In this Pandas tutorial, we will learn six methods to get the column names from Pandas dataframe. One of the nice things about Pandas dataframes is that each column will have a name (i.e., the variables in the dataset). Now, we can use these names to access specific columns by name without knowing which column number it is.
To access the names of a Pandas dataframe, we can use the method columns(). For example, if our dataframe is called df
we just type print(df.columns)
to get all the columns of the Pandas dataframe.
Table of Contents
- Outline
- Importing Data from a CSV File
- Pandas How to Get the Column Names from the Dataframe:
- How to Get Values by Column Name:
- How to Rename a Column
- Conclusion: Getting all the Column Names with Pandas
- Resources
Outline
The outline of this post is to provide you with a comprehensive guide on retrieving column names from a Pandas dataframe. We will explore various methods and techniques to accomplish this task efficiently.
First, we will learn the first technique, which uses Pandas’s columns()
method. This method lets us directly obtain the column names from the dataframe, providing a quick and straightforward solution.
Next, we will explore another method using the keys()
method, which returns the column names as an Index object. This method provides flexibility in accessing and manipulating the column names.
Moving forward, we will discuss how to iterate over the columns of a dataframe to extract their names. This approach allows customized operations or additional processing based on the specific column names.
Additionally, we will explore using the list()
function to print the column names as a list and the tolist()
method to obtain the names as a list object. These methods provide different formatting options depending on your specific needs.
Lastly, we will demonstrate using the sorted()
function to obtain an ordered list of column names, which can be useful when dealing with large datasets or requiring specific column order.
In the latter part of the post, we will focus on retrieving values based on column names, showcasing how to access specific columns and their corresponding values.
By the end of this post, you will have a solid understanding of various techniques to retrieve column names in Pandas, empowering you to work efficiently with and manipulate your dataframe. So, let us start learning how to get column names in Pandas.
Importing Data from a CSV File
First, before learning the six methods to obtain the column names in Pandas, we need some example data. In this post, we will use Pandas read_csv to import data from a CSV file (from this URL). Now, the first step is, as usual, when working with Pandas to import Pandas as pd.
import pandas as pd
df = pd.read_csv('https://vincentarelbundock.github.io/Rdatasets/csv/carData/UN98.csv',
index_col=0)
df.head()
Code language: Python (python)
It is, of course, also possible to read xlsx files using Pandas read_excel method. Another method to get our data into Python is to convert a dictionary to a Pandas dataframe. After you have found the answer to the question, “How do I get column names in Pandas?” you will learn how to get column names in six different ways.
To get the column names in Pandas dataframe you can type print(df.columns)given that your dataframe is named “df”. There are, of course, at least 5 other options for getting the column names of your dataframe (e.g., sorted(df)).
Pandas How to Get the Column Names from the Dataframe:
We are ready to learn how to get all the names using six different methods. First, we use the DataFrame.columns method to print all names:
1. Get the Column Names Using the columns() Method
Now, one of the simplest methods to get all the columns from a Pandas dataframe is, of course, using the columns method and printing it. In the code chunk below, we are doing exactly this.
print(df.columns)
Code language: Python (python)
Right, the columns method will get the labels of the dataframe. That is, when we use print
, we will print column names (i.e., the labels). Here’s the result of the above code:

In the next example, we are going to use the keys() method to print all the names in the dataframe:
2. Using the keys() Method
Second, we can get the exact same result by using the keys()
method. We will also get the column names by the following code.
# Dataframe show all columns
print(df.keys())
Code language: Python (python)
In the following example, we will iterate over the DataFrame.columns to print each name on a separate line.
3. By Iterating the Columns
In the third method, we will iterate over the columns to get the column names. As you may notice, we are again using the columns method.
# Get all names
for col_name in df.columns:
print(col_name)
Code language: Python (python)
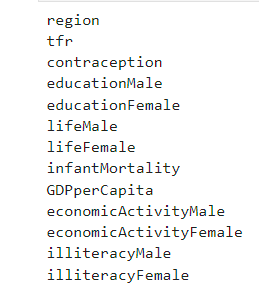
In the next example, we will get all the names using the list() method together with the df.columns
method.
4. Using list() to Print the Names as a list
In the fourth method, on the other hand, we are going to use the list() method to print the column names as a list.
# Print the columns as list
print(list(df.columns))
Code language: Python (python)

Another option, which we will see in the next example, is the tolist() method.
