In this tutorial, you will learn how to randomly select rows in R, an essential skill when working with large datasets or conducting statistical analyses. The ability to randomly sample rows enables us to extract representative subgroups or explore data in a randomized order. For instance, if you are building a random forest regression model and need to create a training and test dataset. Randomly selecting rows can ensure that both datasets have a diverse data representation, avoiding biases and producing robust results.
To achieve this task in R, we will utilize the power of both base R and the tidyverse packages. These packages provide efficient and flexible functions for random sampling from a dataframe.
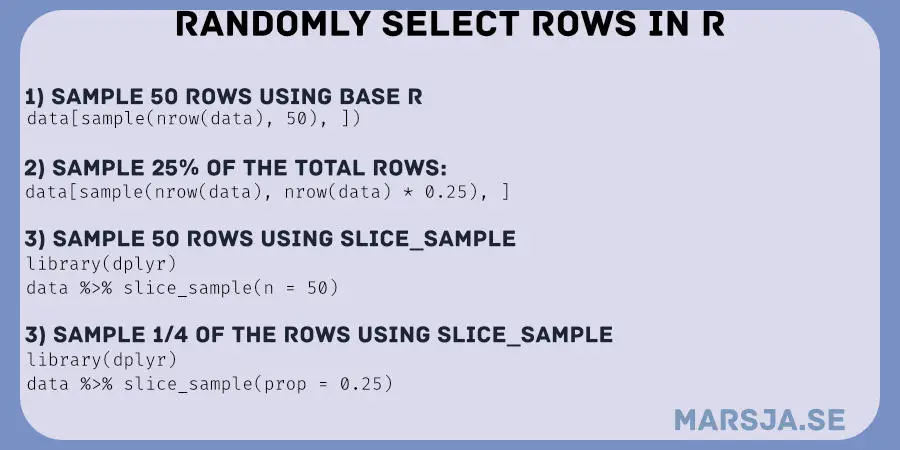
Now, let us dive into the tutorial and explore the step-by-step process of randomly sampling rows in R. We will begin by examining the built-in functions and techniques available in base R. Next, we will explore the rich functionality provided by the tidyverse packages, such as dplyr and tidyr, which offer elegant and intuitive methods for data manipulation and sampling.
We follow clear examples and explanations throughout the tutorial to ensure your understanding. So, let us get started and unlock the power of random sampling in R!
Table of Contents
- Outline
- Functions in R to Randomly Select Rows
- Synthetic Data
- How to Randomly Select Rows in R using the sample() Function
- How to Randomly Select Rows in R using slice_sample()
- Conclusion
- Resources
Outline
In the first section, we will have a look at the widely used sample()
function. This function allows us to randomly select rows by specifying the desired number or proportion of rows to be sampled. Next, we will have a look the slice_sample()
function from the dplyr package. This function also provides a convenient way to randomly select rows by specifying the number or the proportion of rows to be sampled. To demonstrate the usage of these functions, we will then generate a synthetic dataset that simulates data related to hearing and perception in a psychology study. In the following sections, we will present step-by-step examples of how to randomly select a specific number of rows or a proportion of rows from the dataset using both the sample()
function and the slice_sample()
function.
Functions in R to Randomly Select Rows
To take a random sample from a dataframe in R, we have a range of powerful functions and packages at our disposal. We can use functions of base R and the popular tidyverse packages to accomplish this task.
The function sample()
is our go-to random sampling option in base R. This versatile function allows us to extract a specified number of random rows from a dataframe. By specifying the desired sample size, we can ensure that our subset represents the original data.
Alternatively, if you prefer a more expressive and intuitive syntax, the tidyverse packages, such as dplyr and tidyr, provide convenient functions for sampling. With dplyr, we can use the slice_sample() function to randomly select rows from a dataframe based on a given fraction or number. This allows for easy sampling while preserving the overall structure of the dataset.
Furthermore, if you require more advanced sampling techniques like stratified sampling, the dplyr package combines the group_by()
and sample_n()
functions. These functions enable us to stratify our dataframe based on specific variables and obtain random samples from each stratum.
In addition to its capabilities for random sampling, the dplyr package offers a wide array of functions that simplify various data manipulation tasks. With dplyr, we can perform operations such as selecting columns, removing columns, renaming columns, and adding new columns to a dataframe, all concisely and intuitively.
By combining the power of base R and the tidyverse packages, we can confidently tackle any sampling requirement in our data analysis. In the upcoming sections of this tutorial, we willlook at each method in detail, providing practical examples and step-by-step instructions.
Sample()
R’s sample()
function is a powerful tool for randomly selecting elements from a given vector or dataframe.
