In this tutorial, we will learn the basics of installing, working and updating packages in Python. First, we will learn how to install Python packages, then how to use them, and finally, how to update Python packages when needed. More specifically, we are going to learn how to install and upgrade packages using pip, conda, and Anaconda Navigator.
Table of Contents
- Python Packages
- Installing Packages in Python
- How to install a Python Package using Anaconda Navigator
- Bonus: Installing Python Packages in a Virtual Environment
- How to use a Python Package
- What Python Packages are Installed Now?
- Updating Python Packages
- Conclusion:
- Tutorials
Python Packages
Now, before we are going to learn how to install Python packages we are going to answer the question “what is a package in Python?”
Python packages are collections of modules. More specifically, a package typically contains Python functions, methods, and sometimes data, that are packaged in a well-defined format. When we install a package, it gives us access to a set of functions or methods that are not available in the base Python
pip is a standard package-management system that we can use to install and manage software packages written in Python. Many Python packages can be found in the default source for packages and their dependencies — Python Package Index (PyPI). pip is an acronym and stands for “Pip Installs Packages”. That is, we use pip to install Python packages. For example, pip install pandas will install the Python package Pandas and it’s dependencies.
Installing Packages in Python
Several methods enable us to install Python packages. As previously mentioned, in this post we will learn how to install Python packages using pip, conda package manager, and Anaconda Navigator. Note, if using conda package manager or Anaconda Navigator we need to have the Python distribution Anaconda installed.
How to install a Python Package using pip.
First, we are going to learn how to install Pandas using pip. It’s quite easy! If we are using Windows we start up the Command Prompt. Note, this is done by pressing the Windows icon in the left lower corner (or on your keyboard) and typing “Command Prompt”.
When we have the command prompt started we type the following code and press enter: pip install pandas
.
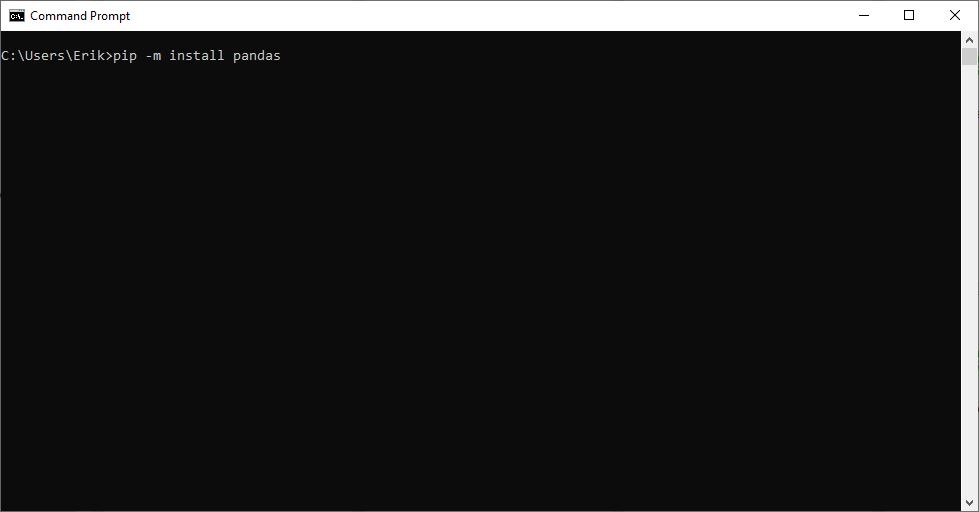
If we want to install multiple Python packages at the same time, we just add them after the first:
pip -m install pandas matplotlib seaborn
Code language: Bash (bash)
Note, it is also possible to use pip to install a specific version of a Python package. For example, you can add “==” after the package name followed by the version of the package you want to install.
How to install a Python Package using conda
Second, we are going to learn how to install a Python package using conda. This is also very easy. First, we start up the Anaconda prompt. As in the example above, we press the Windows icon but this time we type “Anaconda Prompt” and hit enter. In the Anaconda prompt, we type conda install -c anaconda pandas
.
