Knowing the variable type in R is essential for any data analysis or programming task. This post will explore two methods to check the variable type in R and understand when and why it is important.
When working with data in R, knowing the type of variables we are dealing with is crucial. R is a dynamically typed language, which means that variables can change their type during the execution of a program. This flexibility can be both a blessing and a curse. On one hand, it allows for more efficient memory usage and code flexibility. On the other hand, it can lead to unexpected results if we are unaware of the variable types.
Knowing the variable type ensures our code works correctly and avoids potential errors or bugs. It also helps in understanding the structure of our data and choosing the appropriate functions or operations to perform on it. Knowing the variable type lets you change it to the most suitable for your needs. Here are some tutorials on changing data types in R:
- Convert Multiple Columns to Numeric in R with dplyr
- Convert All Character Columns to Factor in R: A Guide
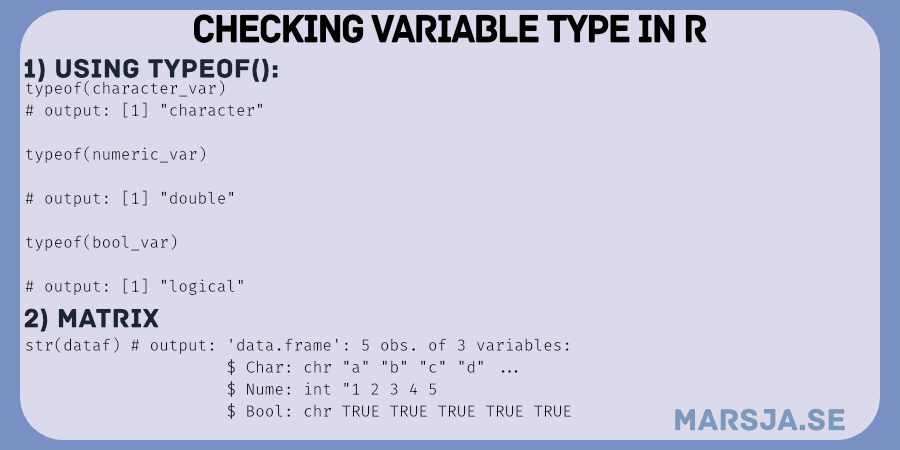
In the upcoming sections, we will explore two commonly used methods to check the variable type in R: the str()
function and the typeof()
function. We will discuss the differences between these methods and provide examples to illustrate their usage. By the end of this article, you will clearly understand how to check the variable type in R and which method is best suited for your needs.
Table of Contents
- Outline
- Prerequisites
- Check Variable Type in R: the different methods.
- Checking Variable type in R with the typeof() function
- How to Check Variable Type in R with the str() Function
- Practical Example: Checking and Changing Variable Type in R
- Best Method to Check Variable Type in R
- FAQ
- Conclusion
- Resources
Outline
The structure of the current post is as follows: First, we will look at what you need to know to follow this post about checking variable types in R, outlining the prerequisites for a comprehensive understanding. Following this, we will get into the heart of the topic by exploring two primary functions for checking variable types: str()
and typeof()
. We will explain the syntax and applications of the two methods. Moving on, we will focus on the practical aspect of checking and changing variable types in R, providing real-world scenarios for clarity.
In the subsequent section, we will address which method is the best for checking variable types in R, guiding you to make an informed choice. To ensure all your queries are addressed, we have dedicated an FAQ section to clarify common doubts and misconceptions surrounding variable type checking. Finally, we will conclude the post with a comprehensive summary, reinforcing the significance of mastering this fundamental skill in data analysis. Whether a novice or an experienced data analyst, this post will equip you with essential knowledge and techniques for efficient data handling in the R programming language.
Prerequisites
Before exploring variable types in R, there are a few essential prerequisites. First and foremost, having a basic understanding of R syntax and programming concepts is beneficial. Familiarity with R’s syntax will facilitate a smoother grasp of the variable type-checking methods discussed in this post.
Additionally, it is advisable to use an up-to-date version of R (learn how to check R version). Newer versions often come with enhanced features and improved compatibility, which can be advantageous when working with data analysis and manipulation. If your R version is not up to date, consider updating R to make the most of the functionalities discussed in this post. With these prerequisites in place, you will be well-prepared to check and manage variable types in R with confidence and efficiency.
