In this very short tutorial, you will learn by example how to use the operator $ in R. First, we will learn what the $ operator does by getting the answer to some frequently asked questions. Second, we will work with a list that we create, and use the dollar sign operator to select and add a variable. Here you will also learn about the downsides of using $ in R and the alternatives you can use. In the following section, we will also work with a dataframe. Both sections will involve creating the list and the dataframe.
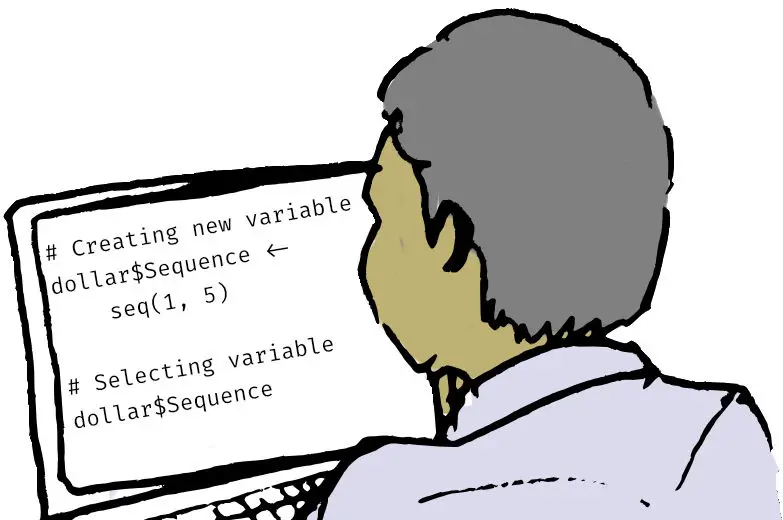
Table of Contents
Requirements
To follow this post, you need a working installation of the R statistical environment, of course. If you want to read the example Excel file, you will need the readxl package.
The $ operator can be used to select a variable/column, assign new values to a variable/column, or add a new variable/column in an R object. This R operator can be used on e.g., lists, and dataframes. For example, if we want to print the values in the column “A” in the dataframe called “dollar,” we can use the following code: print(dollar$A)
,
First, using the double brackets enables us to select multiple columns, e.g., whereas the $ operator only enables us to select one column.
Generally, you will use the $ operator to extract or subset a specific part of, e.g., a dataframe. For example, you can use the $ in R to extract a particular column. However, you can also use it to access elements in a list.
Before we go on to the next section, we will create a list using the list() function.
dollar <- list(A = rep('A', 5), B = rep('B', 5),
'Life Expectancy' = c(10, 9, 8, 10, 2))
Code language: R (r)
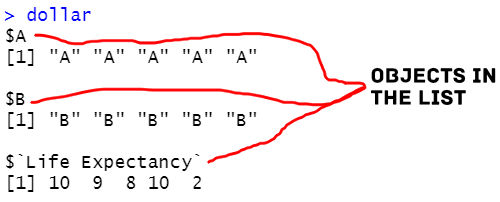
In the next section, we will, then, work with the $ operator to 1) add a new variable to the list, and 2) print a variable in the list. In the third example, we will learn how to use $ in R to select a variable which variable contains whitespaces.
Using $ in R on a List
Here we will start learning, by example, how to work with the $ operator in R. First, however, we will create a list.
Example 1: Using the $ Operator to Add a New Variable to a List
Here is how to use $ in R to add a new variable to a list:
dollar$Sequence <- seq(1, 5)
Code language: R (r)
Notice how we used the name of the list, then the $ operator, and the assignment (“<-”) operator. On the left side of <- we used seq() function to generate a sequence of numbers in R. This sequence of numbers was added to the list. Here is our example list with the new variable:
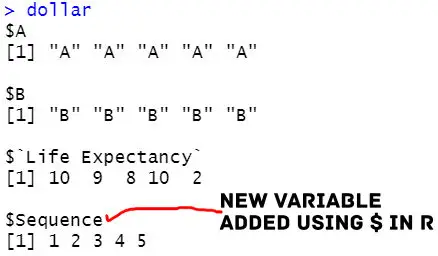
In the next example, we will use the $ operator to print the values of the new variable we added.
Example 2: How to use the $ Operator to Select a Variable in a List
Here is how we can use $ in R to select a variable in a list:
dollar$Sequence
Code language: R (r)
Again, we used the list name and the $ operator to print the new column we previously added:
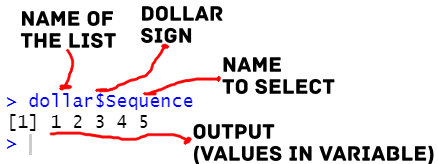
Note that if you want to select two or more columns, you have to use the double brackets and put in each column name as a character. Another option to select columns is, of course, using the select()
function from the excellent package dplyr.
Example 3: How to Select an Object containing White Spaces using $ in R
Here is how we can print or select a variable with whitespace in the name:
dollar$`Life Expectancy`
Code language: R (r)
Notice how we used the ` in the code above. This way, we can select or add values even though the variable contains white space. However, I suggest you rename the column (or replace the white spaces). See the recent post to learn how to rename columns in R. Again, using brackets, in this case, would be the same as when the variable is not containing white spaces.
