In this R tutorial, you will learn how to generate sequences of numbers in R. There are many reasons why we would want to generate sequences of numbers. For example, we may want to generate sequences when plotting the axes of figures or simulating data.
Table of Contents
- Outline
- Create a Sequence of Numbers in R using the : operator
- Generate a Sequence of Numbers in R with the seq() Function
- Generating Repeated Sequences of Numbers in R
- Conclusion
- Resources
Outline
Often, there is no one way to perform a specific task in R. In this post, we are going to use the :
operator, the seq()
, and rep()
functions. First, we start having a look at the : operator. Second, we dive into the seq() function including the arguments that we can use. Third, we will have a look at how we can use the rep() function to generate e.g. sequences of the same numbers or a few numbers.
Create a Sequence of Numbers in R using the : operator
The absolutely simplest way to create a sequence of numbers in R is by using the :
operator. Here’s how to create a sequence of numbers, from 1 to 10:
1:10
Code language: R (r)
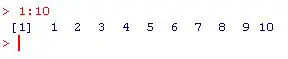
As you can see, in the image above, that gave us every integer from 1 and 10 (an integer is every positive or negative counting number, including 0). Furthermore, the created sequence is in ascending order (i.e., from the smallest number to the largest number). We will soon learn how to generate a sequence in descending order. First, however, if we want our sequence, from 1 to 10, to be saved as a variable we have to use the <-
and create a vector:
numbers <- 1:10
Code language: R (r)
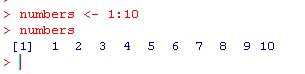
Descending Order
Now, you might already have guessed that we can change the order of the smallest and largest numbers to generate a sequence of numbers in descending order:
25:1
Code language: R (r)

Note, that if you want to know more about a particular R function, you can access its documentation with a question mark followed by the function name: ?function_name_here.
In the particular case, however, an operator like the colon we used above, you must enclose the symbol in backticks like this: ?’:’. Before we go to the next section it is worth mentioning that you can also use R to transpose a matrix or a dataframe.
Generate a Sequence of Numbers in R with the seq() Function
Often, we desire more control over a sequence we are creating than what the :
operator will give us. The seq()
function serves this purpose and is a generalization of the :
operator, which creates a sequence of numbers with a specified arithmetic progression.
Now, the most basic use of seq()
, however, works the same way as the :
operator does. For example, if you t
ype seq(1, 10)
this will become clear. That is, running this command will generate the same sequence as in the first example:
seq(1, 10)
Code language: R (r)

Evidently, we got the same output as using the :
operator. If we have a look at the documentation we can see that there are number of arguments that we can work with:
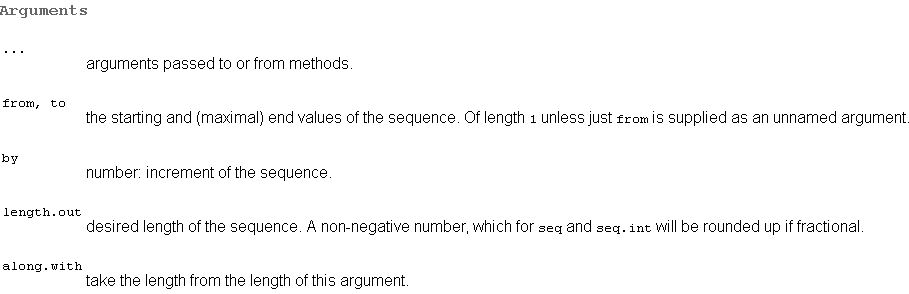
As you can see in the image above (or in the documentation): the first two arguments of seq()
are “from =” and “to =”. In R, we do not have to use the name of the arguments. That is, if we write out their values in the same order as written in the function it will produce the same results as using the names. It is worth noting, however, for more complex functions best practice is to use the names of the arguments. This will also make the code much clearer. For example, we can generate a sequence of descending numbers like this:
seq(from = 20, to = 1)
Code language: R (r)
In the next subsection, we will have a look at the “by=” argument that enables us to define the increment of the sequence.
Create a Sequence in R with a Specified Increment Step
In some cases, we may want, instead of 1 to 20, a vector of numbers ranging from 0 to 20, sequences incremented by e.g. 2. Here’s how to create a sequence of numbers with a specified increment step:
seq(0, 20, by = 2)
Code language: R (r)

