How to check if a file is empty in R can be a valuable skill to know when reading data from multiple files. Imagine a scenario dealing with considerable data collected from numerous participants. In such cases, encountering empty files due to technical glitches or other reasons is not uncommon.
This guide ventures into R programming to learn how to do file validation. We will focus on determining whether a file is empty, a skill of value in refining your data-handling workflows. By learning to identify and skip empty files, you streamline your data processing efforts, ensuring that only meaningful data undergoes analysis.
Through practical examples, we will explore various techniques for checking empty files in R. From individual files to entire folders, you will gain insights into strategies that suit different scenarios.
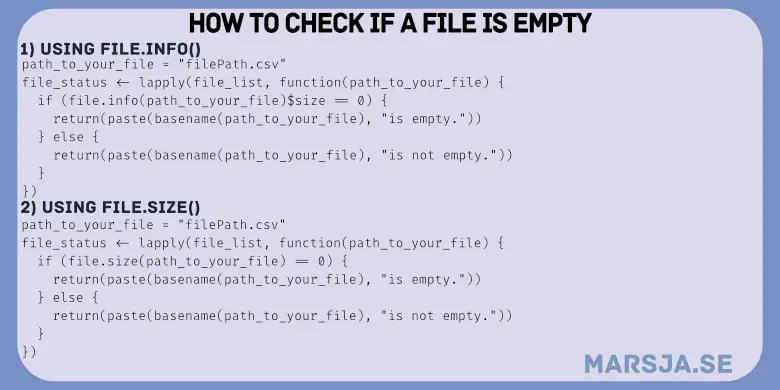
Table of Contents
- Outline
- Prerequisites
- How to Check if a File is Empty
- Example 1: Checking Whether a File is Empty using R’s file.info() Function
- Example 2: How to Check if a File is Empty in R with the file.size() Function
- Example 3: Checking Whether Multiple Files Are Empty in R
- Example 4: Only Import Non-Empty Files in R
- Conclusion: How to Check if a File is Empty in R
- Resources
Outline
The outline of the post is as follows: We will dive into the essential topic of checking file emptiness in R, unveiling powerful techniques for data validation. First, we will introduce the file.info()
function, demonstrating its capacity to determine if a file is empty and extract file attributes. This foundational knowledge will pave the way for exploring the more specialized file.size()
function in Example 2.
Moving forward, we will also look at a practical application of these techniques in Example 3. By showcasing how to assess whether multiple files are empty within a directory, we address real-world scenarios where efficient file validation is crucial, especially when dealing with extensive datasets or diverse file types.
Example 4 will underscore the significance of our learnings by showcasing a tangible use case. We will explore how to import non-empty files exclusively, illustrating the immediate impact of checking whether a file is empty in R.
Prerequisites
To follow this post, the reader should possess a fundamental understanding of R programming and its fundamental functions. For those interested in utilizing dplyr to merge data frames, ensure the installation of the package by employing the install.packages()
function. Notably, dplyr offers a suite of powerful data manipulation tools that extend beyond merging, including the ability to rename factor levels, rename columns, select specific columns, and remove columns—a versatile set of capabilities at your disposal.
Before delving into the content, checking your R version is essential. Ensuring you have the latest version guarantees access to the most up-to-date features and optimizations. Should your version be outdated, consider updating R to benefit from the latest enhancements and improvements.
How to Check if a File is Empty
We can use the file.info()
and file.size()
functions to check if a file is empty in R. Let us look at the syntax and functionality of these functions:
1. file.info()
We can use the file.info()
function to retrieve file information, including details like size, permissions, modification time, and more.
file_info <- file.info(file_path)
Code language: R (r)
In the code chunk above, we can see the general syntax of the file.info()
function. Here is an example output running the function on a Word file containing a correlation table formatted according to APA 7:

2. file.size()
On the other hand, if we only want to know the file size, we can use the file.size()
. This function returns the size of a file in byte ru
file_size <- file.size(file_path)
Code language: R (r)
In the code snippet above, we can see the general use of the file.size()
function. The following sections will cover practical examples of using the functions to check whether a file is empty. However, we will focus on the latter function.