Note, here we can choose which repository we want to install the Python packages from. In the example above, we used the anaconda repository. However, if we want to use another repo we can, of course, do this. For instance, if we want to use conda-forge, the community-led repo, to install Python packages we type the following command:
conda install -c conda-forge pandas
Code language: Bash (bash)
How to install a Python Package using Anaconda Navigator
Finally, we are going to learn to install Python packages using the Anaconda Navigator. To start Anaconda Navigator, we need to have Anaconda installed (see above) and we press the windows icon, down to the left. Here we type, “Anaconda Navigator” and press enter (or click on the app). Basically, there are 7 steps to install a Python package using Anaconda Navigator.
7 Steps to Installing a Python Package in Anaconda Navigator
- With the Anaconda Navigator started, press “Environments”.
- Select your environment, create a new one, or just leave it as it is (base environment)
- Click on the dropdown menu
- Click on “Not Installed”
- Search for Pandas and hit enter,
- Click on the check icon, when Pandas is found
- Click “Apply” in the bottom right corner
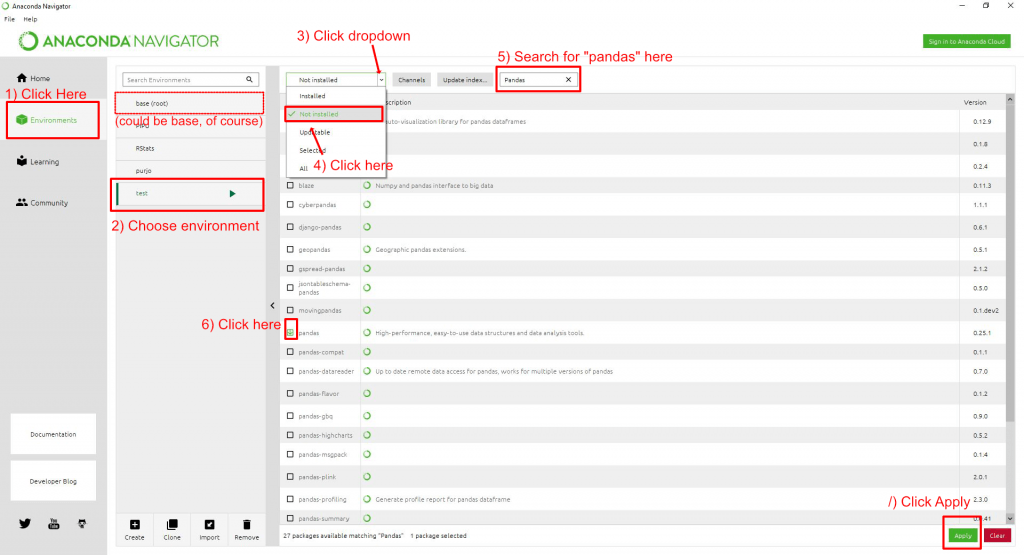
Bonus: Installing Python Packages in a Virtual Environment
Now, as a bonus, we will learn how to create an environment, using conda, and install Python packages in this environment. First, we start up the Anaconda Prompt.