- The first argument,
x
, represents the vector or dataframe we want to sample. - The
size
argument specifies the number of elements we want to sample from x. - By default, the
replace
argument is set toFALSE
, meaning sampling is done without replacement. This ensures that each selected element is unique. However, setting replace = TRUE allows for sampling with replacement, allowing the same element to be selected multiple times. - The optional
prob
argument allows us to assign probabilities to each element in x. This enables us to perform weighted sampling, where elements with higher probabilities are more likely to be selected.
Utilizing the sample() function with these arguments, we can effortlessly generate random samples from vectors or dataframes in R. This flexibility and simplicity make it a go-to choice, for many, when it comes to random sampling in R in various data analysis scenarios.
The slice_sample() Function from dplyr
The slice_sample() function from the dplyr package in R allows us to sample rows from a dataframe based on specified criteria randomly.
- The
.data
argument represents the input dataframe from which we want to sample rows. - The
…
argument allows for additional conditions or expressions to be applied during the sampling process. - The
n
argument specifies the exact number of rows we want to sample from the dataframe. - Alternatively, the prop argument allows us to specify the proportion or fraction of rows to sample from the dataframe.
- The
by
argument enables us to group the dataframe by one or more variables before sampling. This is useful when we want to sample within specific groups or strata. - The
weight_by
argument allows us to assign sampling weights to each row in the dataframe. This can be utilized when performing weighted sampling, where specific rows are more likely to be selected. - By default, the
replace
argument is set toFALSE
, meaning sampling is done without replacement. However, settingreplace = TRUE
allows for sampling with replacement, allowing the same row to be selected multiple times.
By using the slice_sample()
function with some of these arguments, we can effortlessly generate random samples of rows from dataframes in R while considering various conditions and sampling strategies. This versatility, combined with the rich functionality of dplyr, empowers us to perform complex data manipulations and sampling operations with ease.
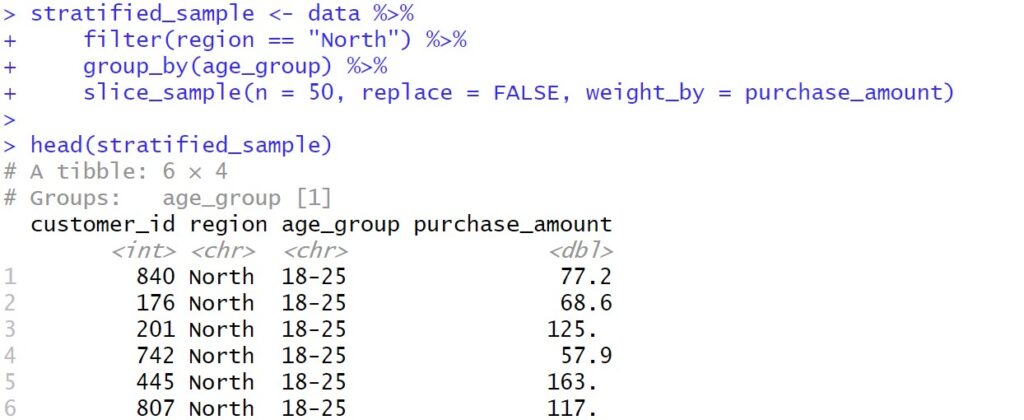
Synthetic Data
Here we generate a synthetic dataset in R that we can use to practice randomly selecting rows:
library(dplyr)
groups <- c("Group A", "Group B", "Group C")
data <- data.frame(
group = rep(groups, each = 100)
) %>%
mutate(
perception = ifelse(
group %in% c("Group A", "Group B") | group == "Group C",
rnorm(300, mean = 8, sd = 1),
NA
)
)
Code language: JavaScript (javascript)
In the code chunk above, we use the dplyr package in R for data manipulation tasks. We start by loading the dplyr library, allowing us to access its functions. Moreover, we define a vector called groups
that contains three group labels: “Group A”, “Group B”, and “Group C”. Next, we create a dataframe named data
using the data.frame() function. Within the dataframe, we create a column named group
by repeating the elements of groups
100 times each, resulting in 300 rows.
To assign values to the perception
column, we use the mutate() function from dplyr. Using the ifelse()
function, we apply conditions using %in% in R and the or operator (‘|’). If the group
is either “Group A” or “Group B” or if it is equal to “Group C”, we generate 300 random normal values with a mean of 8 and a standard deviation of 1. Otherwise, we assign the value NA to the perception
column for the remaining cases. In the next section, we will use sample()
to take a random sample from the synthetic data.
How to Randomly Select Rows in R using the sample() Function
Here is how to randomly select rows in R using the sample()
function:
# Randomly select 50 rows from the dataframe
random_sample <- data[sample(nrow(data), 50), ]
Code language: PHP (php)
In the code chunk above, we used the sample()
function to randomly select rows from the dataframe. Here is a breakdown of the code:
nrow(data)
returns the number of rows in thedata
dataframe.sample(nrow(data), 50)
generates a random sample of 50 row indices from 1 to the number of rows in data.- Finally, we use these randomly selected row indices to subset the
data
dataframe and store the result in the random_sample variable.