Check Variable Type in R: the different methods.
There are several methods available in R to check the variable type. Two commonly used methods are the str()
function and the typeof()
function. These functions provide different ways to determine the type of a variable.
The str()
function is short for “structure” and is used to display the structure of an R object. It provides a compact and informative summary of the object, including its type, length, and content. This function is handy when working with complex data structures, such as data frames or lists, as it gives a comprehensive overview of the object’s components.
On the other hand, the typeof()
function returns the variable type as a character string. It provides a more basic information level than the str()
function. The typeof()
function is useful when we only need to know the fundamental type of a variable, such as whether it is a numeric, character, or logical value.
Both the str()
and typeof()
functions have advantages and can be used depending on the specific requirements of we analysis or programming task. The following sections will explore each method in detail, discussing their syntax, usage, and examples. By the end of this article, we will clearly understand how to check the variable type in R using these different methods.
str()
The str()
function in R is a powerful tool for checking an object’s variable type and structure. It concisely summarizes the object’s type, length, and content. Understanding the syntax of the str()
function is essential for effectively using it in our R code.
To use the str()
function, we must pass the object we want to examine as an argument. For example, if we have a variable named my_variable
and we want to check its type, we would use the following syntax:
str(my_variable)
Code language: R (r)
The str()
function will then display a detailed summary of the object, including its type and any nested components. This can be particularly useful when working with complex data structures like dataframes or lists.
By examining the output of the str()
function, we can quickly identify the type of our variable and gain insights into its structure. This information is crucial for performing data analysis or debugging we code.
typeof()
The typeof()
function in R is another method for checking the variable type. It provides a simple way to determine the type of an object without displaying its structure or content. Understanding the syntax of the typeof()
function is crucial for effectively using it in our R code.
To use the typeof()
function, we must pass the object we want to examine as an argument. For example, if we have a variable named “my_variable” and we want to check its type, we would use the following syntax:
typeof(my_variable)
Code language: R (r)
The typeof()
function will then return a character string representing the type of the object. The possible return values include “integer”, “double”, “character”, “logical”, “complex”, “raw”, “list”, “expression”, “function”, and “NULL”.
The typeof()
function is particularly useful when we only need to know the basic type of an object and don’t require a detailed summary of its structure. It can be handy for quick type checks or conditional statements in our R code.
In the following sections, we will explore examples of how to use the str()
and typeof()
functions to check the variable type in R. We will cover different scenarios and demonstrate how the typeof()
function can be valuable in your R programming toolkit.
Checking Variable type in R with the typeof() function
The typeof()
function in R is another method for checking the variable type. It returns a character string indicating the type of the object.
To use the typeof()
function, we must pass the object we want to check as an argument. As previously mentioned, if we have a variable named my_variable
” and we want to determine its type, we would use the following syntax:
typeof(my_variable)
Code language: R (r)
Here are some examples to illustrate the usage of the typeof()
function:
x <- 5
typeof(x)
Code language: R (r)
Here is for a character variable:
y <- "Hello"
typeof(y)
Code language: JavaScript (javascript)
And for a boolean variable:
z <- TRUE
typeof(z)
Code language: JavaScript (javascript)
By using the typeof()
function, you can quickly determine the basic type of your variables. However, it may not provide as much detailed information as the str()
function. In the next section, we will look at a practical example that showcase how we can use dplyr
together with typeof()
.
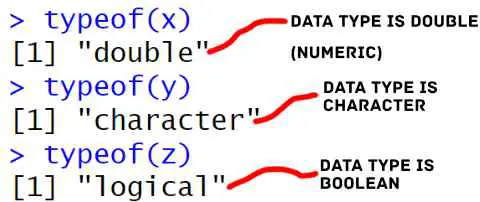
How to Check Variable Type in R with the str() Function
The str()
function in R is another useful method for checking the variable type. It provides a detailed summary of the structure and content of an object, making it easier to understand its type.
To use the str()
function, we must pass the object we want to examine as an argument. For instance, if we have a variable named “my_variable” and we want to check its type, we would use the following syntax:
str(my_variable)
Code language: R (r)
In the code chunk above, we used str()
on a character variable. Naturally, we can also use str()
on a matrix:
str(my_matrix)
Code language: R (r)
Finally, we can also use str()
on a dataframe str(my_datafram
e)
. Here is the output:
In the following section, we will briefly discuss which of these two methods is the best for checking the data type in R.
