method is the Quickest Getting the Highest Value?
- Conclusion
- Resources
Outline
The outline of this post will guide you through finding the highest value in a dictionary in Python. Before diving into the specifics, having a basic understanding of Python and familiarity with dictionaries is essential.
We will begin by exploring the Python dictionary data structure, which stores key-value pairs. A solid understanding of dictionaries is crucial for effectively retrieving the highest value.
Next, we will learn different methods for finding the highest value. Our discussion will cover various approaches, including using built-in functions, sorting values, utilizing the collections module, and leveraging the power of the Pandas library.
First, we will focus on the techniques for finding the highest value. This will involve accessing values directly, sorting the dictionary values, and employing the collections module.
Subsequently, we will explore methods for finding the key associated with the highest value. This will enable us to retrieve the highest value and its corresponding key.
Throughout the post, we will compare the advantages and drawbacks of each method, taking into consideration factors such as performance and ease of implementation. Additionally, we will address scenarios involving multiple highest values and discuss appropriate handling strategies.
We will accompany our explanations with code examples and detailed explanations to provide practical insights. Furthermore, we will measure and compare the execution times of different methods to determine the most efficient approach.
By the end of this post, you will have a comprehensive understanding of various methods for finding the highest value in a dictionary. With this knowledge, you can confidently choose the most suitable approach based on your requirements.
Prerequisites
Before exploring the highest value in a dictionary using Python, let us go through a few prerequisites to ensure a solid foundation.
First, it is essential to have Python installed on your system. Python is a widely-used programming language that provides a powerful and versatile data manipulation and analysis environment.
Additionally, a basic understanding of Python programming is recommended. Familiarity with concepts such as variables, data types, loops, and dictionaries will help you follow along with the examples and code provided in this tutorial.
To set the context, let us briefly review the concept of dictionaries in Python. In Python, a dictionary is an unordered collection of key-value pairs, where each key is unique. It provides efficient lookup and retrieval of values based on their associated keys.
Furthermore, this tutorial will also cover converting a dictionary of lists into a Pandas dataframe. This knowledge will enable us to work with the data more effectively and perform various operations to find the highest value in the dictionary.
With these prerequisites and a solid understanding of dictionaries, we are well-prepared to explore finding the highest value in a dictionary using Python!
Python Dictionary
Dictionaries in Python are versatile data structures that allow us to store and retrieve values using unique keys. Each key is associated with a value in a dictionary, similar to a real-life dictionary where words are paired with their definitions. This data structure is particularly useful when quickly accessing values based on specific identifiers.
Let us create a dictionary to represent the popularity of different programming languages. We will use the programming languages as keys and their corresponding popularity values as the associated values.
# Create a dictionary of programming languages and their popularity
programming_languages = {
"Python": 85,
"Java": 70,
"JavaScript": 65,
"C++": 55,
"C#": 45,
"Ruby": 35,
"Go": 30
}
Code language: Python (python)
In the code chunk above, we define a dictionary called programming_languages. The keys represent different programming languages, such as “Python”, “Java”, “JavaScript”, and so on, while the values represent their respective popularity scores. Each language is paired with a numeric value indicating its popularity level.
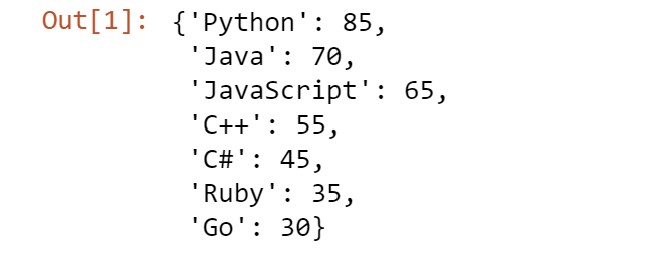
Now that we have our dictionary set up, we can find the highest value in the dictionary to determine the most popular programming language.
How to Find the Highest Value in a Dictionary in Python
We can utilize various techniques to find the highest value in a dictionary in Python. One straightforward approach uses the max()
function and a custom key to determine the maximum value. We can easily identify the highest value by passing the dictionary’s values to the max()
function. Additionally, we can use the items()
method to access the keys and values of the dictionary simultaneously.
