In this comprehensive tutorial, we will look at the capabilities of one operator that is very handy for data wrangling: or in R. We will learn how useful and powerful the or operator, symbolized by “|”, can be and explore how it can be used in our data analysis workflows. Whether you are a seasoned data scientist or a beginner getting into the world of R programming, understanding and harnessing the full potential of the or operator will empower you to manipulate, analyze, and visualize data with flexibility and precision.
Example of when to use an or (|) operator
Imagine you are investigating the interplay between cognition and hearing. As you explore the dataset, you may encounter scenarios where you must extract specific observations satisfying multiple conditions. This is precisely where the or operator becomes an invaluable tool. Using the R’s or operator, you can combine logical conditions to filter your data to obtain subsets that meet your desired criteria.
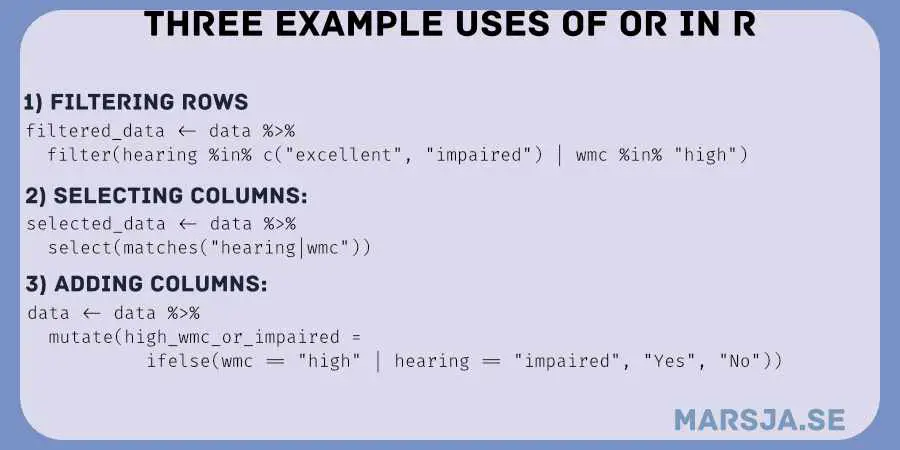
Let us consider a practical example. Suppose you want to analyze the relationship between cognition and hearing in individuals above 60 years of age or with a hearing impairment. Here, we can use the or operator to filter the dataset effortlessly, for example, by including only those participants who fulfill either of these conditions. This focused subset will serve as the foundation for further analysis. It can enable you to gain insights specific to your research questions.
Throughout this tutorial, we will explore different applications of the or operator in R. You will learn how to construct complex logical expressions, perform efficient data filtering, and unlock the true potential of your datasets. By the end of this tutorial, you will have a solid understanding of utilizing the or operator effectively, empowering you to handle diverse data-wrangling challenges confidently.
Table of Contents
- Example of when to use an or (|) operator
- Outline
- Requirements
- Synthetic Data
- 1. Filtering based on multiple conditions using the or and %in% operators in R:
- 2. Selecting columns that match specific patterns using matches() and or in R:
- 3. Selecting columns that contain specific substrings using or and contains():
- 4. Adding a new column based on the values in another column using or and mutate():
- 5. Filtering based on multiple logical conditions using or and comparison operators:
- 6. Conditional recoding of a variable using case_when() and or in R:
- 7. Combining logical conditions with or in R in an if statement:
- 8. Summarizing data based on multiple conditions using or and group_by() with summarize():
- Conclusion
- Resources
Outline
This blog post will first outline the requirements to follow along effectively. You must install R and an interactive development environment (IDE) like RStudio. Basic knowledge of R programming is also recommended.
Next, We will dive into synthetic data generation to create a dataset for practicing the or operator in R. This dataset will involve variables related to hearing and working memory capacity.
We will then explore various examples of utilizing the or operator in R. We will cover filtering based on multiple conditions using the or operator in conjunction with the ‘%in%’ operator. Additionally, we will demonstrate selecting columns that match specific patterns using the ‘matches()’ function and or in R.
Next, We will discuss selecting columns that contain specific substrings using contains()
and the or operator. We will also showcase adding a new column based on values in another column using the or operator and mutate()
.
