In this Python tutorial, we will learn how to get the absolute value in Python. First, we will use one of Pythons built-in functions abs() to do this. In this section, we will go through a couple of examples of how to get the absolute value. Second, we will import data with Pandas and use the abs() method to get the absolute values in a dataframe.
Table of Contents
- Outline
- Prerequisites
- Python abs() Function
- Python abs syntax
- How to get Absolute Value in Python with abs() Example 1
- Python abs() Example 2
- Use Python’s math (fabs()) to Ge Absolute Value
- Python get Absolute Values in Python using Pandas
- Absolute Value in Python YouTube Tutorial:
- Conclusion to the Python Absolute Value Tutorial
- Resources
Outline
The structure of the post is as follows. First, we establish the prerequisites needed to understand and apply the concepts covered in this guide. Familiarity with basic Python syntax and functions is beneficial as we explore the versatile abs()
function.
We begin by comprehensively introducing Python’s abs(
) function and discussing its syntax. This foundation prepares us to demonstrate how to get the absolute value in Python through practical examples. Example 1 provides a step-by-step breakdown of the process, showcasing the function’s straightforward application. In Example 2, we further solidify our understanding by highlighting alternative scenarios where the abs()
function proves useful.
We will learn about Python’s math module to expand our exploration, explicitly focusing on the fabs()
function to obtain absolute values.
Next, we we will learn how to get the absolute value using Pandas. Through examples, we showcase how to apply the abs()
function to Pandas Series, enabling efficient calculations across large datasets. In Examples 1 and 2, we navigate through practical scenarios that demonstrate the utility of Pandas in calculating absolute values.
As we continue to use Pandas, Example 3 illustrates the application of absolute value calculations to dataframe columns. This comprehensive approach ensures you can handle various data formats and structures.
To provide diverse learning options, we offer a video tutorial that visually guides you through calculating absolute values in Python. This engaging format enhances your understanding and reinforces your ability to utilize the concepts covered confidently.
Prerequisites
In this post, we are going to use the built-in function abs()
and fabs()
. The latter function is part of the math module and, therefore, we don’t need to install anything. However, if you plan on working with Pandas, you must install this Python package.
Now, before we go on with the examples of how to get the absolute value of a number using Python, we will answer to questions.
Python abs() Function
The Python abs()
function is one of the math functions in Python. This function will return the positive absolute value of a specific number or an expression. In the next sections, we will see plenty of examples of how to get the absolute value in Python. First, however, we will look at the syntax of the abs() function.
Python abs syntax
The syntax of the abs()
function is shown below. Here is how to get the absolute value in Python:
# Get absolute value of x
abs(x)
Code language: Python (python)
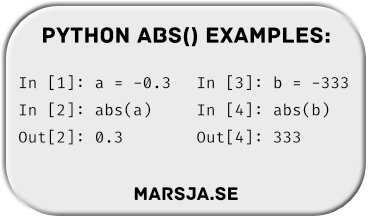
Now, x can be any number we want to find the absolute value. For instance, if x is positive or negative zero, Pythons abs()
function will return a positive zero.
If we, however, put in something that is not a number, we will get a TypeError (“bad operand type for abs(): ‘str’”.
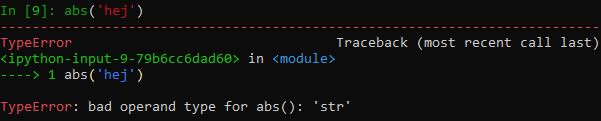
How to get Absolute Value in Python with abs() Example 1
The abs function will enable us to find the absolute value of a numeric value. Here is how to get the absolute value of an integer:
# Print the absolute value of an integer
abs(-33)
Code language: Python (python)
As you can see, it is pretty simple to work with the abs() method. That is, you type the method and put your negative number between the parentheses and press enter.
Python abs() Example 2
Now, if we have a list of numbers, we cannot use the abs() function as we did in the first example. Note, if we do we get a TypeError, again. Thus, in this example, we will use Python list comprehension and the abs() function.
# List of negative integers
numbers = [-1, -2.1, -3, -444]
# Get the absolute values from ints in a list:
[abs(number) for number in numbers]
Code language: Python (python)
In the code chunk above, we first created a list with negative numbers. Second, we used Python list comprehension to loop through these numbers and used the abs()
function on each separate number.
Use Python’s math (fabs()) to Ge Absolute Value
Note it is also possible to import the math module and use the fabs()
method to get the absolute value of a number in Python. However, when using fabs()
, we will get the absolute value as a float:
import math
# Get absolute value with fabs() from math:
math.fabs(-33)
Code language: Python (python)
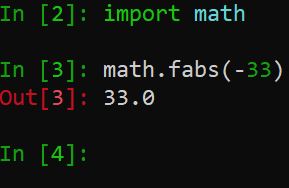
Note that using math and the fabs() function to get the absolute value results in a float number!
Python get Absolute Values in Python using Pandas
Now, if we want to get absolute values from a dataset we can use Pandas to create a dataframe from a datafile (e.g., CSV). In this Python absolute value example, we will find the absolute values for all the records present in a dataframe. First, we will use Python to get absolute values in one column using Pandas abs method. Second, we will do the same but for two columns in the dataset. Finally, we will get the absolute values for all columns in the Pandas dataframe.