Example 1: Checking Whether a File is Empty using R’s file.info() Function
Here is an example of how to check whether a file is empty using the file.info()
function:
file_info <- file.info("data5.txt")
# Check if the file is empty
if (file_info$size == 0) {
cat("The file is empty.\n")
} else {
cat("The file is not empty.\n")
}
Code language: R (r)
In the code chunk above, we use the file.info()
function to retrieve information about the file “data5.txt.” We then employ an if statement to determine if the file is empty. If the size information from file_info equals zero (0), it indicates an empty file, and a message confirming its emptiness is displayed. Otherwise, if the size information exceeds 0, the code ensures the file is not empty. To use this code, provide the correct file path and name.
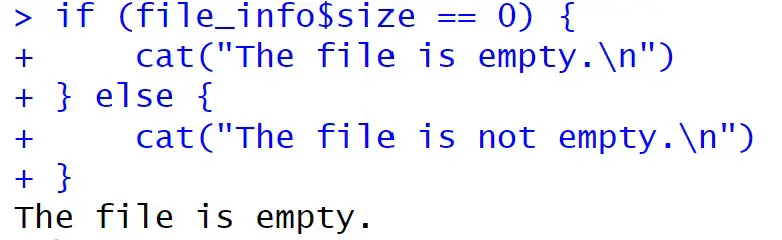
Example 2: How to Check if a File is Empty in R with the file.size() Function
To check whether a file is empty, we can also use the file.size()
function:
# Check if the file is empty
if (is.na(file.size("data5.txt"))) {
cat("The file is empty.\n")
} else {
cat("The file is not empty.\n")
}
Code language: R (r)
In the code snippet above, we check whether the file “data5.txt” is empty. Again, we use an if statement and the check whether the file size is zero (0). If the condition is met, indicating an empty file, a message indicates that the file is indeed empty. Conversely, if the file size information is greater than zero, the code affirms that the file is not empty.
It is important to note that this approach is not limited to just text files like “data5.txt.” It also extends its utility to other file formats, such as .csv and .json files.
Example 3: Checking Whether Multiple Files Are Empty in R
We can modify our code to determine whether files in a specified folder are empty using the R function file.size()
:
# Specify the directory path
dir_path <- "./text_files/"
# List files in the directory
file_list <- list.files(path = dir_path, full.names = TRUE)
# Check if each file is empty using lapply
file_status <- lapply(file_list, function(file_path) {
if (file.size(file_path) == 0) {
return(paste(basename(file_path), "is empty."))
} else {
return(paste(basename(file_path), "is not empty."))
}
})
# Print the results
cat(paste(file_status, collapse = "\n"), "\n")
Code language: R (r)
In the code chunk above, we extend the previous examples to efficiently check the emptiness of multiple files within a specified directory.
We begin by setting the dir_path variable to the directory path containing the files we want to check, denoted as “./text_files/”. The list.files()
function compiles a list of files within this directory while full.names = TRUE
ensures that we obtain the full paths of these files.
Using the lapply()
function, we iterate through each file in file_list
. For each file, we execute an anonymous function. Within this function, we employ the file.size()
function to ascertain the file size. If the file’s size equals 0, the if condition evaluates as TRUE
, indicating that the file is empty. In such cases, we return a message stating that the file is empty, accompanied by its basename.
Conversely, if the file’s size is not 0, the else block is executed, and a message declaring that the file is not empty is returned.
Finally, the paste()
function combines the file status messages generated by the lapply()
loop. By applying collapse = "\n"
, we format the messages to display on separate lines. The cat()
function prints the consolidated results, indicating whether each directory file is empty. In the following section, we will look at a more practical example.
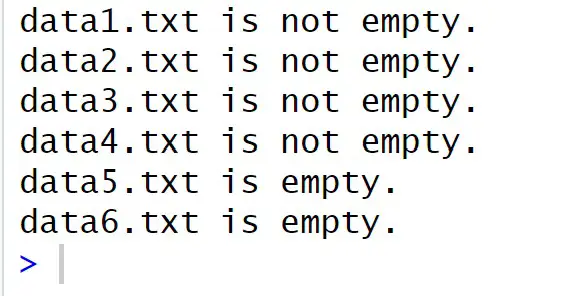
Example 4: Only Import Non-Empty Files in R
Here is a more practical example of checking whether files are empty in R. We can modify the previous code only to read the files that are not empty.