Furthermore, We will demonstrate how to filter based on multiple logical conditions using the or operator and comparison operators. Conditional recoding of a variable using case_when
() and or in R will also be covered.
Additionally, we will explore combining logical conditions with or in R within if statements and summarizing data based on multiple conditions using or and group_by()
with summarize()
functions.
Throughout this blog post, We will provide detailed explanations and code examples to ensure a clear understanding of each concept. So, let us get started and learn the full potential of the or operator in R for efficient data manipulation and analysis.
Requirements
To effectively follow this blog post, ensure that you have R installed on your system, as it will serve as the programming language for implementing the concepts discussed. Make sure you have a version of R that is up-to-date. Additionally, it is recommended to utilize an interactive development environment (IDE) such as RStudio, Jupyter Notebook with R kernel, or Visual Studio Code with R extensions. These IDEs provide a user-friendly interface with syntax highlighting and code completion features, enabling a seamless coding experience.
While prior programming experience is not mandatory, having a basic understanding of R programming will greatly facilitate your comprehension. Familiarity with concepts such as variables, functions, conditional statements, and data structures in R is beneficial and will aid in following the examples provided.
By meeting these requirements, you will be well-prepared to learn from this tutorial on mastering the or operator in R for efficient data wrangling. Embrace this opportunity to enhance your data manipulation skills and gain valuable insights from the power of the ‘or’ operator in conjunction with dplyr functions.
Synthetic Data
Here, we generate a synthetic dataset specifically designed for practicing the usage of the or in R. This dataset will serve as a valuable resource to enhance your skills in working with logical conditions.
# Loading required libraries
library(dplyr)
# Generating the dataset
hearing <- c("excellent", "impaired", "normal")
wmc <- c("low", "medium", "high")
# Creating combinations of hearing and working memory capacity (WMC)
data <- expand.grid(hearing = hearing, wmc = wmc)
# Generating the dependent variable SNR
data <- data %>%
mutate(snr = ifelse(hearing == "impaired", -6.1, -9.1))
Code language: R (r)
In the code chunk above, we start by loading the necessary library, dplyr
, which provides powerful functions for data manipulation in R. Next, we generate the dataset by defining the levels for the variables hearing
and wmc
. The hearing
variable includes categories for “excellent,” “impaired,” and “normal,” while wmc
consists of “low,” “medium,” and “high.”
To create a comprehensive dataset, we utilize the expand.grid()
function. This function generates all possible combinations of the specified variables, resulting in a dataset with the combinations of hearing
and wmc
.
Moving forward, we introduce the dependent variable, SNR (Signal-to-Noise Ratio), to the dataset using the mutate()
function. With the help of the ifelse()
function, we assign values to the snr
variable based on a conditional statement. If the variable’s value is “impaired,” the corresponding SNR value is set to -6.1. Otherwise, for “excellent” and “normal” hearing levels, the SNR is assigned -9.1. By using the %>%
pipe operator, we update the dataset data
with the newly created snr
variable.
Here are eight examples of using the or operator in R with the provided dataset:
1. Filtering based on multiple conditions using the or and %in%
operators in R:
Here is how we can use or in R together with the R’s %in% operator:
filtered_data <- data %>%
filter(hearing %in% c("excellent", "impaired") | wmc %in% "high")
Code language: R (r)
In the code chunk above, we used the or operator in R in conjunction with the filter()
function to create the filtered_data
dataset. This code allows us to filter rows based on specific conditions selectively.
Using the pipe operator %>%
, we pass the data
dataset to the filter()
function. Within the filter()
function, we specify the filtering conditions using the or operator |
.
The first condition, hearing %in% c("excellent", "impaired")
, checks if the value of the hearing
variable is either “excellent” or “impaired”. The %in%
operator checks for membership in a vector, and here it determines if the value of hearing
matches any of the specified levels.
The second condition, wmc %in% "high"
, checks if the value of the wmc
variable is “high”. Similarly, the %in%
operator checks for a match between wmc
and the specified level.
By using the or operator |
between these conditions, we instruct R to include rows in the filtered_data
dataset that satisfy either of the conditions. In other words, if the value of hearing
is “excellent” or “impaired”, or if the value of wmc
is “high”, the row will be included in the filtered_data
.