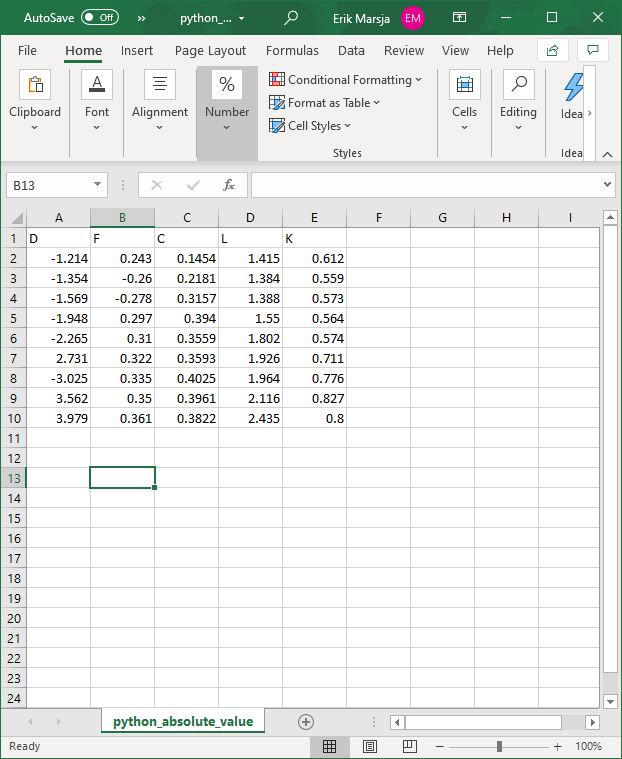
Now, for this absolute value in Python example, we will use the CSV data in the image above. If needed, see the post about Pandas read csv method to understand the steps in importing data from a CSV file. Here’s how to do it with the example file (python_absolute_value.csv):
import pandas as pd
# Import data from .csv
df = pd.read_csv('python_absolute_value.csv')
Code language: Python (python)
Now, when we have the data loaded, we are ready to get the absolute values using Python Pandas.
Python Pandas Absolute Values Example 1
In the first Python absolute values example using Pandas, we are going to select one column (“D”):
# Absolute value from one column
df['D'].abs()
Code language: Python (python)
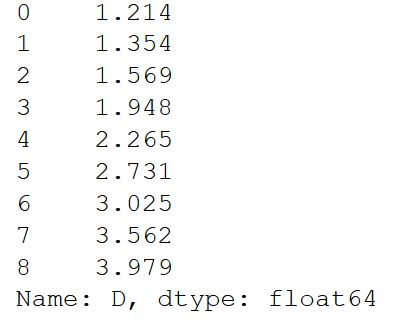
Python Pandas Absolute Values Example 2
Now, in the second absolute value in Python example, we are going to select two columns (“D” and “F”):
# Absolute value from two columns
df[['D', 'F']].abs()
Code language: Python (python)
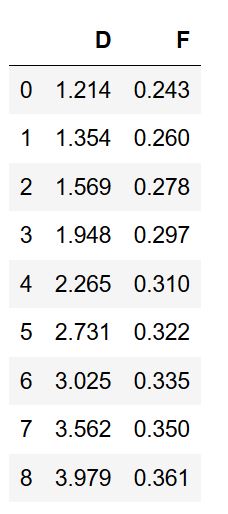
Now, if needed, there is more information about slicing and indexing Pandas dataframes in that post.
Python Pandas Absolute Values Example 3
Finally, we are going to get the absolute values from all columns in the Pandas dataframe:
df.abs()
Code language: Python (python)
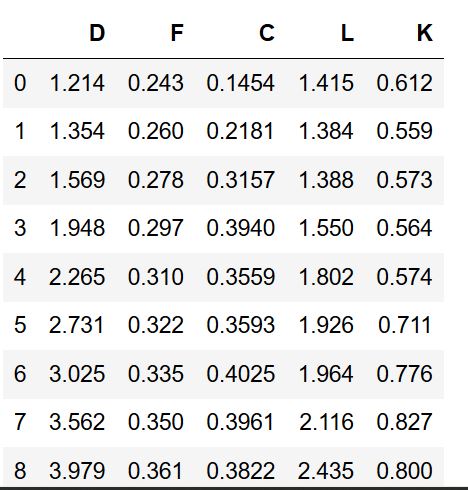
Absolute Value in Python YouTube Tutorial:
Here’s a YouTube tutorial explaining, by examples, how to get the absolute value in Python:
Conclusion to the Python Absolute Value Tutorial
In this post, we have learned about the concepts and techniques associated with calculating absolute values in Python. The structure of the post was organized as follows. We began by establishing the prerequisites for comprehending the guide’s content
We started with a comprehensive introduction to Python’s abs()
function. Then, we looked at its syntax and fundamental usage, preparing the ground for understanding how to obtain the absolute value in Python through practical examples. In the first example, we provided a step-by-step breakdown of the process, highlighting its straightforward application. A second example showcased alternative scenarios where the abs()
function proved beneficial.
As our exploration deepened, we ventured into Python’s math module, specifically focusing on the fabs()
function to obtain absolute values. This module allowed us to handle more complex mathematical operations while maintaining precision.
Then, we learned how to acquire absolute values using Pandas. Through examples, we demonstrated how the abs()
function can be applied to Pandas Series, enabling efficient calculations across extensive datasets. The examples illustrated its utility in various scenarios.
Additionally, we explored the application of absolute value calculations to dataframe columns in Pandas. This comprehensive approach ensured that readers could handle diverse data formats and structures.
Resources
Here are some more Python tutorials:
- Python Check if File is Empty: Data Integrity with OS Module
- Coefficient of Variation in Python with Pandas & NumPy
- Pandas Count Occurrences in Column – i.e. Unique Values
- How to Convert a NumPy Array to Pandas Dataframe: 3 Examples
- How to Make a Column Index in Pandas Dataframe – with Examples