In this post, we will learn how to remove a specific row in R using both base functions and the popular dplyr package. Previously, we learned how to remove a row from a dataframe with conditions and delete duplicated rows using dplyr. In this post, we will extend our data manipulation skills by understanding various methods to remove specific rows in R. Whether you are dealing with missing values or refining your dataset for analysis, this post should have you covered. Let us get into the basics and explore practical examples.
Table of Contents
- Outline
- Prerequisites
- Base R Examples of Removing a Specific Row in R
- Examples of using dplyr to remove specific row in R
- Base R vs. dplyr
- Summary
Outline
The post is structured as follows. First, we look at Base R examples demonstrating how to remove a specific row. Then, we explore techniques such as removing a row by index and excluding rows with NA values in specific columns. Following this, we transition to dplyr, showcasing its effectiveness in removing specific rows in R. With the slice()
function, we detail how to eliminate a specific row by index. Subsequently, we demonstrate using dplyr to remove rows based on NA values, both in a specific column and across any column. The examples offer practical insights into using base R and dplyr for efficient row removal, catering to diverse data manipulation scenarios.
Prerequisites
Prerequisites for this post include a basic understanding of R scripting, including writing and saving scripts. Familiarity with loading data into R is essential, but worry not if you’re new to certain coding concepts; each code chunk will be thoroughly explained. A fundamental grasp of R’s syntax and basic data manipulation concepts will enhance your experience, making it easier to follow. As we explore examples using base R and dplyr, these prerequisites ensure you can confidently navigate the code and grasp the presented techniques.
Base R Examples of Removing a Specific Row in R
Here are three examples using base functions to remove a row in R:
1. Remove a Row by Index
Here is how we remove the third row in a dataframe:
# Example 1: Remove row by index
data <- data[-c(3), ]
Code language: R (r)
In the code chunk above, we employed base R to remove a specific row using index-based referencing. We use the square brackets []
for subsetting in R, and the c()
function creates a vector containing the row index to be removed. In this case, we removed the third row from the data
dataframe. It is important to note that the comma after the index ensures that we are also specifying the columns (in this case, we are selecting all columns).
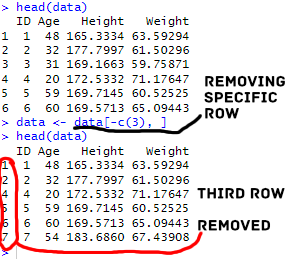
2. Remove Row if NA in Specific Column
Here is how to use base R to remove a row if NA in a specific column:
# Example 2: Remove row if NA in the 'Age' column
data <- data[!is.na(data$Age), ]
Code language: R (r)
In this code chunk, we used base R to eliminate rows with missing values in the ‘Age’ column. Building upon the first example, we continued using square brackets []
for subsetting. The !
symbol signifies the logical NOT operator, and is.na(data$Age)
creates a logical vector, identifying rows where the ‘Age’ column has missing values. By incorporating this vector into the subsetting operation, we removed all rows containing NA in the ‘Age’ column.
Remove Row if NA in Any Column
Here is how we remove a row if we have missing values (NA) is in any column:
# Example 3: Remove row if NA in any column
data <- data[complete.cases(data), ]
Code language: CSS (css)
In this example, we extended our base R approach to remove rows containing missing values in any column. Here, we used the function complete.cases(data)
which generates a logical vector. This vector contains rows without any missing values across all columns. By applying this vector within square brackets []
, we selected only those rows with complete cases,
eliminating rows with NA in any column. We can also remove a row with missing values in all important columns:
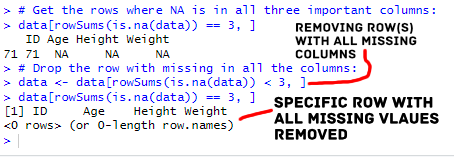
Examples of using dplyr to remove specific row in R
We can use dplyr for the same data manipulation task as when we use base R functions:
1. Remove Specific Row with the slice() function
Here is how we can remove specific row in R with dplyr’s slice() function:
# Example 4: Remove row by index using dplyr
data <- data %>%
slice(-3)
Code language: R (r)
In the code snippet above, we use the %>%
(pipe) operator to perform operations on the dataframe using the dplyr package. With slice(-3)
, we use the slice()
function to remove the row at index three from the dataframe. The %>%
operator allows us to chain operations, making it clear that we’re modifying the dataframe in a sequential manner.
2. Remove Row if NA in Specific Column
# Example 5: Remove row if NA in the 'Age' column using dplyr
data <- data %>%
filter(!is.na(Age))
Code language: R (r)
In the snippet above, we used the filter()
function to keep rows where the ‘Age’ column is not missing (!is.na(Age
)). This builds on the %>% operator, offering a more streamlined and readable approach than base R.
3. Remove Row if NA in Any Column
Here is how to use R to remove a row if NA in any of the columns:
# Example 6: Remove row if NA in any column using dplyr
data <- data %>%
drop_na()
Code language: R (r)
In the code snippet above, we use drop_na()
from the dplyr package. This function efficiently removes rows containing any missing values in the dataset. It is a concise and intuitive method provided by dplyr to handle missing data, offering a cleaner alternative to the base R approach (i.e., in example 3).
Base R vs. dplyr
There are trade-offs when considering whether to use dplyr or base R for data manipulation. Base R offers self-sufficiency; it does not rely on external packages, making it suitable for environments with installation restrictions. Here, we do not rely on maintaining external packages such as dplyr as well. However, this independence comes with limitations. Base R might require more code and lack the streamlined functionality of dplyr, which is part of the Tidyverse.
dplyr, on the other hand, offers an intuitive syntax, promoting readable and concise code. It seamlessly integrates with other Tidyverse packages, providing additional tools for tasks like selecting columns, removing variables in R, and calculating observations in R. While depending on external packages may pose maintenance concerns, the enhanced readability and efficiency of dplyr make it a compelling choice, especially in data analysis workflows where clarity and reproducibility are important.
Summary
In this guide, we learned different methods to remove specific row in R using both base functions and the dplyr package. Starting with base R, we covered different examples such as removing rows by index, eliminating those with missing values in specific columns, and filtering out rows with any missing values. Transitioning to dplyr, we used it to achieve the same outcomes with greater readability. We learned to use functions like slice()
, filter()
, and drop_na()
, making our code more intuitive and concise. Whether you prefer the simplicity of base R or the elegance of the tidyverse, this post equips you with versatile techniques to address various data-cleaning challenges.
Your feedback is invaluable! Please share this post on social media to help others, and do not hesitate to comment with corrections, suggestions, or requests for future topics. Engaging with the community enhances the learning experience for everyone!