2. Selecting columns that match specific patterns using matches()
and or in R:
Here is another example where we use the select()
and matches()
function together with or in R:
selected_data <- data %>%
select(matches("hearing|wmc"))
Code language: HTML, XML (xml)
In the code chunk above, we selected specific columns from the data
dataset using the select()
function in R.
Within the select()
function, we used the matches()
function along with the pattern “hearing|wmc”. This pattern specifies a regular expression that matches column names “hearing” or “wmc”.
Using the pipe operator %>%
, we pass the data
dataset to the select()
function for further processing.
As a result, the selected_data
dataset is created, consisting of only the columns that match the specified pattern. Any column names that include “hearing” or “wmc” in their names will be included in the selected_data
dataset, while other columns will be excluded.
This code allows for selecting specific columns based on patterns in their names, providing flexibility in working with datasets that contain a large number of columns.
3. Selecting columns that contain specific substrings using or and contains()
:
Here is a third example where we use or in R to select columns:
selected_data <- data %>% select(contains("hear") | contains("wmc"))
Code language: JavaScript (javascript)
In the code chunk above, we utilized the select()
function in R to choose specific columns from the data
dataset. Building upon the previous example (Example 2), we employed the contains()
function within the select()
function to identify columns based on specific substrings.
By using the pipe operator %>%
, we passed the data
dataset to the select()
function, similar to Example 2.
Within the select()
function, we incorporated the contains()
function. This function searches for columns that contain either the substring “hear” or “wmc” in their column names.
4. Adding a new column based on the values in another column using or and mutate()
:
Here we add a column to the dataframe based on other columns using mutate()
and or in R:
data <- data %>%
mutate(high_wmc_or_impaired =
ifelse(wmc == "high" | hearing == "impaired", "Yes", "No"))
Code language: JavaScript (javascript)
In the code chunk above, we employed the mutate()
function in R to add a new column to the data
dataset. The new column is named high_wmc_or_impaired
, and we used the ifelse()
function to determine its values based on specific conditions.
Using the pipe operator %>%
, we passed the data
dataset to the mutate()
function for further transformation.
Within the mutate()
function, we utilized the ifelse()
function to assign values to the high_wmc_or_impaired
column. The condition wmc == "high" | hearing == "impaired"
evaluates whether the value of the wmc
column is “high” or the value of the hearing
column is “impaired”.
If the condition is met, the corresponding value in the high_wmc_or_impaired
column is set to “Yes”. Otherwise, if the condition is not satisfied, the value is set to “No”.
By incorporating the or operator |
within the condition of the ifelse()
function, we instruct R to evaluate both conditions and assign the appropriate value to each row in the high_wmc_or_impaired
column.
5. Filtering based on multiple logical conditions using or and comparison operators:
Here we subset data in R using the or operator and the filter()
function:
filtered_data <- data %>% filter(wmc == "medium" | snr < -7)
Code language: HTML, XML (xml)
In the code chunk above, we utilized the filter()
function in R to create the filtered_data
dataset. This code allows us to filter rows based on specific conditions selectively.
Using the pipe operator %>%
, we passed the data
dataset to the filter()
function for further processing.
Within the filter()
function, we specified the filtering conditions using the or operator |
. The first condition, wmc == "medium"
, checks if the value of the wmc
column is equal to “medium”. The second condition, snr < -7
, checks if the value of the snr
column is less than -7.
By using the or operator |
between these conditions, we instruct R to include rows in the filtered_data
dataset that satisfy either of the conditions. In other words, if the value of wmc
is “medium” or the value of snr
is less than -7, the row will be included in the filtered_data
.
6. Conditional recoding of a variable using case_when()
and or in R:
Here we recode a variable using the case_when()
function and the or operator in R:
data <- data %>%
mutate(hearing_group =
case_when(hearing == "excellent" | hearing == "impaired" ~ "Good",
TRUE ~ "Normal"))
Code language: PHP (php)
In the code chunk above, we used the mutate()
function in R to add a new column called hearing_group
to the data
dataset. We employed the case_when()
function to assign values to the new column based on specific conditions.
Using the pipe operator %>%
, we passed the data
dataset to the mutate()
function for further transformation.
