In Python, it is possible to print numbers in scientific notation using base functions and NumPy. Specifically, using three different methods, you will learn how to use Python to print large or small (i.e., floating point) numbers in scientific notation. In the final two sections, before concluding this post, you will also learn how to suppress scientific form in NumPy arrays and Pandas dataframe.
Table of Contents
- Outline
- Requirements
- Python Scientific Notation with the format() function
- Python Scientific Form with fstrings
- Scientific Notation in Python with NumPy
- How to Suppress Scientific Notation in NumPy Arrays
- How to Suppress Scientific Form in Pandas Dataframe
- Conclusion
- Other Useful Python Tutorials:
Outline
As mentioned, this post will show you how to print scientific notation in Python using three different methods. First, however, we will learn more about scientific notation. After this, we will have a look at the first example using the Python function format()
. In the next example, we will use fstrings
to represent scientific notation. In the third and final example, we will use NumPy. After these three examples, you will learn how to suppress standard index form in NumPy arrays and Pandas dataframes.
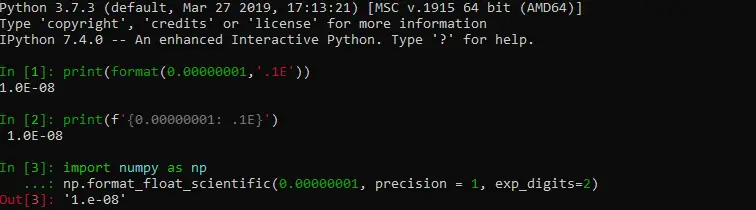
Requirements
To follow this post, you need to have a working Python installation. Moreover, if you want to use fstrings
you need to have at least Python 3.6 (or higher). If you want to use the Python package NumPy to represent large or small (floating numbers) in scientific notation, you must install this Python package. In Python, you can install packages using pip:
pip install numpy
Code language: Bash (bash)
In the next section, we will learn more about scientific notation and then look at the first example using the format()
function.
Scientific notation, also known as scientific form, standard index form, or standard form (in the UK), is used to represent numbers that are either too large or too small to be represented in decimals.
To remove scientific notation in Python, we can use the NumPy package and the set_printoptions
method. For example, this code will suppress scientific notation: np.set_printoptions(suppress=True)
.
Python Scientific Notation with the format() function
Here’s how to represent scientific notation in Python using the format()
function:
print(format(0.00000001,'.1E'))
Code language: Python (python)
Typically, the format()
function is used when you want to format strings in a specific format. In the code chunk above, the use of the format()
function is pretty straightforward. The first parameter was the (small) number we wanted to represent in scientific form in Python. Moreover, the second parameter was used to specify the formatting pattern. Specifically, E indicates exponential notation to print the value in scientific notation. Moreover, .1 is used to tell the format()
function that we want one digit following the decimal. Here are two working examples using Python to print large and small numbers in scientific notation:
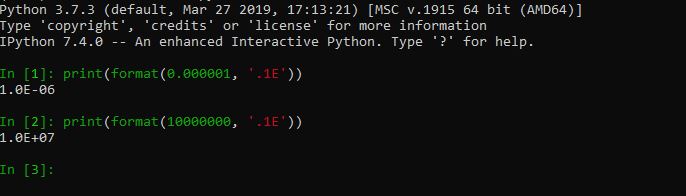
Now, if we want to format a string we can use the format()
function like this:
'A large value represented in scientific form in Python: {numb:1E}'.format(numb=1000000000000000000)
Code language: Python (python)
Notice how we used the curly brackets where we wanted the scientific notation. Now, within the curly braces, we added numb and, again, .1E (for the same reason as previously). In the format() function, we used numb again, and here we added the number we wanted to print as standard index form in Python. In the next section, we will use Python’s fstrings
to print numbers in standard index form.

Python Scientific Form with fstrings
Here’s another method you can use if you want to represent small numbers as scientific notation in Python:
print(f'{0.00000001: .1E}')
Code language: Python (python)
The syntax in this example is fairly similar to the one we used in the previous example. Notice, however, how we used f
prior to single quotation marks. Within the curly braces, we put the decimal number we want to print in scientific form. Again, we use .1E
similarly as above. To tell fstrings that we want to be formatted in scientific notation. Here are two examples in which we do the same for both small and large numbers:

Remember, fstrings
can only be used if you have Python 3.6 or higher installed, and it will make your code a bit more readable compared to when using the format()
function. In the next example, we will use NumPy.