5. Using tolist() to Print the Names as a List
Now, we can use the values method, as well, to get the columns from Pandas dataframe. If we also use the tolist()
method, we will get a list.
# Show all columns as list
print(df.columns.values.tolist())
Code language: Python (python)
6. Using sorted() to Get an Ordered List
Now, in the final, and sixth, method to print the names, we will use sorted()
to get the columns from a Pandas dataframe in alphabetic order:
# Dataframe show all columns sorted list
sorted(df)
Code language: Python (python)
As previously mentioned, when using sorted we will get this ordered list of column names:
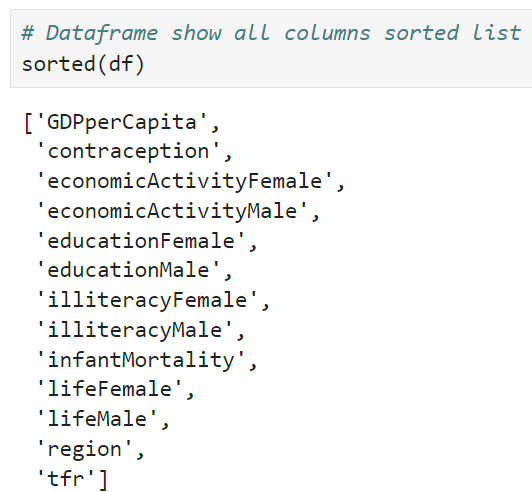
How to Get Values by Column Name:
Now, that we know the column names of our dataframe we can access one column (or many). Here’s how we get the values from one column:
print(df['tfr'].values)
Code language: Python (python)
If we, on the other hand, want to access more than one column we add a list: df[['tfr', 'region']]
How to Rename a Column
In the final example, what we can do when we know the column names of a Pandas dataframe is to rename a column.
df.rename(columns={'tfr': 'TFR'})
Code language: Python (python)
Note, if we want to save the changed name to our dataframe we can add the inplace=True, to the code above. In the video below, you will learn how to use the inplace parameter and all the other things from this post. In a recent post, you will learn all you need about renaming columns in Pandas dataframe.
Conclusion: Getting all the Column Names with Pandas
In this post, you have learned various techniques to retrieve column names from a Pandas dataframe. We explored the columns()
and keys()
methods, which provided direct access to the column names. By iterating over the columns, using list()
or tolist()
, and employing sorted()
for an ordered list, you gained versatility in obtaining and manipulating column names.
We also covered retrieving values by column name, enabling you to access specific columns and their corresponding values effectively. Throughout the post, you comprehensively understood these methods, empowering you to confidently navigate and utilize column names in your data analysis tasks.
By mastering these techniques, you can streamline your workflow, perform targeted operations, and take advantage of the power of Pandas for efficient data manipulation. Whether you are extracting insights, transforming data, or conducting further analysis, the knowledge gained here will prove invaluable.
Remember to explore the diverse possibilities offered by Pandas, including its integration with other Python libraries, to enhance your data exploration and manipulation capabilities further. With your newfound expertise in retrieving column names, you are well-equipped to excel in your data analysis endeavors.
I hope this post has provided you with valuable insights and practical guidance. Now, confidently retrieve Pandas column names to unlock your data-driven projects’ full potential. Please share this post on your social media accounts if you like it. Others may also find it useful!
Finally, here is the Jupyter Notebook with all the code.
Resources
Here are some other useful Pandas tutorials:
- Python Scientific Notation & How to Suppress it in Pandas & NumPy
- How to Convert a NumPy Array to Pandas Dataframe: 3 Examples
- Pandas Count Occurrences in Column – i.e. Unique Values
- How to Make Column Index in Pandas Dataframe – with Examples
- Pandas Convert Column to datetime – object/string, integer, CSV & Excel
- Adding New Columns to a Dataframe in Pandas (with Examples)
- How to Convert JSON to Excel in Python with Pandas
you can also print/get one specific column name using:
> df.columns[#]
where # is the column number
Hey Anibel! Thanks for this comment. Always nice when readers add to the posts with other methods. Like in this case, how to print a specific column.
Best,
Erik
Very insightful.
Thank you, Hicham, for the kind comment. I am glad you liked learning how to get the column names from Pandas dataframe.
Thanks a lot. Just have found sth I was looking for it a lot!
Hey there! Thanks for your comment. I am glad it helped you get the column names from Pandas dataframe.
print(df.dtypes) is a good approach as well. Yes, we will see data types as well.
Hey Denis,
Thanks for your comment and suggestion,
Best,
Erik