Within the mutate()
function, we utilized the case_when()
function to evaluate different conditions. The first condition, hearing == "excellent" | hearing == "impaired"
, checks if the value of the hearing
column is either “excellent” or “impaired”.
If the condition is met, the corresponding value in the hearing_group
column is set to “Good”. Otherwise, if the condition is not satisfied, the value is set to “Normal”.
By using the or operator |
within the condition of the case_when()
function, we evaluate multiple conditions and assign the appropriate value to each row in the hearing_group
column.
7. Combining logical conditions with or in R in an if statement:
Here is another example of using the or operator in R:
for (i in 1:nrow(data)) {
if (data$hearing[i] == "impaired" | data$wmc[i] == "high") {
print(paste("Participant", i, "meets the criteria")) } }
Code language: PHP (php)
In the code chunk above, we used a for loop to iterate over each row in the data
dataset and performed a conditional check on the values of the hearing
and wmc
columns.
The loop starts with the for
statement, where we define a loop variable i
that iterates from 1 to the total number of rows in the data
dataset, specified by nrow(data)
.
Within the loop, we used an if
statement to check if the value of the hearing
column at the current iteration (data$hearing[i]
) is equal to “impaired” or if the value of the wmc
column at the current iteration (data$wmc[i]
) is equal to “high”.
If the condition is true, meaning either the hearing
is “impaired” or the wmc
is “high”, we execute the code block inside the curly braces. In this case, we print a message using the print()
function and the paste()
function to concatenate the strings “Participant”, the current iteration value i
, and “meets the criteria”.
By using the paste()
function, we create a formatted string that displays the participant number (i
) and indicates that they meet the specified criteria.
8. Summarizing data based on multiple conditions using or and group_by()
with summarize()
:
Here we calculate descriptive statistics using the group_by()
and summarize()
functions together with or in R:
summary_data <- data %>%
group_by(wmc %in% c("medium", "high") | snr < -8) %>%
summarize(mean_snr = mean(snr))
Code language: HTML, XML (xml)
In the code chunk above, we performed data summarization using the group_by()
and summarize()
functions in R.
Using the pipe operator %>%
, we passed the data
dataset to the group_by()
function for grouping the data based on specific conditions. Within the group_by()
function, we used the wmc %in% c("medium", "high") | snr < -8
condition. This condition checks if either the wmc
column value is “medium” or “high”, or if the snr
column value is less than -8. It groups the data accordingly. Next, we used the %>%
operator again to pass the grouped data to the summarize()
function for calculating the mean of the snr
column.
Within the summarize()
function, we specified mean_snr = mean(snr)
to compute the mean of the snr
column for each group. As a result, the summary_data
dataset is created, containing the mean snr
value for groups based on the specified conditions. These examples demonstrate various ways the or operator can filter, select, mutate, and summarize data in R. This way showcases its versatility and power in data manipulation tasks.
Conclusion
In this post, you have learned about the powerful applications of the or operator in R. You learned how it can greatly enhance your data manipulation and analysis workflows. By mastering the use of or in combination with various functions and operators, you can efficiently filter, select, mutate, and summarize your data based on multiple conditions.
Throughout the post, we explored different examples and techniques that showcased the versatility of or in R. You gained insights into filtering data based on multiple conditions using the or operator with %in%
, matching specific patterns with matches()
, and selecting columns containing specific substrings using contains()
.
Additionally, you learned how to add new columns based on values in other columns using the or operator with mutate()
, and perform conditional recoding using case_when()
. We also discussed how to combine logical conditions with or in R’s if statements and how to summarize data based on multiple conditions using group_by()
and summarize()
.
Now that you have acquired these valuable skills apply them to your data analysis tasks. Share this post with your colleagues and friends who might benefit from learning about the versatile or operator in R. Together, we can expand our knowledge and use the full potential of R in data manipulation and analysis.
Resources
Here are some resources that you might find helpful:
- Countif function in R with Base and dplyr
- Plot Prediction Interval in R using ggplot2
- How to Create a Sankey Plot in R: 4 Methods
- Sum Across Columns in R – dplyr & base
- How to Convert a List to a Dataframe in R – dplyr
- R Count the Number of Occurrences in a Column using dplyr
- How to Rename Column (or Columns) in R with dplyr