Creating a Python Environment using conda
Now, that we have the Anaconda Prompt we can continue to create a Python environment. Creating a Python environment is easy when we are using conda. We just type the following command:
conda create -n pandasenv
Code language: Bash (bash)
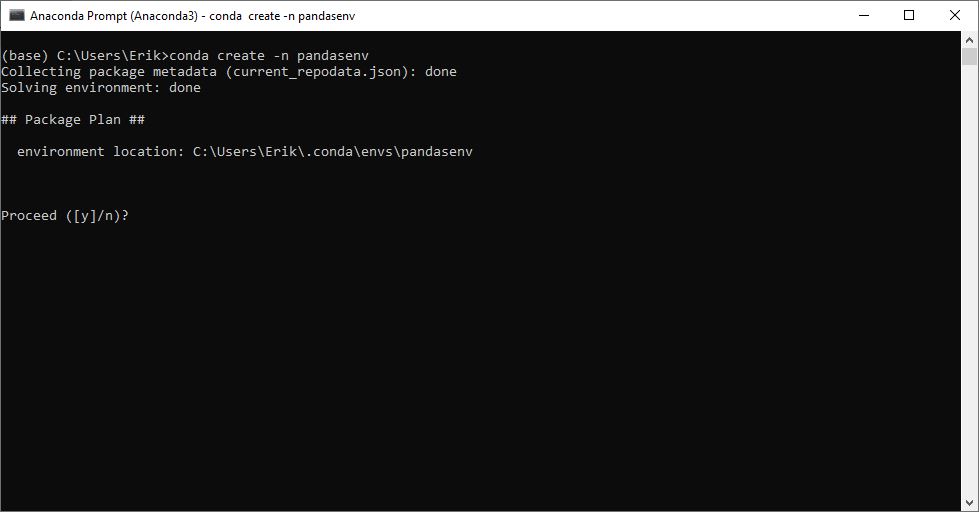
Note, “pandasenv” is the name of the Python environment. If we want our Python environment to have any other name we just change this to the name we want.
Activating the Python Environment
Next, we need to activate the environment. Now, we just typeconda activate pandasenv
to activate our Python environment.
Installing a Python package in a Python Environment
Of course, installing Python packages, using conda, in an environment is not any different than using conda to install Python packages in the base environment. We type conda install -c anaconda pandas
.
Note,
however, if we can also install multiple Python packages when creating the
environment. For instance, we may want to install Pandas, matplotlib, seaborn,
and statsmodels. In this case, what we will do, is just type conda create -n
pandasenv pandas matplotlib seaborn statsmodels
How to use a Python Package
Once a Python package is installed (basically the functions, methods, and so on, are downloaded to your computer), we need to “call” the package into the current session of Python. This is essentially like saying, “Hey Python, we will be using these functions and methods now, please have them ready to go”. Note, that we have to do this every time we start a new Python script or session, so this should be at the top of our script.
When we want to call a package, use import <packagename>
or often we follow some kind of convention and type import <packagename> as <abbrevation> For instance after we have installed Pandas we can start up our favorite Python IDE or a Jupyter Notebook. At the beginning of the script, we type import pandas as pd
at the top of the script.
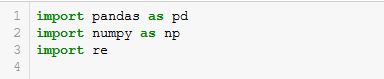
What Python Packages are Installed Now?
If we are ever curious about what Python packages we have installed on our computers, we can, of course, check this. In this section, we will learn how to list all the installed Python packages using pip and conda.
List all Installed Python Packages using pip
Here, we are going to use pip to list all the installed Python packages. Note, in the example here, we use pip in a Python environment and there are not many packages installed. If we have a lot more packages installed, the result will, of course, look different. Here’s one way to get all the installed Python packages using pip:
pip list
Code language: Bash (bash)
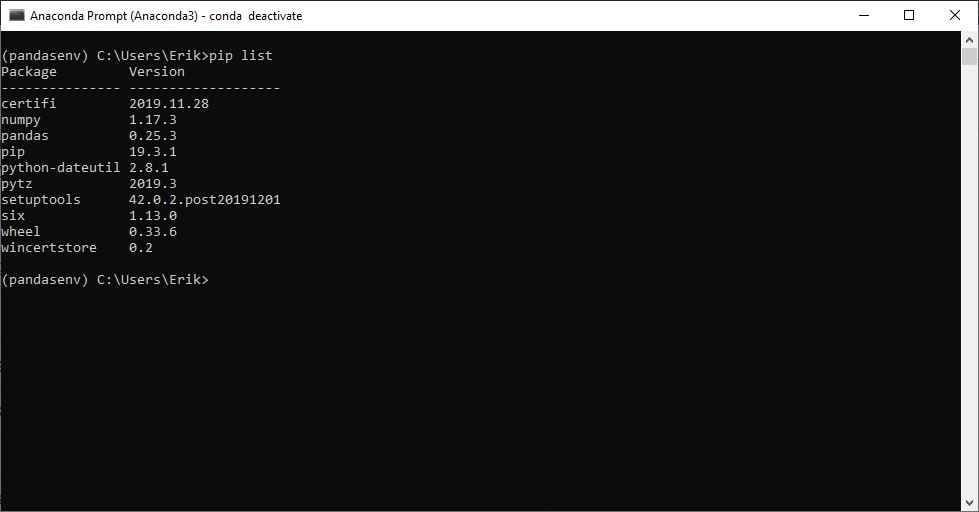
See all Installed Python Packages using conda
In this subsection, we are going to use conda to see all the Python packages we have installed. This is very simple. We type conda list
.