Scientific Notation in Python with NumPy
Here’s how we can use NumPy to print numbers in scientific notation in Python:
import numpy as np
np.format_float_scientific(0.00000001, precision = 1, exp_digits=2)
Code language: Python (python)
In the code chunk above, we used the function format_float_scientific()
. Here we used the precision parameter to specify the number of decimal digits
and the exp_digits
to tell how many digits we want in the exponential notation. Note, however, that NumPy will default print large and small numbers in scientific form. In the next and last example, we will look at how to suppress scientific notation in Python.
How to Suppress Scientific Notation in NumPy Arrays
Here’s how we can suppress scientific form in Python NumPy arrays:
import numpy as np
# Suppressing scientific notation
np.set_printoptions(suppress=True)
# Creating a np array
np_array = [np.random.normal(0, 0.0000001, 10),
np.random.normal(0, 1000000, 10)]
np_array
Code language: Python (python)
In the example here, we first created a NumPy array (a normal distribution with ten small and ten large numbers). Second, we used the set_printoptions()
function and the parameter suppress. Setting this parameter to True will print the numbers “as they are”.
In the next and final example, we will look at how to suppress scientific notation in Pandas dataframes.
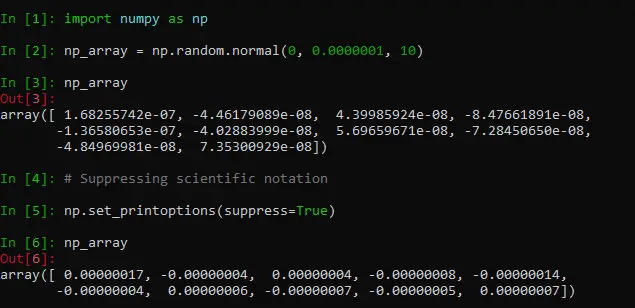
How to Suppress Scientific Form in Pandas Dataframe
Here is how we can use the set_option() method to suppress scientific notation in Pandas dataframe:
import pandas as pd
pd.set_option('display.float_format', '{:20.2f}'.format)
df = pd.DataFrame(np.random.randn(4, 2)*100000000,
columns=['A', 'B'])
Code language: Python (python)
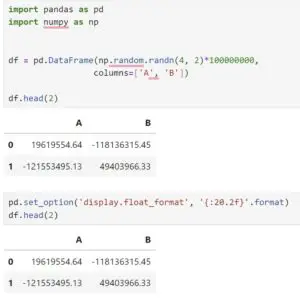
In the code chunk above, we used Pandas dataframe method to convert a NumPy array to a dataframe. This dataframe, when printed, will show the numbers in scientific form. Therefore, we used the set_option()
method to suppress this print. It is also worth noting that this will set the global option in the Jupyter Notebook. There are other options as well, such as using the round()
method.
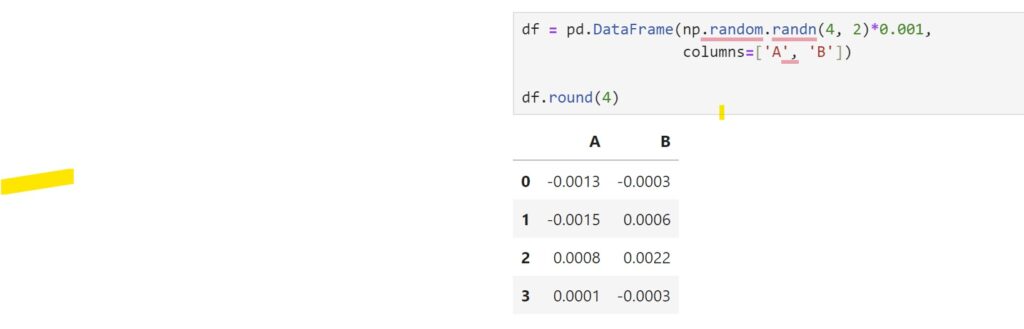
Conclusion
In this post, you have learned how to use Python to represent numbers in scientific notation. Specifically, you have learned three different methods to print large and small numbers in scientific form. After these three examples, you have also learned how to suppress scientific notation in NumPy arrays and Pandas Dataframes. I hope you learned something valuable. If you did, please leave a comment below and share the article on your social media channels. Finally, if you have any corrections or suggestions for this post (or any other post on the blog), please comment below or use the contact form.
Other Useful Python Tutorials:
Here are some other helpful Python tutorials:
- How to get Absolute Value in Python with abs() and Pandas
- Create a Correlation Matrix in Python with NumPy and Pandas
- How to do Descriptive Statistics in Python using Numpy
- Pipx: Installing, Uninstalling, & Upgrading Python Packages in Virtual Envs
- How to use Square Root, log, & Box-Cox Transformation in Python
- Pip Install Specific Version of a Python Package: 2 Steps