In this tutorial, you will learn how to use pip to install a specific version of a Python package. The post’s outline (as seen in the ToC) is as follows. First, you will get a brief introduction with examples of when you might need to install e.g., an older package version. Second, you will get the general syntax for how to carry out this task. After that, you will get two steps to install specific versions of Python packages with pip. This section will also teach you how to work in a virtual environment. In the next section, we will look at specifying the version of multiple Python packages by creating a .txt file.
Table of Contents
- Outline
- Why install an older version of a package?
- Pip’s Syntax for Installing a Specific Version of a Package
- Two Steps to Install Specific Version of a Package with Pip:
- Dealing with Multiple Packages and Installing Specific Versions
- Conclusion
- Resources
Outline
This post is structured as follows to guide you through the process of using pip to install a specific version of a package. In the first section, we will explore the rationale behind using pip to downgrade a package and highlight why we might want to have an older version of a package in specific scenarios. Following this, we will learn about pip’s syntax for installing a particular package version, clarifying how this process works.
The post contains two steps outlining the procedure for installing a specific package version with pip. Step 1 emphasizes the importance of creating a virtual environment using virtualenv, ensuring a controlled environment for version management. Step 2 walks you through the precise process of using pip to install the desired package version within the virtual environment.
The post also addresses situations involving multiple packages and their respective versions. We discuss strategies for managing different package versions and offer insights into potential challenges in such scenarios.
Why install an older version of a package?
Now, there may be several reasons that you may want to install a specific version of a Python package. For example, you may need to install an older version of a package if the package has changed in a way that is incompatible with the version of Python you have installed, with other packages you have installed, or with your Python code. As previously mentioned, we will work with the package manager, pip. Still, installing a specific package version is also possible if you use other package managers. For example, using the package manager conda (Anaconda Python distribution) is also possible.
Here are some instructions on how to use pip to install a specific (e.g., older) version of a Python package:
Pip’s Syntax for Installing a Specific Version of a Package
Here’s the general Pip syntax that you can use to install a specific version of a Python package:
pip install <PACKAGE>==<VERSION>
Code language: Bash (bash)
As you may understand, now, you exchange “<PACKAGE>” and “<VERSION>” for the name of the package and the version you want to install, respectively. Don’t worry, the next section will show you, by example, more exactly how this is done.

If you get the warning, as in the image above, you can upgrade pip to the latest version: pip --install upgrade pip
. Before getting into more details, here is how to install a specific version of a Python package:
To install a specific version of a Python package, you can use pip: pip install YourPackage==YourVersion
. For example, if you want to install an older version of Pandas, you can do as follows: pip install pandas==1.1.3. Of course, you must open up, e.g., Windows Command Prompt or your favorite terminal emulator in Linux. Note it is also possible to use conda to install a certain package version.
In the next section, you will learn two important steps for installing a certain Python package version using pip package manager. First, you will learn how to install and create a virtual environment. Second, you will learn how to use pip to install a version you need of a Python package using the syntax you’ve already learned.
Two Steps to Install Specific Version of a Package with Pip:
In this section, you will learn how to install an older Python package version using pip. First, I would recommend creating a virtual environment. Therefore, you will first learn how to install the virtual environment package, create a virtual environment, and install a specific version of a Python package.
1) Install virtualenv and create an environment
First, you should install the virtualenv package. Here’s how to install a Python package with pip:
pip install virtualenv
Code language: Bash (bash)

In the code chunk above, we install a Python package called “virtualenv” pip.
Virtualenv is a tool used to create isolated Python environments. We use it to create a separate environment for each project, with its dependencies and Python version. This helps avoid conflicts between projects requiring different versions of the same package.
When we run this command, pip will download the virtualenv package from the Python Package Index (PyPI) and install it on your system. Once the installation is complete, you can use the virtualenv command to create new virtual environments for your Python projects.
Here is how we create and then activate a virtual environment:
virtualenv myproject
source myproject/bin/activate
Code language: Bash (bash)
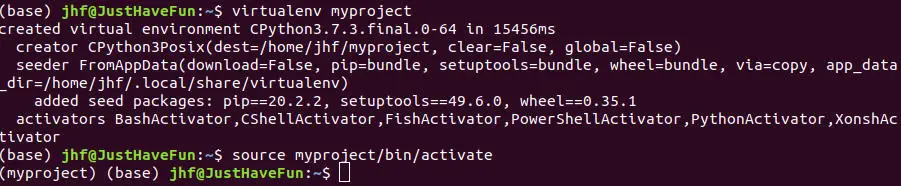
Now that your virtual environment is set up, you can go on to the next step and install an older Python package version. In step two, we use pip again (like when installing virtualenv), but now we will also use the general syntax we’ve learned earlier in this post.
2) Install the Specific Version you Need with Pip
Now that your virtual environment is ready to use. Here’s how to use pip to install a specific version of the package Pandas:
# Use pip to install a specific version:
pip install pandas==1.1.1
Code language: Bash (bash)
In the code chunk above, we can see an example when we run pip in the command-line interface (CLI) to install a specific version of the Pandas library for Python.
Here’s a breakdown of the code snippet:
pip
is a package installer for Python.install
is the command being executed.pandas
is the name of the library being installed.==1.1.1
is a version specifier that tellspip
to install version 1.1.1 of the Pandas library.
So when you run pip install pandas==1.1.1
in your command-line interface, pip
will download and install version 1.1.1 of the Pandas library. Voila! We have successfully installed a specific version of a package using pip.
It is, of course, possible to add more packages and their versions if you have many packages that you want to install a certain version of. However, this may be cumbersome, and in the next section, we will look at how to install older versions of multiple packages. That is when storing them in a text file.
Dealing with Multiple Packages and Installing Specific Versions
That was pretty simple, but using the above steps may not be useful if you, for instance, need to install many Python packages. When we are installing packages using pip we can create a .txt file (e.g., requirements.txt). Here’s an example text file with a few Python packages and their versions:
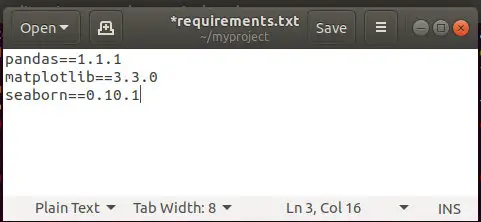
As you can see, you should keep each package on one line in the text file. Moreover, you should follow the syntax you’ve learned earlier in the post. This is also evident in the image above. Here’s how to install a specified version of multiple packages using the text file:
# Pip install specific versions of multiple packages:
pip install -r myproject/requirements.txt
Code language: Bash (bash)
The code chunk above is a command in a command-line interface (CLI) to install Python dependencies for a project.
Here’s a breakdown of the code chunk:
- As we already know
pip
is a package installer for Python. install
is the command being executed.-r
stands for “requirements”, and it will makepip
read the list of dependencies from a file instead of specifying them directly on the command line.myproject/requirements.txt
is the file that contains the list of dependencies.
So when you run pip install -r myproject/requirements.txt
in your command-line interface (i.e., bash), pip
will read the requirements.txt
file in the myproject
directory and install all of the dependencies listed in that file.
Installing an older version of one package can lead to problems with the package’s dependencies. You will still get the newest versions of the dependencies. That is, the version you use allows, of course. One backside of this is that it can later break your application or workflow. Luckily, there are some solutions to combat this issue. For example, if you want your data analysis reproducible, using Binder, Jupyter Notebooks, and Python may be a solution. However, if you are developing applications, you may need another strategy. In the last section, we will have a look at another Python package that may be useful: Pipenv (see resources at the bottom for a great tutorial on Pipenv).
Conclusion
In this brief Python tutorial, you learned how to use pip to install a certain package version. First, you learned the syntax of pip for specifying a version. After that, you learned how to 1) create a virtual environment and 2) install the version of a package you needed. In the final section, we looked at how to deal with multiple packages of certain versions. How to set the version of multiple packages you wanted to install.
Please comment below if you have any suggestions or corrections to the current post. I always appreciate it when I get to learn from others.
Resources
Here are some useful packages and tutorials, as well as the documentation that may be worth having a look at:
- Pipx: Installing, Uninstalling, & Upgrading Python Packages in Virtual Envs
- Pipenv
- Virtualenv documentation
- Pip documentation
- Pipenv guide
Congratulation for the excellent tutorial.
Thanks Vasco for your kind words.