Here is an example of how to find the highest value in a dictionary using the max()
function:
# Find the highest value in the dictionary
highest_value = max(programming_languages.values())
print("The highest value in the dictionary is:", highest_value)
Code language: Python (python)
In the code chunk above, we apply the max() function to the values() of the programming_languages dictionary. The result is stored in the highest_value variable, representing the highest popularity score among the programming languages. Finally, we print the highest value to the console.
After finding the highest value, we can retrieve the corresponding key(s) or perform further analysis based on this information. Understanding how to find the highest value in a dictionary allows us to extract valuable insights from our data efficiently.
How to Find the Key of the Max Value in a Dictionary in Python
If we, on the other hand, use max(programming_languages)
without explicitly specifying the values()
method, Python will consider the dictionary’s keys for comparison instead of the values. The result is the key with the highest lexical order (based on the default comparison behavior for strings).
Let us see an example:
# Find the maximum key (based on lexical order) in the dictionary
max_key = max(programming_languages)
print("The key with the highest lexical order is:", max_key)
Code language: Python (python)
In the code chunk above, max(programming_languages)
returns the key ‘Python’ because it is the last key in the alphabetical order among the programming languages. This behavior occurs because, by default, Python compares dictionary keys when no specific key or value is provided.
It is important to note that using max()
without specifying values()
may not give you the desired result when you want to find the highest value in a dictionary. To accurately identify the highest value, it is crucial to explicitly apply the max()
function to the dictionary’s values, as demonstrated in the previous example.
Finding the Highest Value in a Dictionary in Python with sorted()
Another method to find the highest value in a dictionary is using the sorted()
function and a lambda function as the key parameter. This approach allows us to sort the dictionary items based on their values in descending order and retrieve the first item, which will correspond to the highest value.
Here is an example:
# Find the maximum value in the dictionary
max_value = sorted(programming_languages.items(),
key=lambda x: x[1], reverse=True)[0][1]
print("The highest value in the dictionary is:", max_value)
Code language: Python (python)
We can modify our approach when multiple values can be the highest in a dictionary. Here we compare each value to the maximum value and add the corresponding keys to a list. Consequently, we retrieve all the key-value pairs with the highest value.
Here is an example:
# Find all keys with the highest value in the dictionary
max_value = max(programming_languages.values())
highest_keys = [key for key, value in programming_languages.items() if value == max_value]
print("The highest value(s) in the dictionary is/are:", highest_keys)
Code language: PHP (php)
In the code chunk above, max_value = max(programming_languages.values())
finds the maximum value in the dictionary. Then, the list comprehension [key for key, value in programming_languages.items() if value == max_value]
iterates over the dictionary items and selects the highest-value keys.
This approach allows us to obtain all the keys corresponding to the highest value in the dictionary, even if multiple keys have the same highest value.
Find the Highest Value in a Dictionary in Python using Collections
A third method we can use to get the maximum value from a Python dictionary is utilizing the collections module. This module provides the Counter class, which can be used to count the occurrences of values in the dictionary. We can retrieve the value with the highest count by using the most_common()
method and accessing the first item.
Here is an example:
import collections
max_value = collections.Counter(programming_languages).most_common(1)[0][1]
print("The highest value in the dictionary is:", max_value)
Code language: JavaScript (javascript)
In the code chunk above, we import the collections module and use the Counter class to count the occurrences of values in the programming_languages dictionary. By calling most_common(1)
, we retrieve the item with the highest count, and [0][1]
allows us to access the count value specifically. Finally, we print the highest value from the dictionary.
Using the collections module provides an alternative method for obtaining the maximum value from a dictionary, particularly when counting the occurrences of values relevant to the analysis or application at hand.
Finding the Highest Value in a Python Dictionary using Pandas
We can also use the Pandas Python package to get the highest value from a dictionary if we want to. Pandas provides a powerful DataFrame structure that allows us to organize and analyze data efficiently. By converting the dictionary into a DataFrame, we can make use of Pandas’ built-in data manipulation and analysis functions.
Here is an example:
import pandas as pd
df = pd.DataFrame(programming_languages.items(), columns=['Language', 'Popularity'])
max_value = df['Popularity'].max()
print("The highest value in the dictionary is:", max_value)
Code language: JavaScript (javascript)
In the code chunk above, we import the Pandas library and create a DataFrame df using the pd.DataFrame()
function. We pass the programming_languages.items()
to the function to convert the Python dictionary items into rows of the DataFrame. Using the columns parameter, we specify the column names as ‘Language’ and ‘Popularity’.
