In this blog post, we will learn how to replace NA (missing) values in a data.table
in R and we have previously looked on how to replace NA with the previous value in a data.table. Handling missing data is a common task in data analysis, and data.table offers a powerful way to manipulate large datasets, including replacing missing values with specific values. We will walk through two examples: first, replacing NAs with 0, and then with another common value.
Table of Contents
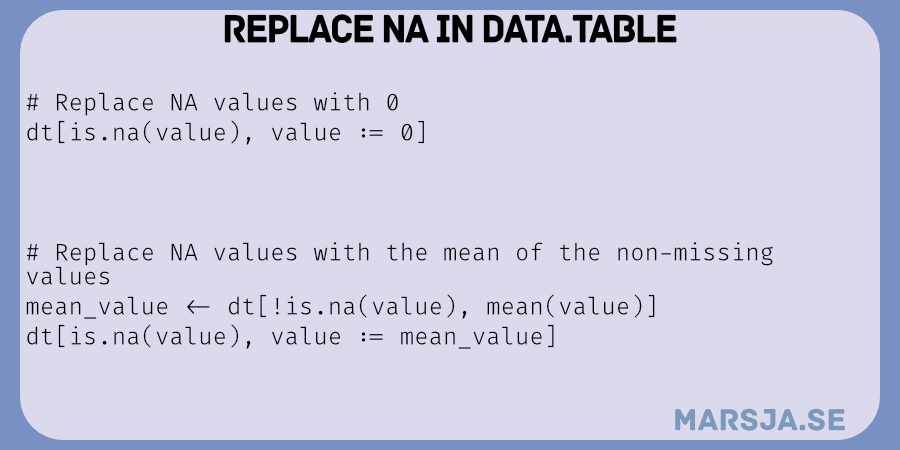
Replacing NA with 0
The first example involves replacing missing values (NA) with zero. This is a common approach, especially when dealing with numerical data, where zero can signify an absence of value or a baseline. Here is how you can do this with data.table:
# Replace NA values with 0
dt[is.na(value), value := 0]
# View the result
print(dt)
Code language: R (r)
In the code above, we first create a simple data.table
with some NA
values. The is.na(value)
condition checks for missing values, and the :=
operator allows us to replace them directly in the data.table
by assigning 0
to those positions. This method is efficient and works well even with larger datasets.
Replacing NA with Another Value (e.g., Mean)
Next, we will replace missing values with a more meaningful value, such as the mean of the non-missing values. This is a common approach in imputation strategies, where we want to preserve the data’s distribution by replacing missing values with the mean (or median, mode, etc.).
# Replace NA values with the mean of the non-missing values
mean_value <- dt[!is.na(value), mean(value)]
dt[is.na(value), value := mean_value]
# View the result
print(dt)
Code language: R (r)
Here, we calculate the mean of the value
column by excluding the NAs (!is.na(value)
). Then, we replace the missing values with the computed mean using the same is.na(value)
condition and :=
operator.
Summary
In this post, we demonstrated two methods for replacing NA values in a data.table. First, we replaced missing values with zero, and then we replaced them with the mean of the non-missing values. These techniques help clean and prepare data, especially when you need to handle large datasets efficiently. By using data.table you can perform these operations at high speed and with minimal memory usage.
Sharing is caring! If you found these methods helpful, share this post with others who may benefit from them. If there is something specific related to R and data.table that you would like me to cover in a future post, drop a comment below! I would love to hear your thoughts and make more great content that fits your needs!
Resources
Here are some more data.table tutorials: