In this tutorial, you will learn to use Pandas to convert all columns to string. As a data enthusiast or analyst, you have likely encountered datasets with diverse data types, and harmonizing them is important.
Table of Contents
- Outline
- Optimizing Data Consistency
- Why Convert All Columns?
- How to Change Data Type to String in Pandas
- The to_string() function to Convert all Columns to a String
- Synthetic Data
- Convert all Columns to String in Pandas Dataframe
- Pandas Convert All Columns to String
- Conclusion
- More Tutorials
Outline
The structure of this post is outlined as follows. First, we discuss optimizing data consistency by converting all columns to a uniform string data type in a Pandas dataframe.
Next, we explore the fundamental technique of changing data types to strings using the .astype()
function in Pandas. This method provides a versatile and efficient way to convert individual columns to strings.
To facilitate hands-on exploration, we introduce a section on Synthetic Data. This synthetic dataset, containing various data types, allows you to experiment with the conversion process, gaining practical insights.
This post’s central part demonstrates how to comprehensively convert all columns to strings in a Pandas dataframe, using the .astype()
function. This method is precious when a uniform string representation of the entire dataset is desired.
Concluding the post, we introduce an alternative method for converting the entire DataFrame to a string using the to_string()
function. This overview provides a guide, empowering you to choose the most suitable approach based on your specific data consistency needs.
Optimizing Data Consistency
Imagine dealing with datasets where columns contain various data types, especially when working with object columns. By converting all columns to strings, we ensure uniformity, simplifying subsequent analyses and paving the way for seamless data manipulation.
Why Convert All Columns?
This conversion is a strategic move, offering a standardized approach to handle mixed data types efficiently. Whether preparing data for machine learning models or ensuring consistency in downstream analyses, this tutorial empowers you with the skills to navigate and transform your dataframe effortlessly.
Let us get into the practical steps and methods that will empower you to harness the full potential of pandas in managing and converting all columns to strings.
How to Change Data Type to String in Pandas
In Pandas programming, the .astype()
method is a versatile instrument for data type manipulation. When applied to a single column, such as df['Column'].astype(str)
, it swiftly transforms the data within that column into strings. However, when converting all columns, a more systematic approach is required. To navigate this, we learn a broader strategy, exploring how to iterate through each column, applying .astype(str)
dynamically. This method ensures uniformity across diverse data types. Additionally, it sets the stage for further data preprocessing by employing complementary functions tailored to specific conversion needs. Here are some more posts using, e.g., the .astype()
to convert columns:
- Pandas Convert Column to datetime – object/string, integer, CSV & Excel
- How to Convert a Float Array to an Integer Array in Python with NumPy
The to_string() function to Convert all Columns to a String
In Pandas programming, the .to_string()
function emerges as a concise yet potent tool for transforming an entire dataframe into a string representation. Executing df.to_string()
seamlessly converts all columns, offering a comprehensive dataset view. Unlike the targeted approach of .astype()
, .to_string()
provides a more general solution, fostering consistency throughout diverse data types
Synthetic Data
Here, we generate a synthetic data set to practice converting all columns to strings in Pandas dataframe:
# Generating synthetic data
import pandas as pd
import numpy as np
np.random.seed(42)
data = pd.DataFrame({
'NumericColumn': np.random.randint(1, 100, 5),
'FloatColumn': np.random.rand(5),
'StringColumn': ['A', 'B', 'C', 'D', 'E']
})
# Displaying the synthetic data
print(data)
Code language: PHP (php)
In the code chunk above, we have created a synthetic dataset with three columns of distinct data types: ‘NumericColumn’ comprising integers, ‘FloatColumn’ with floating-point numbers, and ‘StringColumn’ containing strings (‘A’ through ‘E’). This dataset showcases how to convert all columns to strings in Pandas. Next, let us proceed to the conversion process.
Convert all Columns to String in Pandas Dataframe
One method to convert all columns to string in a Pandas DataFrame is the .astype(str) method. Here is an example:
# Converting all columns to string
data2 = data.astype(str)
# Displaying the updated dataset
print(data)
Code language: PHP (php)
In the code chunk above, we used the .astype(str)
method to convert all columns in the Pandas dataframe to the string data type. This concise and powerful method efficiently transforms each column, ensuring the entire dataset is represented as strings. To confirm this transformation, we can inspect the data types before and after the conversion:
# Check the data types before and after conversion
print(data.dtypes) # Output before: Original data types
data = data.astype(str)
print(data2.dtypes) # Output after: All columns converted to 'object' (string)
Code language: PHP (php)
The first print statement displays the original data types of the dataframe, and the second print statement confirms the successful conversion, with all columns now being of type ‘object’ (string).
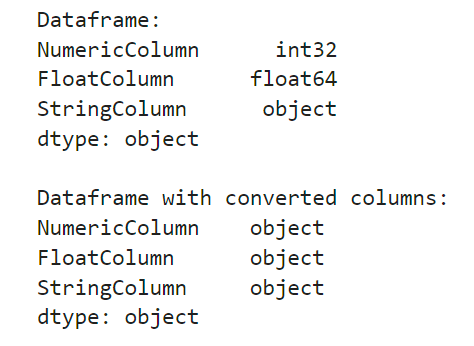
Pandas Convert All Columns to String
If we, rather than creating string objects of the columns, want the entire data frame to be represented as a string, we can use the to_string
function in Pandas. It is particularly useful when printing or displaying the entire dataframe as a string, especially if the dataframe is large and does not fit neatly in the console or output display.
Here is a basic example:
# Use to_string to get a string representation
data_string = data.to_string()
Code language: PHP (php)
In the code chunk above, we used the to_string
method on a Pandas dataframe named data^. This function is applied to render the dataframe as a string representation, allowing for better readability, especially when dealing with large datasets. After executing the code, the variable
data_string` now holds the string representation of the dataframe.
To demonstrate the transformation, we can use the type
function to reveal the data type of the original dataframe and the one after the conversion:
print(type(data))
data2 = data.to_string()
print(type(data2))
Code language: PHP (php)
Here, we confirm that data
is of type dataframe, while data_string
is now a string object. That is, we have successfully converted the Pandas object to a string.
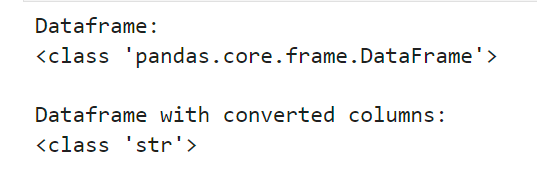
Conclusion
In this post, you learned to convert all columns to string in a Pandas dataframe using the powerful .astype()
method. We explored the significance of this conversion in optimizing data consistency ensuring uniformity across various columns. The flexibility and efficiency of the .astype()
function were demonstrated, allowing you to tailor the conversion to specific columns.
As a bonus, we introduced an alternative method using the to_string()
function, showcasing its utility for converting the entire dataframe into a string format. Understanding when to use .astype()
versus to_string()
adds a layer of versatility to your data manipulation toolkit.
Your newfound expertise empowers you to handle diverse datasets effectively, ensuring they meet the consistency standards required for robust analysis. If you found this post helpful or have any questions, suggestions, or specific topics you would like me to cover, please share your thoughts in the comments below. Consider sharing this resource with your social network, extending the knowledge to others who might find it beneficial.
More Tutorials
Here are som more Pandas and Python tutorials you may find helpful:
- How to Get the Column Names from a Pandas Dataframe – Print and List
- Combine Year and Month Columns in Pandas
- Coefficient of Variation in Python with Pandas & NumPy
- Python Scientific Notation & How to Suppress it in Pandas & NumPy