As you can see, in the image below, this produces a vector with fewer numbers but every number is increased by 2. In the next section, we will have a look at how to specify how many numbers we want to generate between two specified numbers.
Generating a Specified Amount of Numbers between two Numbers
Here’s how we can use the length argument to generate 30 numbers between 1 and 30:
# sequence
nums <- seq(1, 30, length = 10)
Code language: R (r)
Now, this generated 30 floating-point numbers between 1 and 30. If we want to check whether there really are 30 numbers in our vector we can use the length()
function:
length(nums)
Code language: R (r)
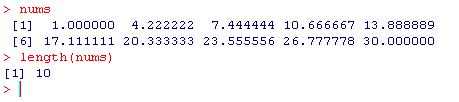
Now, as previously mentioned there are often many different approaches for solving the same problems. This is, of course, also for R statistical programming language. In general, choosing the simplest approach which includes as little code as possible is probably the way to go. That said, we will go to the next section where we will be learning to get a sequence of the same number (e.g, “0”). In a more recent post, you will learn 7 examples of when and how to use the %in% operator in R.
Generating Repeated Sequences of Numbers in R
To get a repeated sequence of a number we can use the rep() function. Here’s how to create a vector containing 10 repetitions of the number 0:
rep(0, 10)
Code language: R (r)
Now, the rep() function can also be used together with the :
operator, the c()
or the seq()
functions.
Repeated Sequences of Specified Numbers in R
In this example, we are going to get the numbers 1, 2, 3 generated 10 times. Here’s how to repeat a sequence of numbers.
# Repat a sequence of numbers:
rep(c(1, 2, 3), times=10)
Code language: R (r)

If we, on the other hand, want to replicate a sequence (e.g., 1 to 5) 10 times we can use the :
operator:
# Repeating a sequence of numbers ten times
rep(1:5, times=10)
Code language: R (r)
Finally, it is also possible to get each number, that we want in our sequence, to be generated a specified amount of times:
rep(1:5, each=10)
Code language: R (r)

Note, that if we want to repeat a function or generate e.g., sequences of numbers we can use the repeat and replicate functions in R.
If you want to generate non-random numbers, you can use the : operator. For instance, to generate numbers between 1 and 10 you type 1:10. Another option is to use the seq() function.
If you want to create a sequence vector containing numbers you use the : operator. For example, 1:15 will generate a vector with numbers between 1 and 15. To gain more control, you can use the seq() method.
To repeat a sequence of numbers in R, you can use the rep() function. For example, if you type rep(1:5, times=5) you will get a vector with the sequence 1 to 5 repeated 5 times.
The function rep() in R will repeat a number or a sequence of numbers n times. For example, typing rep(5, 5) will print the number 5 five times.
Check out the following posts if you need to extract elements from datetime in e.g. a vector:
- How to Extract Year from Date in R with Examples
- How to Extract Day from Datetime in R with Examples
- How to Extract Time from Datetime in R – with Examples
Conclusion
In this post, you have learned how to get a sequence of numbers using the : operator, the seq() and rep() functions. Specifically, you learned how to create numbers with a specified increment step. You have also learned how to repeat a number to get a sequence of the same numbers.
Resources
Here are a couple of R tutorials that you may find useful:
- Correlation in R: Coefficients, Visualizations, & Matrix Analysis
- Coefficient of Variation in R
- How to Create a Word Cloud in R
- ggplot Center Title: A Guide to Perfectly Aligned Titles in Your Plots
- How to Take Absolute Value in R – vector, matrix, & data frame
- Mastering SST & SSE in R: A Complete Guide for Analysts
- Wide to Long in R using the pivot_longer & melt functions
- Plot Prediction Interval in R using ggplot2
- Binning in R: Create Bins of Continuous Variables