This post provides a step-by-step guide on how to rename files in Python. The post explains how to rename a single file in Python in four simple steps. First, you need to get the file path of the file that you want to rename. Second, copy the path of the file to rename. Third, import the os module, a built-in Python module that uses operating system-dependent functionality. Finally, use the os module to rename the file.
The post also provides instructions on how to rename multiple files in Python. It explains that replacing characters in the file name is one way to rename multiple files. This can be done by looping through the files in a directory and using the string method replace() to replace the characters in the file name.
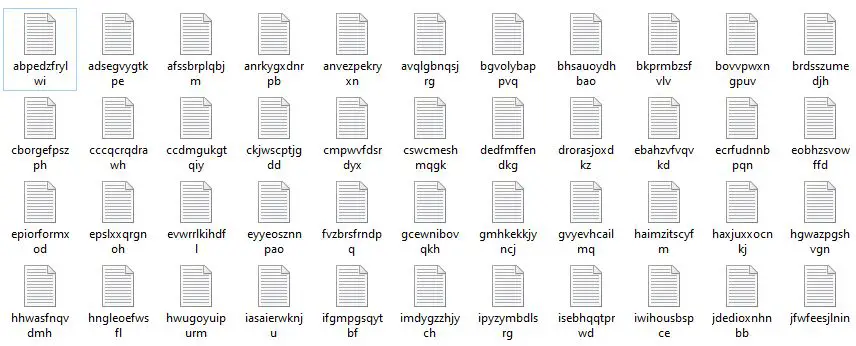
Table of Contents
- How to Rename a File in Python
- 4 Simple Steps to Rename a File in Python
- How to Rename Multiple Files in Python
- Conclusion: Renaming Files in Python
- Python Tutorials
How to Rename a File in Python
For instance, if we have a file called python-rename-files.txt we need to know where we have stored it. There are, of course, different ways to do this. We can place the Python script in the same directory or just run it to rename the file. Here is a simple example of how to rename a file in Python:
import os
os.rename('python-rename-files.txt', 'renamed-file.txt')
Code language: Python (python)
4 Simple Steps to Rename a File in Python
In the first section, we will learn how to rename a single file in Python step-by-step. Now, the general procedure is similar when using Linux or Windows. However, how we go about the first step to rename a file in Python may differ depending on which OS we use. In the renaming a file in Python examples below, we will learn how to carry on and change names in Linux and Windows.
1. Getting the File Path of the File we Want to Rename With Python
First, to get Python to rename a file, Python needs to know where the file is located. Step 1 is finding the file’s location we want to change the name on.
If we store our Python scripts (or Jupyter notebooks) in certain directories, we need to tell Python the complete path to the file we want to rename.
Finding the File Path in Windows
If we use Windows, we can open up File Explorer. First, go to the folder where the file is located (e.g., “Files_To_Rename”) and click on the file path (see image below). It should look something like “C:\Users\erima96\Documents\Files_To_Rename”.
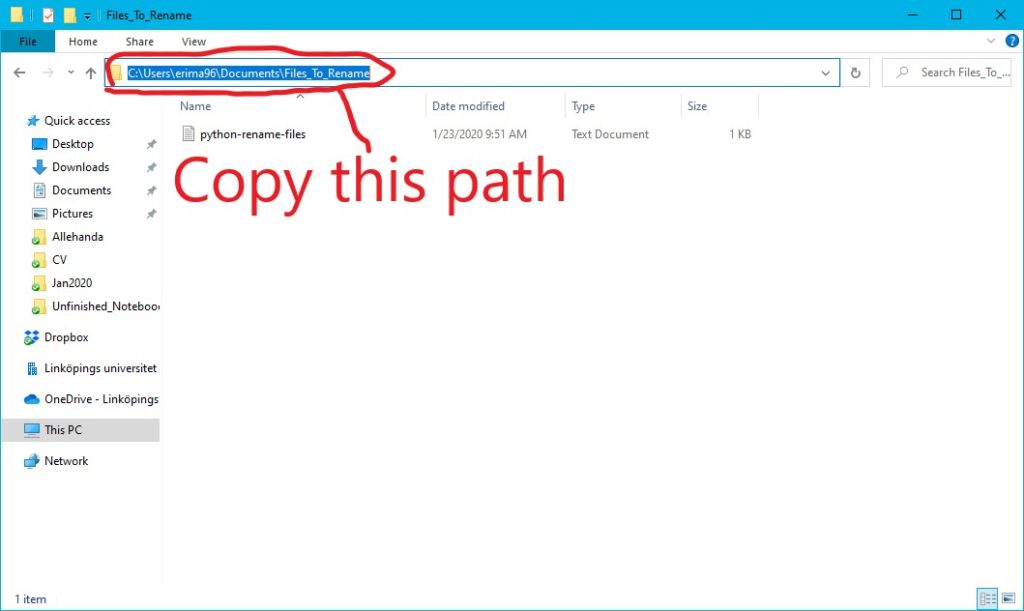
Finding the File Path in Linux
Now if we are to rename a file using Python in Linux, we need to know the path. There are, of course, many methods to find the file path in Linux. One way is to open up a Terminal Window and use the readlink and xclip command-line applications:
readlink -f FILE_AND PATH | xclip -i
Code language: Bash (bash)
For instance, if the file we want to rename with Python is located in the folder “/home/jhf/Documents/Files_To_Rename/python-rename-files.txt” this is how we would do it:
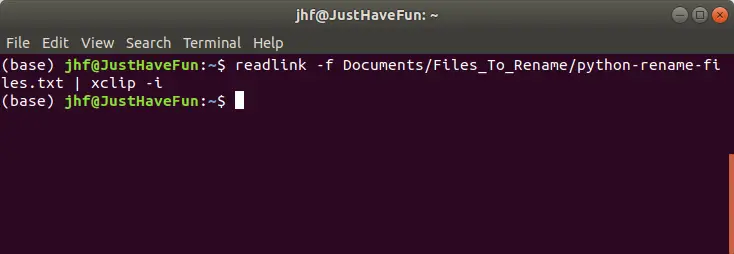
2. Copy the Path of the File to Rename
Second, we copy the path of the file we want to rename. Note, this is as simple as just right-clicking on the path we already have marked:
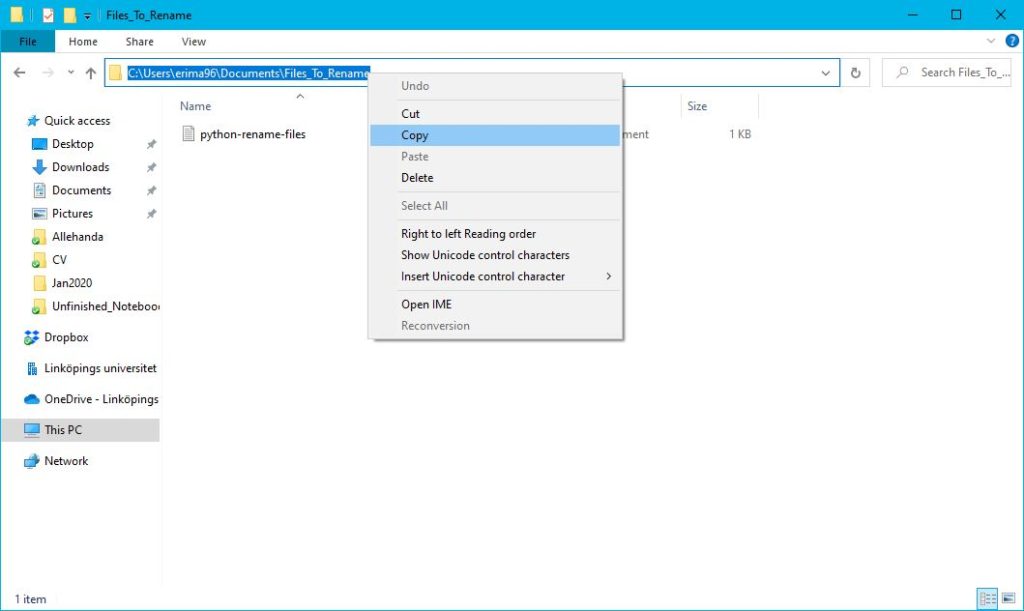
3. Importing the os Module
Third, we need to import a Python module called os. Luckily, this is very easy, and we type: import os
. In the next step, we will rename a file using Python!