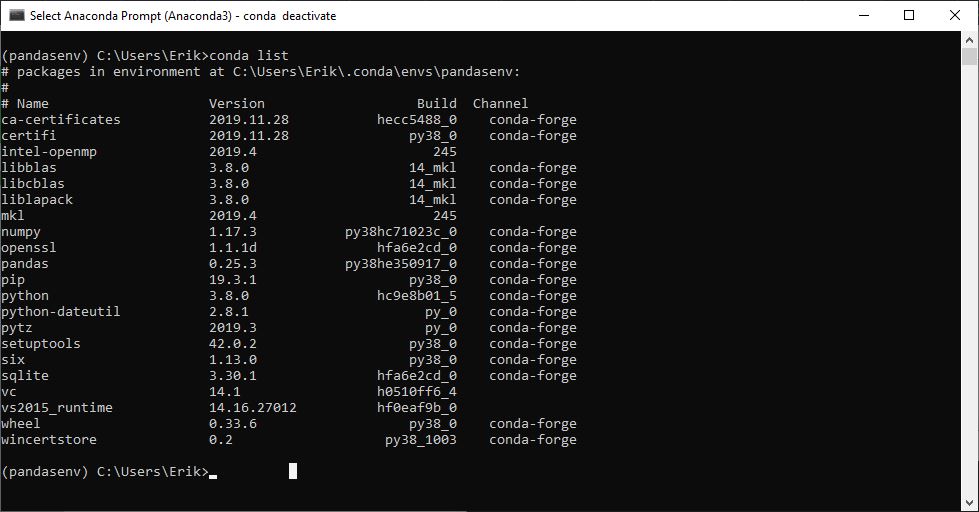
If we have Anaconda installed, we can also check out the Environments tab. Here we can check what Python packages we have installed in our different environments. We just leave the dropdown on “Installed” and we can scroll down to see all the installed packages.
Updating Python Packages
In this section, we will learn how to update Python packages using pip and conda. This is handy as sometimes packages are updated by the users who created them. Updating packages can sometimes make changes to both the package and also to how your code runs. Before we update a Python package, we need to make sure we know what any changes are new and how potentially may affect our code. That is, if we have a lot of Python code, upgrading a Python package may completely change the behavior, or functionality, of our code.
Conda: Updating Python Packages
If we have Anaconda installed, we can update a Python package using conda. More specifically, we use the command conda update <packagename>
. For instance, if we need to update the Python package statsmodels, we type conda update statsmodels
.