The following section will use the examples above but on a dataframe. First, however, we will read an .xlsx file in R using the readxl package.
dataf <- read_excel('example_sheets.xlsx',
skip=2)
Code language: R (r)
Note that we used the skip argument to skip the first two rows. In the example data (download here), the column names are on the third row. We can print the first five rows of the dataframe using the head()
function:
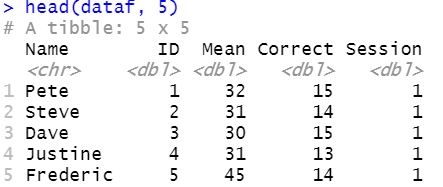
Here we can see that there are five columns. The next section will use the $ operator on this dataframe.
How to use $ in R on a Dataframe
In the first example, we will add a new column to the dataframe. After this, we will select the new column and print it using the $ operator. Finally, we will also add a new example of how to use this operator: to remove a column.
Example 4: Using $ to Add a new Column to a Dataframe
Here is how we can use $ to add a new column in R:
dataf$NewData <- rep('A', length(dataf$ID))
Code language: R (r)
Notice how we used R’s rep() function to generate a vector containing the letter ‘A’. We must generate a vector of the same length as the number of rows in our dataframe. Therefore, we used the length() function as the second argument.
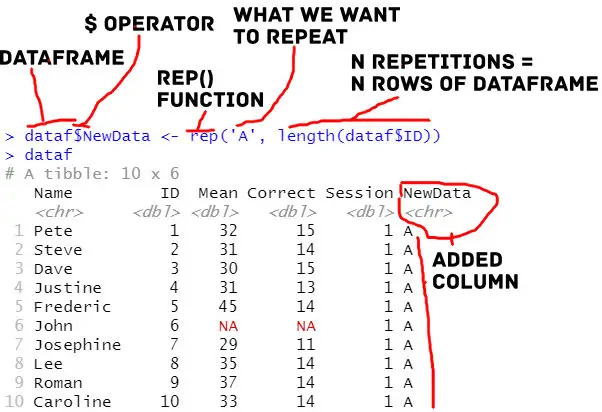
Now, if you want to learn easier ways to add a column in R, check the following posts:
- How to Add a Column to a Dataframe in R with tibble & dplyr
- R: Add a Column to Dataframe Based on Other Columns with dplyr
- How to Add an Empty Column to a Dataframe in R (with tibble)
In the next example, we will select this column using the $ operator and print it.
Example 5: Using $ to Select and Print a Column
Here is how we select and print the values in the column we created:
dataf$NewData
Code language: R (r)
Notice to select and print the values of a column in a dataframe we used R’s $ operator the same way we used it when we worked with a list. Here is the output of the code above:
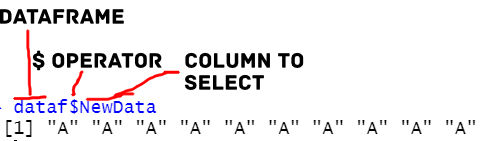
Now, it is easier to use the R package dplyr to select certain columns in R compared to using the $ operator. Another option is, of course, to use the double brackets.
In the following example, we will drop a column from the dataframe.
Example 6: Using $ in R together with NULL to delete a column
Here is how we can delete a column using the $ operator and the NULL object:
dataf$NewData <- NULL
Code language: PHP (php)
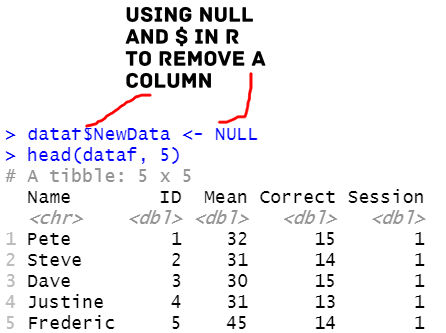
Again, we can use the R package dplyr to remove columns and remove a row in R. More specifically, we can use the select() function to delete multiple columns quickly and easily.
Note, that example 3 will also work if we have a column containing white spaces in our dataframe. Finally, before concluding this post, we will have a quick look at how to use brackets to select a column:
dataf['ID']
Code language: R (r)
Notice how we used the column name of the variable we wanted to select. This, again, will work on a list as well.
Example 7: Using $ together with the %in% operator in R
Here is how we can use $ together with %in% in R to subset our data:
df <- data.frame(names = c("Alice", "Bob", "Charlie", "Dave"),
reaction_time = c(0.2, 0.5, 0.3, 0.1),
age = c(25, 35, 30, 45),
city = c("New York", "Los Angeles", "Chicago", "Houston"))
# use the $ operator to extract the reaction_time column for people in New York and Los Angeles
ny_la_rt <- df$reaction_time[df$city %in% c("New York", "Los Angeles")]
Code language: PHP (php)
In the code chunk above, we use the $ operator to extract specific columns from the data frame. Specifically, df$reaction_time extracts the reaction_time column from df. We also use the %in% operator to extract rows that meet certain conditions. Here the df$city %in% c(“New York”, “Los Angeles”) returns a logical vector indicating which rows of df have a city value of either “New York” or “Los Angeles”. Using the $ operator, we use this logical vector to extract the reaction times of people from those cities using the $ operator.
Conclusion:
In this post, you have learned, by example, how to use $ in R. First, we worked with a list to add a new variable and select a variable. Then, we used the same methods on a dataframe. As a bonus, we also looked at how to remove a column using the $ operator. I hope you learned something. If you did, please share the post in your work, on your social media accounts, or link to it in your blog posts. Please comment below if you have any comments or suggestions for the post.
More Resources
- Sum Across Columns in R – dplyr & base
- R Count the Number of Occurrences in a Column using dplyr
- How to Rename Column (or Columns) in R with dplyr
- How to Rename Factor Levels in R using levels() and dplyr
- How to Remove Duplicates in R – Rows and Columns (dplyr)