4. Renaming the File
Finally, we are ready to change the file name using the os module from Python. As the file path can be long we will create a string variable first and then rename the file using os.rename
. Here’s how to rename a file using Python:os.rename(PATH_TO_THE_FILE, PATH_TO_THE_NEW_FILE)
Changing a Name of a File in Windows
Here is a complete code example, for Windows users, on how to change the name of a file using Python:
import os
file_path = 'C:\\Users\\erima96\\Documents\\Files_To_Rename\\'
os.rename(file_path + 'renamed-file.txt',
file_path + 'python-rename-files.txt')
Code language: Python (python)
Note how we added an extra ”\” to each subfolder (and at the end). In the os.rename
method, we added the file_path to both the file we want to rename and the new file name (the string variable with the path to the file) and added the file name using ‘+’. If we don’t do this, the file will also be moved to the Python script folder. If we run the above code, we can see that we have successfully renamed the file using Python:
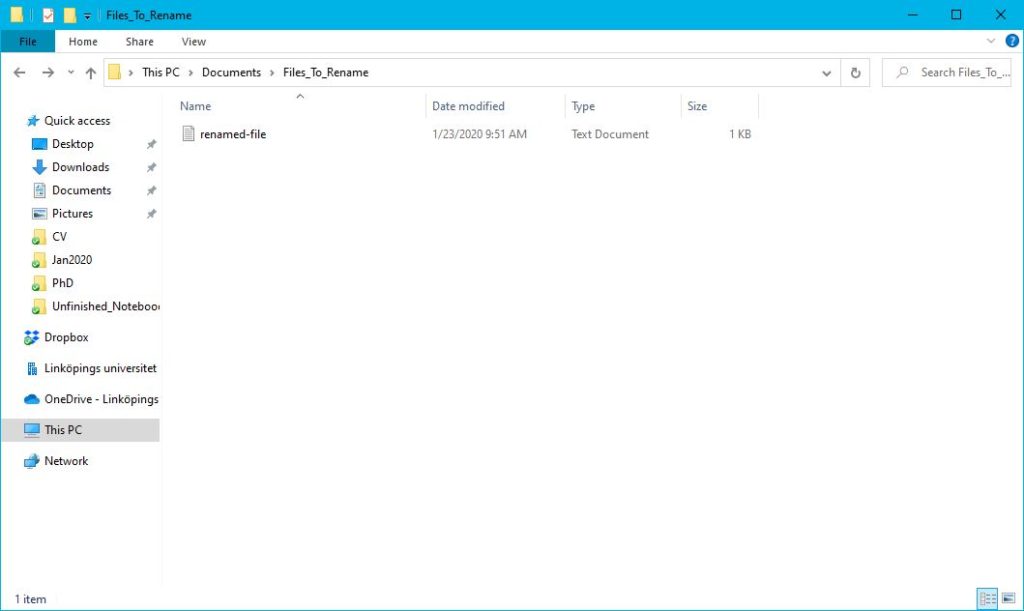
Other use cases of the os module:
Renaming a File in Linux
Here’s how to rename the file in Linux. Note the file path will be a bit different (as we saw earlier when we found the file path):
import os
file_path = '/home/jhf/Documents/Files_To_Rename/python-rename-files.txt'
os.rename(file_path + 'renamed-file.txt',
file_path + 'python-rename-files.txt')
Code language: Python (python)
Now that we have learned how to rename a file in Python, we will continue with an example in which we change the name of multiple files. Renaming numerous files can be useful if many need new names.
How to Rename Multiple Files in Python
In this section, we will learn how to rename multiple files in Python. There are, of course, several ways to do this. One method could be creating a Python list with all the file names we want to change. However, if we have many files, this is not an optimal way to change the name of multiple files. Thus, we will use another method from the os module; listdir. Furthermore, we will also use the filter method from the module called fnmatch.
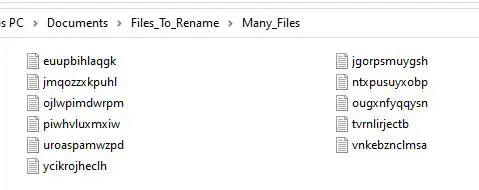
Before renaming the files, the first step is to create a list of all text files in a folder (e.g., in the subfolder “Many_Files”). Note that we have added the subfolder to the file_path string.
import os, fnmatch
file_path = 'C:\\Users\\erima96\\Documents\\Files_To_Rename\\Many_Files\\'
files_to_rename = fnmatch.filter(os.listdir(file_path), '*.txt')
print(files_to_rename)
Code language: Python (python)

Next, we will loop through each file name and rename them. In the example below, we use the file_path string from the code chunk above because this is where the files are located. If we were to have the files we want to rename in a different folder, we’d change file_path to that folder. Furthermore, we are creating a new file name (e.g., new_name = ‘new_file’), and we use the enumerate method to give them a unique name. For example, the file ‘euupbihlaqgk.txt’ will be renamed ‘Datafile1.txt’.
new_name = 'Datafile'
for i, file_name in enumerate(files_to_rename):
new_file_name = new_name + str(i) + '.txt'
os.rename(file_path + file_name,
file_path + new_file_name)
Code language: Python (python)
As can be seen in the rename a file in Python example above, we also used the str() method to change the data type of the integer variable called i to a string.
Remember, if we were using Linux, we’d have to change the file_path so that it would find the files we want to rename.
Now, there are other useful functions in Python. For instance, if we need to get the absolute value in Python, we can use the abs() function.
Renaming Multiple Files by Replacing Characters in the File Name
Now, sometimes we have files that we want to replace certain characters. In this final example, we will use the replace method to replace underscores (“_”) from file names. This, of course, means that we need to rename the files.
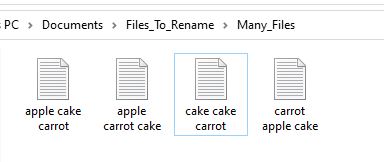
import os, fnmatch
file_path = 'C:\\Users\\erima96\\Documents\\Files_To_Rename\\Many_Files\\'
files_to_rename = fnmatch.filter(os.listdir(file_path), '*.txt')
for file_name in files_to_rename:
os.rename(file_path + file_name,
file_path + file_name.replace(' ', '-'))
Code language: Python (python)
In the code chunk above, we have successfully replaced the “_” from the filenames using replace and then renamed the files. Again, remember if we rename files in Linux, the file_path string needs to be changed (e.g., ” “).
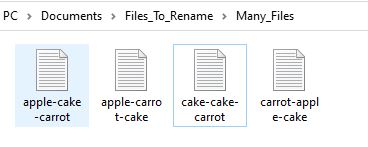
Conclusion: Renaming Files in Python
In this post, you have learned how to rename Python files for single and multiple files. By following the step-by-step instructions in this post, you can easily change the names of files in a directory and save time and effort. Python’s built-in os module provides a convenient and efficient way of renaming files, and this post has explained how to use this module for this purpose.
Renaming files in Python can be useful for various programming projects, and this post has provided a beginner-friendly guide for anyone looking to rename files in Python. With the instructions and code examples in this post, you can confidently apply these techniques to your projects and modify file names to suit your needs.
Remember, commenting and sharing on social media is a great way to engage with the community and show support for the content that you find valuable. So, if you found this post helpful, why not share it with others who might benefit from it? And do not forget to leave a comment below to let me know how this post has helped you in your Python programming journey!
Python Tutorials
Here are some more tutorials you will find helpful:
- Coefficient of Variation in Python with Pandas & NumPy
- Find the Highest Value in Dictionary in Python
- Pandas Count Occurrences in Column – i.e. Unique Values
- How to Get the Column Names from a Pandas Dataframe – Print and List
- How to Perform a Two-Sample T-test with Python: 3 Different Methods
thanks for the content
Hey Lava, thanks for your comment. I always aim to be as comprehensive as possible in my posts. Hope you learned what you needed about renaming files in Python.
Best,
Dr. Marsja