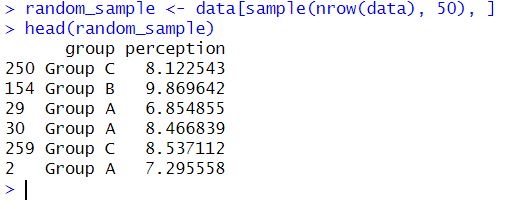
After running the code, the random_sample
dataframe will contain 50 randomly selected rows from the original data dataframe. In the next subsection, we will randomly select 1/4 of the total rows of a dataframe in R using the same function.
Randomly Select 1/4 of the Total Rows of a dataframe in R
Here is how to randomly select one-fourth (25%) of the data from the dataframe data:
# Randomly select 25% of the rows from the dataframe
random_sample <- data[sample(nrow(data), nrow(data) * 0.25), ]
Code language: PHP (php)
In the code chunk above, we multiply the total number of rows in the data
dataframe (nrow(data)
) by 0.25
to select one-fourth of the data. Here is a breakdown of the code:
nrow(data) * 0.25
calculates the desired number of rows, which is 25% (1/4) of the total number of rows indata
.sample(nrow(data), nrow(data) * 0.25)
generates a random sample of row indices using the calculated number of rows.- Finally, we use these randomly selected row indices to subset the
data
dataframe and store the result in therandom_sample
variable.
After running this updated code, the random_sample
dataframe will contain approximately one-fourth of the randomly selected rows from the original data
dataframe. The next section will cover how to take a random sample of a dataframe using slice_sample()
.
How to Randomly Select Rows in R using slice_sample()
Here is how to use slice_sample()
in R to take a random sample from dataframe:
library(dplyr)
# Randomly select 50 rows from the dataframe
random_sample <- data %>% slice_sample(n = 50)
Code language: PHP (php)
In the code chunk above code, we used the slice_sample()
function to randomly select a specific number of rows from the dataframe. Here’s an explanation of the code:
data %>%
is a pipe operator (%>%
) that passes the dataframedata
as the input for the subsequent function.slice_sample(n = 50)
performs the random sampling operation, wheren
is set to 50 to indicate the desired number of rows to be selected randomly.
After running this code, the random_sample
dataframe will contain 50 randomly selected rows from the original data
dataframe using the slice_sample()
function.
Randomly Select 25% of the Total Rows using slice_sample()
Here is how we randomly select 1/4 of the total rows in R using slice_sample()
:
library(dplyr)
# Randomly select 25% of the rows from the dataframe
random_sample <- data %>% slice_sample(prop = 0.25)
Code language: PHP (php)
In this code, we utilize the slice_sample()
function from dplyr to randomly select rows based on a proportion of the data. Here’s an explanation of the code:
data %>%
is a pipe operator (%>%
) that passes the dataframedata
as the input for the subsequent function.slice_sample(prop = 0.25)
performs the random sampling operation, whereprop
is set to 0.25 to indicate the desired proportion (25%) of the data to be selected randomly.
By executing this code, the random_sample
dataframe will contain approximately one-fourth of the randomly selected rows from the original data
dataframe using the slice_sample()
function.
Conclusion
In this post, you have learned how to select rows in R using two powerful functions randomly, sample()
and slice_sample()
.
First, we explored the sample()
function, which allowed us to randomly select a specific number or proportion of rows from a dataframe. We saw how this function can be useful for selecting a random subset of data for analysis or modeling purposes.
Next, we introduced the slice_sample()
function from the dplyr
package, which provided an alternative approach to random row selection. With this function, we could easily specify the number or proportion of rows to be sampled, making it convenient for various sampling needs.
Throughout the post, we used a synthetic dataset based on psychology example data to demonstrate the functionality of these functions. By following the examples and explanations provided, you gained the ability to use these functions for your data analysis tasks.
If you found this post informative and valuable, I encourage you to share it on social media and with your colleagues. Spread the knowledge and help others discover the techniques for randomly selecting rows in R. Additionally, I would love to hear from you! Comment on the blog if you have any specific topics or techniques you want me to cover in future posts. Your feedback is valuable to us. Please let me know if you encounter any errors or have suggestions for improvement.
Resources
Here are some other blog posts you may find useful:
- Countif function in R with Base and dplyr
- Sum Across Columns in R – dplyr & base
- How to Convert a List to a Dataframe in R – dplyr
- How to Create a Matrix in R with Examples – empty, zeros
- How to Rename Factor Levels in R using levels() and dplyr
- R: Add a Column to Dataframe Based on Other Columns with dplyr