We use the max()
function on the ‘Popularity’ column of the DataFrame, df[‘Popularity’], to find the highest value. This function returns the maximum value in the column. Finally, we print the highest value using the print()
function.
Using Pandas offers an alternative approach for retrieving the highest value from a dictionary. Using Pandas is especially beneficial when the data is structured as a DataFrame or when additional data analysis operations need to be performed. Here are some more Pandas tutorials:
- Pandas Convert Column to datetime – object/string, integer, CSV & Excel
- How to Convert a NumPy Array to Pandas Dataframe: 3 Examples
- Adding New Columns to a Dataframe in Pandas (with Examples)
- How to Add Empty Columns to Dataframe with Pandas
Which Method is the Quickest Getting the Highest Value?
The method’s efficiency becomes crucial when seeking the highest value in a Python dictionary. Finding the quickest approach is essential, especially when dealing with large dictionaries or when performance is a significant factor. Measuring the execution time of various methods allows us to determine which performs best.
In the provided code snippet, we have four distinct methods for finding the highest value in a dictionary. The first method employs the built-in max()
function directly on the dictionary’s values. The second method converts the dictionary values into a list and then applies the max()
function. The third method involves using the Counter
class from the collections
module to identify the most common element. Lastly, the fourth method utilizes Pandas to convert the dictionary to a DataFrame and employs the max()
function on a specific column.
To measure the execution time of each method accurately, we use the time
module. By recording the start and end times for each method’s execution, we can calculate the elapsed time and compare the results.
Here is the code snippet for timing the different methods:
import time
import collections
import pandas as pd
# Generate a large dictionary
large_dict = {i: i * 2 for i in range(10000000)}
# Method 1:
start_time_method1 = time.time()
max_value_method1 = max(large_dict.values())
end_time_method1 = time.time()
execution_time_method1 = end_time_method1 - start_time_method1
# Method 2:
start_time_method2 = time.time()
max_value_method2 = sorted(large_dict.values())[-1]
end_time_method2 = time.time()
execution_time_method2 = end_time_method2 - start_time_method2
# Method 3:
start_time_method3 = time.time()
max_value_method3 = collections.Counter(large_dict).most_common(1)[0][1]
end_time_method3 = time.time()
execution_time_method3 = end_time_method3 - start_time_method3
# Method 4:
start_time_method4 = time.time()
df = pd.DataFrame(large_dict.items(), columns=['Key', 'Value'])
max_value_method4 = df['Value'].max()
end_time_method4 = time.time()
execution_time_method4 = end_time_method4 - start_time_method4
# Print the execution times for each method
print("Execution time for Method 1:", execution_time_method1)
print("Execution time for Method 2:", execution_time_method2)
print("Execution time for Method 3:", execution_time_method3)
print("Execution time for Method 4:", execution_time_method4)
Code language: PHP (php)
To compare the performance of different methods in finding the highest value in a large dictionary, we created large_dict with 10 million key-value pairs. Using the time module, we measured the execution time of each method to evaluate its efficiency.

Method 1 directly utilized the max()
function on the dictionary values. This method seemed to have the shortest execution time of approximately 0.295 seconds. Method 2 involved sorting the values and retrieving the last element. This method was close, with an execution time of around 0.315 seconds.
The execution times obtained from these tests provide insights into the efficiency of each method. They can help determine the most effective approach for finding the highest value in a dictionary. By considering the execution times, we can select the method that best suits our requirements regarding speed and performance.
On the other hand, Method 3 utilized the collections. Counter class to find the most common element in the dictionary, resulting in an execution time of approximately 1.037 seconds. Finally, Method 4 involved converting the dictionary to a Pandas DataFrame and using the max()
function on a specific column. This method exhibited the longest execution time, taking around 7.592 seconds.
Based on the results, Methods 1 and 2 directly access the dictionary values are the most efficient approaches for finding the highest value in a large dictionary. These methods require minimal additional processing, resulting in faster execution times. Method 3, although slightly slower, offers an alternative approach using the collections module. However, Method 4, which employs Pandas and DataFrame conversion, is considerably slower due to the additional overhead of DataFrame operations.