Practical Example: Checking and Changing Variable Type in R
Below is a practical example showcasing how we can check the variable type in R and perform data type conversions using the powerful dplyr package. In data analysis and manipulation, it is common to encounter datasets with mixed or incorrect data types. This could hinder our analysis, making it crucial to verify and adjust data types as required.
# Load the dplyr library
library(dplyr)
# Sample dataframe
data <- data.frame(
ID = c(1, 2, 3, 4, 5),
Name = c("Alice", "Bob", "Charlie", "David", "Eve"),
Age = c("25", "30", "22", "35", "28"),
Score = c(92.5, 87.3, 78.9, 94.6, 89.2),
Passed = c("TRUE", "TRUE", "FALSE", "TRUE", "TRUE")
)
# Check and convert data types
data <- data %>%
mutate_if(is.character, as.numeric) %>%
mutate_if(is.logical, as.factor)
Code language: R (r)
In the code chunk above, we utilize the dplyr library in R to perform a practical example of checking and manipulating variable types. The sample dataframe contains columns containing mixed data types, characters, and logical values. Using the mutate_if() function, we check for columns of character and logical data types. Subsequently, we convert these columns to the appropriate data types—numeric and factor, respectively. This example demonstrates the value of verifying and adjusting variable types in data analysis, ensuring data consistency and facilitating accurate analyses.
Best Method to Check Variable Type in R
When it comes to checking variable types in R, we have discussed two functions that can be used: str()
and typeof()
. Both functions provide information about the type of an object, but they have some differences.
The str()
function is known for its ability to provide detailed information about an object. It tells us the object type and provides additional details such as the object’s length, dimension, and content. This can be particularly useful when dealing with complex data structures or when we need a comprehensive understanding of the object.
On the other hand, the typeof()
function is more straightforward and provides a basic type of object. It returns a character string indicating the type of the object, such as “integer”, “numeric”, “character”, or “logical”. This function is useful when we only need to know the basic type of the variable and don’t require detailed information.
So, which method is the best? It depends on our specific needs. The str()
function is better if we require detailed information about the object. However, if we only need to know the basic type of the variable, the typeof()
function is sufficient. In the next section, we will address frequently asked questions about checking variable types in R to clarify your doubts further.
FAQ
This section will address frequently asked questions about checking variable types in R.
To check the data type of a variable in R, you can use the str() or typeof() function. The str() function provides detailed information about the object, including its type, length, dimension, and content. On the other hand, the typeof() function gives you the basic type of the object, such as “integer”, “numeric”, “character”, or “logical”.
The type of a variable in R depends on its content. R supports various data types, including numeric, integer, character, and logic. You can use the typeof() function to determine the basic type of a variable.
You can use the is.numeric() function to check if a column is numeric in R. This function returns a logical value indicating whether each element in the column is numeric. You can apply this function to a specific column or the entire dataset.
I hope to have clarified how to check variable types in R by addressing these frequently asked questions.
Conclusion
In conclusion, this article has provided various methods to check variable types in R. By using the str()
function, you can obtain detailed information about the object, including its type, length, dimension, and content. On the other hand, the typeof()
function gives you the basic type of the object, such as “integer”, “numeric”, “character”, or “logical”.
Throughout this article, we have addressed frequently asked questions about checking variable types in R, providing clarity on the topic. We have also discussed the best method for checking variable types in R. Now that you have a solid understanding of checking variable types in R, I encourage you to share this valuable information with others. By sharing this article on social media, you can help fellow R users enhance their data analysis skills and improve their programming efficiency.
Remember, accurately determining the variable type is crucial for performing appropriate operations and analyses in R. Whether you are working with numeric, character, or logical data, the methods discussed in this article will assist you in effectively checking variable types in R. Thank you for reading, and I hope this article has been informative and helpful to you.
Resources
Here are some additional blog posts that you may find helpful:
- How to Check if a File is Empty in R: Practical Examples
- How to use %in% in R: 8 Example Uses of the Operator
- Modulo in R: Practical Example using the %% Operator
- How to Rename Column (or Columns) in R with dplyr
- How to Remove a Column in R using dplyr (by name and index)