# Specify the directory path
dir_path <- "./csv_files/"
# List CSV files in the directory
csv_files <- list.files(path = dir_path,
pattern = "\\.csv$", full.names = TRUE)
# Function to read non-empty CSV files
read_non_empty_csv <- function(file_path) {
if (file.size(file_path) == 0) {
cat(paste("Skipping empty file:", basename(file_path), "\n"))
return(NULL)
} else {
cat(paste("Reading data from:", basename(file_path), "\n"))
return(read.csv(file_path))
}
}
# Read non-empty CSV files using lapply
data_list <- lapply(csv_files, read_non_empty_csv)
# Filter out NULL entries (empty files)
data_list <- data_list[!sapply(data_list, is.null)]
# Print the results
cat(paste(file_status, collapse = "\n"), "\n")
Code language: R (r)
The code chunk above focuses on reading and processing non-empty CSV files from a specified directory.
We start by defining the dir_path variable, indicating the directory path where the CSV files are located. The list.files()
function then compiles a list of CSV files within this directory, using the pattern argument with the regular expression "\.csv$"
to filter out only CSV files. Again, we use the full.names = TRUE
option to ensure we obtain these files’ full paths.
We define the read_non_empty_csv()
function to handle the reading process efficiently. This function takes a file path as an argument and checks whether the file is empty using the file.size()
function. A message is displayed if the file is empty and NULL
is returned. Otherwise, the function reads the data from the CSV file using read.csv()
and displays a message indicating the file being read.
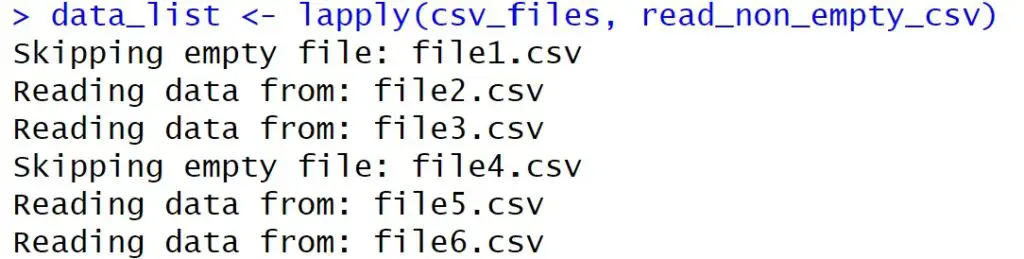
Using lapply()
, we apply the read_non_empty_csv()
function to each CSV file in the list. This results in a list of data frames named data_list
containing data from non-empty CSV files.
In the subsequent step, we filter out NULL
entries from the data_list
using the sapply()
function and logical indexing. This effectively removes dataframes corresponding to empty files. We can use, e.g., dplyr
and bind_rows()
to merge the dataframes to one dataframe:
# Merge dataframes from the list using dplyr
merged_data <- bind_rows(data_list)
Code language: R (r)
Here is the structure of the resulting dataframe:

Conclusion: How to Check if a File is Empty in R
n this post, we have explored various techniques to address a fundamental data handling concern: checking if a file is empty in R. By utilizing R’s built-in functions, file.info()
and file.size()
, we have provided an overview of how to verify the emptiness of files in different scenarios effectively.
In Example 1, we used R’s file.info()
function, demonstrating how it can aid in detecting empty files and extracting essential file information. In Example 2, we expanded our repertoire by highlighting the efficiency of the file.size() function. This function offers a streamlined approach designed to detect file size.
In Example 3, we explored the practical application of these techniques by illustrating how to assess the emptiness of multiple files within a directory efficiently. This real-world scenario highlights the importance of determining file emptiness when working with large datasets or diverse file types.
Example 4 further solidified the significance of this skill by showcasing a common use case. We demonstrated how to read and import only non-empty files, showcasing the direct impact of detecting whether a file is empty on real data manipulation tasks.
Please share this post with fellow data enthusiasts. Moreover, I welcome your thoughts and suggestions in the comments section below. Your feedback fuels my commitment to providing valuable insights and practical solutions for your data-related challenges.
Resources
Here are plenty more tutorials for your needs:
- How to Create Dummy Variables in R (with Examples)
- Durbin-Watson Test in R: Step-by-Step incl. Interpretation
- How to Take Absolute Value in R – vector, matrix, & data frame
- Correlation in R: Coefficients, Visualizations, & Matrix Analysis
- How to Calculate Z Score in R
- ggplot Center Title: A Guide to Perfectly Aligned Titles in Your Plots
- Binning in R: Create Bins of Continuous Variables
Thanks for the post! Just a minor comment: file.size() is vectorized, so example 3 could be written as
paste(file_list, ‘is’, ifelse(file.size(file_list) == 0, ”, ‘not’), ’empty’, collapse = ‘\n’)
Thanks for your comment, Yihui. I will add that to Example 3.