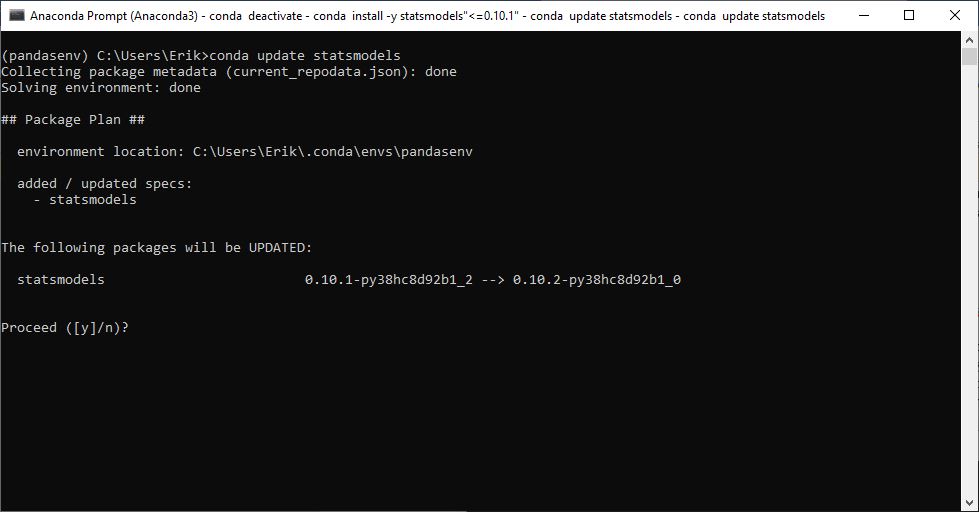
Updating all Python Packages using conda
If we want to update ALL installed Python packages, we remove the package name and add the “—all” argument:
conda update --all
Code language: Bash (bash)
Upgrading Python Packages using Pip
Finally, we are going to learn how to update packages using pip. It is also very simple. When we need to update a Python package using pip we just type pip install -–upgrade
and we will upgrade this package. For example, if we want to upgrade the Python package pingouin we type pip install –-upgrade pingouin
.
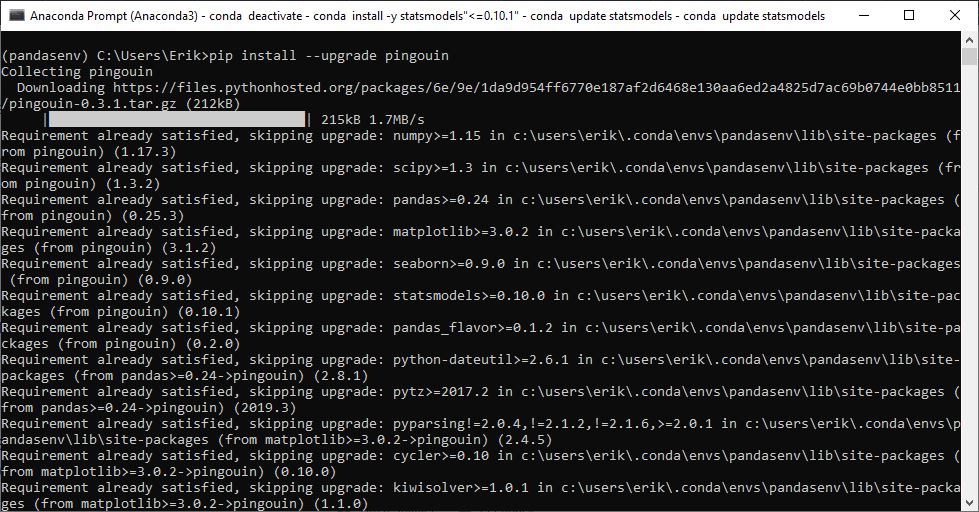
Sometimes, of course, pip gets an update and we may want to update pip. This can be done, using pip itself, the conda package manager, and the Anaconda Navigator. See more about this in the post 3 easy methods to update pip.
Conclusion:
In this post, we have learned how to install Python packages, how to use them, and how to upgrade Python packages.
Tutorials
- Find the Highest Value in Dictionary in Python
- Coefficient of Variation in Python with Pandas & NumPy
- How to use Square Root, log, & Box-Cox Transformation in Python
- How to Convert JSON to Excel in Python with Pandas
- How to Convert a Python Dictionary to a Pandas DataFrame