When choosing the best method for finding the highest value in a dictionary, it is crucial to consider both speed and simplicity. Methods 1 and 2 balance efficiency and straightforward implementation, making them ideal choices in most scenarios.
By understanding the performance characteristics of different methods, we can make informed decisions when handling large dictionaries in Python, ensuring optimal performance for our applications.
However, it is important to consider your use case’s trade-offs and specific requirements. Factors such as the size of the dictionary, the frequency of operations, and the need for additional functionality influence the optimal choice of method.
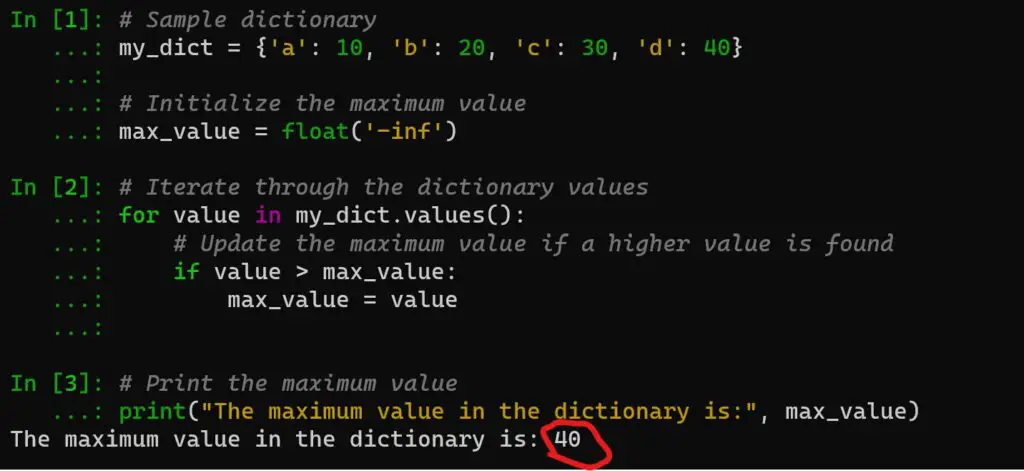
Conclusion
In this post, you have learned various methods to find the highest value in a dictionary in Python. We started by understanding the Python dictionary data structure and key-value pairs, forming the foundation for efficiently retrieving the max value.
We explored multiple approaches, including direct value access, sorting, using the collections module, and take advantage of the power of the Pandas library. Each method offers advantages and considerations, allowing you to choose the most suitable approach based on your specific requirements.
To evaluate their performance, we conducted timing tests on large dictionaries. The results showed that methods utilizing built-in functions and direct value access, such as max(), tended to be the quickest for finding the max value. However, the performance may vary depending on the dictionary’s size and structure.
By familiarizing yourself with these methods, you have gained the knowledge and tools to find the max value in a dictionary in Python effectively. Whether you need to retrieve the highest value itself or its associated key, you now have a range of techniques at your disposal.
Remember, the most efficient method depends on the context and characteristics of your dictionary. When choosing the appropriate approach, it is essential to consider factors like performance, data structure, and any additional requirements.
In conclusion, finding the max value in a dictionary in Python is a fundamental task, and with the insights gained from this post, you are well-equipped to tackle it confidently. To further enhance your learning experience, you can explore the accompanying Notebook, containing all the example codes in this post. You can access the Jupyter Notebook here.
If you found this post helpful and informative, please share it with your fellow Python enthusiasts on social media. Spread the knowledge and empower others to enhance their Python skills as well. Together, we can foster a vibrant and supportive community of Python developers.
Thank you for joining me on this journey to discover the methods for finding the max value in a dictionary in Python. I hope this post has provided you with valuable insights and practical techniques that you can apply in your future projects. Keep exploring, experimenting, and pushing the boundaries of what you can achieve with Python.
Resources
Here are some Python resources that you may find good:
- How to Read a File in Python, Write to, and Append, to a File
- Rename Files in Python: A Guide with Examples using os.rename()
- How to use Python to Perform a Paired Sample T-test
- Pip Install Specific Version of a Python Package: 2 Steps
- How to Convert JSON to Excel in Python with Pandas
- Python Scientific Notation & How to Suppress it in Pandas & NumPy
- Wilcoxon Signed-Rank test in Python